/*
* Copyright (c) 1995 - 2008 Sun Microsystems, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* - Neither the name of Sun Microsystems nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS
* IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* ListCutPaste.java requires the following files:
* ListTransferHandler.java
* TransferActionListener.java
*/
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.GridLayout;
import java.awt.KeyboardFocusManager;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.StringSelection;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.IOException;
import javax.swing.Action;
import javax.swing.ActionMap;
import javax.swing.BorderFactory;
import javax.swing.DefaultListModel;
import javax.swing.DropMode;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JList;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.KeyStroke;
import javax.swing.ListSelectionModel;
import javax.swing.TransferