Utility methods for mathematical problems.
/*
* MathUtil.java
*
* Copyright (C) 2005-2008 Tommi Laukkanen
* http://www.substanceofcode.com
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
*/
//package com.substanceofcode.util;
/**
* Utility methods for mathematical problems.
*
* @author Tommi Laukkanen
*/
public class MathUtil {
/** Square root from 3 */
final static public double SQRT3 = 1.732050807568877294;
/** Log10 constant */
final static public double LOG10 = 2.302585092994045684;
/** ln(0.5) constant */
final static public double LOGdiv2 = -0.6931471805599453094;
public final static double EVal = 2.718281828459045235;
/** Creates a new instance of MathUtil */
private MathUtil() {
}
/**
* Returns the value of the first argument raised to the power of the second
* argument.
*
* @author Mario Sansone
*/
public static int pow(int base, int exponent) {
boolean reciprocal = false;
if (exponent < 0) {
reciprocal = true;
}
int result = 1;
while (exponent-- > 0) {
result *= base;
}
return reciprocal ? 1 / result : result;
}
public static double pow(double base, int exponent) {
boolean reciprocal = false;
if (exponent < 0) {
reciprocal = true;
}
double result = 1;
while (exponent-- > 0) {
result *= base;
}
return reciprocal ? 1 / result : result;
}
/** Arcus cos */
static public double acos(double x) {
double f = asin(x);
if (f == Double.NaN) {
return f;
}
return Math.PI / 2 - f;
}
/** Arcus sin */
static public double asin(double x) {
if (x < -1. || x > 1.) {
return Double.NaN;
}
if (x == -1.) {
return -Math.PI / 2;
}
if (x == 1) {
return Math.PI / 2;
}
return atan(x / Math.sqrt(1 - x * x));
}
/** Arcus tan */
static public double atan(double x) {
boolean signChange = false;
boolean Invert = false;
int sp = 0;
double x2, a;
// check up the sign change
if (x < 0.) {
x = -x;
signChange = true;
}
// check up the invertation
if (x > 1.) {
x = 1 / x;
Invert = true;
}
// process shrinking the domain until x<PI/12
while (x > Math.PI / 12) {
sp++;
a = x + SQRT3;
a = 1 / a;
x = x * SQRT3;
x = x - 1;
x = x * a;
}
// calculation core
x2 = x * x;
a = x2 + 1.4087812;
a = 0.55913709 / a;
a = a + 0.60310579;
a = a - (x2 * 0.05160454);
a = a * x;
// process until sp=0
while (sp > 0) {
a = a + Math.PI / 6;
sp--;
}
// invertation took place
if (Invert) {
a = Math.PI / 2 - a;
}
// sign change took place
if (signChange) {
a = -a;
}
//
return a;
}
public static double log(double x) {
if (x < 0) {
return Double.NaN;
}
//
if (x == 1) {
return 0d;
}
if (x == 0) {
return Double.NEGATIVE_INFINITY;
}
//
if (x > 1) {
x = 1 / x;
return -1 * _log(x);
}
return _log(x);
}
public static double _log(double x) {
double f = 0.0;
// Make x to close at 1
int appendix = 0;
while (x > 0 && x < 1) {
x = x * 2;
appendix++;
}
//
x = x / 2;
appendix--;
//
double y1 = x - 1;
double y2 = x + 1;
double y = y1 / y2;
//
double k = y;
y2 = k * y;
//
for (long i = 1; i < 50; i += 2) {
f = f + (k / i);
k = k * y2;
}
//
f = f * 2;
for (int i = 0; i < appendix; i++) {
f = f + (LOGdiv2);
}
//
return f;
}
/**
* Replacement for missing Math.pow(double, double)
*
* @param x
* @param y
* @return
*/
public static double pow(double x, double y) {
// Convert the real power to a fractional form
int den = 1024; // declare the denominator to be 1024
/*
* Conveniently 2^10=1024, so taking the square root 10 times will yield
* our estimate for n. In our example n^3=8^2 n^1024 = 8^683.
*/
int num = (int) (y * den); // declare numerator
int iterations = 10; /*
* declare the number of square root iterations
* associated with our denominator, 1024.
*/
double n = Double.MAX_VALUE; /*
* we initialize our estimate, setting it to
* max
*/
while (n >= Double.MAX_VALUE && iterations > 1) {
/*
* We try to set our estimate equal to the right hand side of the
* equation (e.g., 8^2048). If this number is too large, we will
* have to rescale.
*/
n = x;
for (int i = 1; i < num; i++) {
n *= x;
}
/*
* here, we handle the condition where our starting point is too
* large
*/
if (n >= Double.MAX_VALUE) {
iterations--; /* reduce the iterations by one */
den = (int) (den / 2); /* redefine the denominator */
num = (int) (y * den); // redefine the numerator
}
}
/*************************************************
** We now have an appropriately sized right-hand-side. Starting with
* this estimate for n, we proceed.
**************************************************/
for (int i = 0; i < iterations; i++) {
n = Math.sqrt(n);
}
// Return our estimate
return n;
}
public final static int abs(int in) {
if (in < 0.0) {
return in * -1;
}
return in;
}
public final static long abs(long in) {
if (in < 0.0) {
return in * -1;
}
return in;
}
public final static double abs(double in) {
if (in < 0.0) {
return in * -1.0;
}
return in;
}
}
Related examples in the same category
1. | Absolute value | | |
2. | Find absolute value of float, int, double and long using Math.abs | | |
3. | Find ceiling value of a number using Math.ceil | | |
4. | Find exponential value of a number using Math.exp | | |
5. | Find floor value of a number using Math.floor | | |
6. | Find minimum of two numbers using Math.min | | |
7. | Find power using Math.pow | | |
8. | Find square root of a number using Math.sqrt | | |
9. | Find natural logarithm value of a number using Math.log | | |
10. | Find maximum of two numbers using Math.max | | |
11. | Get the power value | | 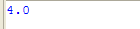 |
12. | Using the Math Trig Methods | | 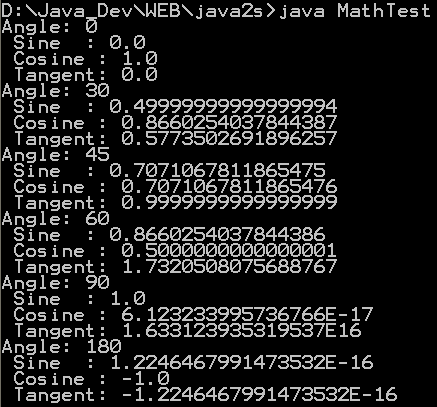 |
13. | Using BigDecimal for Precision | | 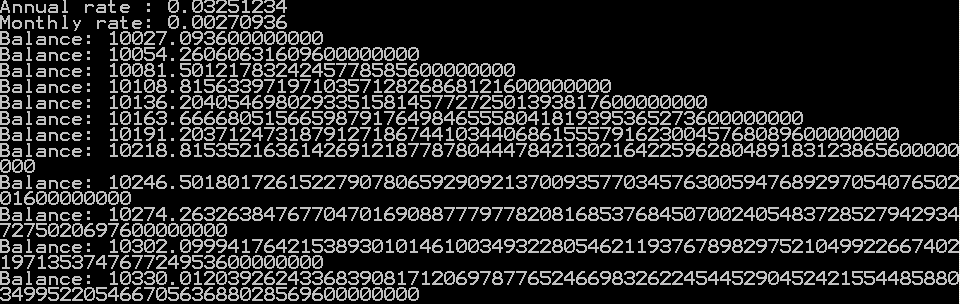 |
14. | Demonstrate our own version round() | | 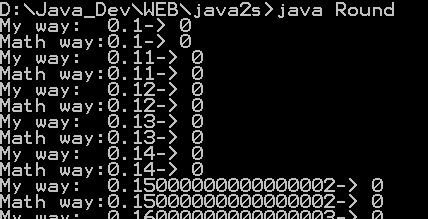 |
15. | Demonstrate a few of the Math functions for Trigonometry | |  |
16. | Exponential Demo | | 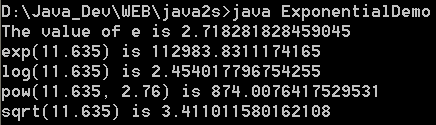 |
17. | Min Demo | | |
18. | Basic Math Demo | | 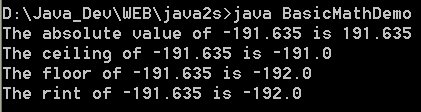 |
19. | Using strict math in applications | |  |
20. | Conversion between polar and rectangular coordinates | | |
21. | Using the pow() function | | |
22. | Using strict math at the method level | | |
23. | Calculating hyperbolic functions | | |
24. | Calculating trigonometric functions | | |
25. | Weighted floating-point comparisons | | |
26. | Solving right triangles | | |
27. | Applying the quadratic formula | | |
28. | Calculate the floor of the log, base 2 | | |
29. | Greatest Common Divisor (GCD) of positive integer numbers | | |
30. | Least Common Multiple (LCM) of two strictly positive integer numbers | | |
31. | Moving Average | | |
32. | Make Exponention | | |
33. | Caclulate the factorial of N | | |
34. | Trigonometric Demo | | 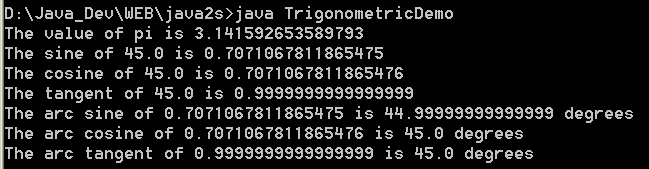 |
35. | Complex Number Demo | | |
36. | sqrt(a^2 + b^2) without under/overflow | | |
37. | Returns an integer hash code representing the given double array value. | | |
38. | Returns an integer hash code representing the given double value. | | |
39. | Returns n!. Shorthand for n Factorial, the product of the numbers 1,...,n as a double. | | |
40. | Returns n!. Shorthand for n Factorial, the product of the numbers 1,...,n. | | |
41. | Returns the hyperbolic sine of x. | | |
42. | Contains static definition for matrix math methods. | | |
43. | For a double precision value x, this method returns +1.0 if x >= 0 and -1.0 if x < 0. Returns NaN if x is NaN. | | |
44. | For a float value x, this method returns +1.0F if x >= 0 and -1.0F if x < 0. Returns NaN if x is NaN. | | |
45. | Normalize an angle in a 2&pi wide interval around a center value. | | |
46. | Normalizes an angle to a relative angle. | | |
47. | Normalizes an angle to an absolute angle | | |
48. | Normalizes an angle to be near an absolute angle | | |
49. | Returns the natural logarithm of n!. | | |
50. | Returns the least common multiple between two integer values. | | |
51. | Gets the greatest common divisor of the absolute value of two numbers | | |
52. | Matrix manipulation | | |
53. | Returns exact (http://mathworld.wolfram.com/BinomialCoefficient.html) Binomial Coefficient | | |
54. | Returns a double representation of the (http://mathworld.wolfram.com/BinomialCoefficient.html) Binomial Coefficient | | |
55. | Returns the natural log of the (http://mathworld.wolfram.com/BinomialCoefficient.html) Binomial Coefficient | | |
56. | Returns the hyperbolic cosine of x. | | |
57. | Math Utils | | |
58. | Implements the methods which are in the standard J2SE's Math class, but are not in in J2ME's. | | |
59. | A math utility class with static methods. | | |
60. | Computes the binomial coefficient "n over k" | | |
61. | Log Gamma | | |
62. | Log Beta | | |
63. | Beta | | |
64. | Gamma | | |
65. | Factorial | | |
66. | Computes p(x;n,p) where x~B(n,p) | | |
67. | Returns the sum of two doubles expressed in log space | | |
68. | sigmod | | |
69. | sigmod rev | | |
70. | Numbers that are closer than this are considered equal | | |
71. | Returns the KL divergence, K(p1 || p2). | | |
72. | Returns the sum of two doubles expressed in log space | | |
73. | Returns the difference of two doubles expressed in log space | | |
74. | Is Prime | | |
75. | Statistical functions on arrays of numbers, namely, the mean, variance, standard deviation, covariance, min and max | | |
76. | This class calculates the Factorial of a numbers passed into the program through command line arguments. | |  |
77. | Calculates the Greatest Common Divisor of two numbers passed into the program through command line arguments. | | |
78. | Variance: the square of the standard deviation. | | |
79. | Population Standard Deviation | | |
80. | Returns from a static prime table the least prime that is greater than or equal to a specified value. | | |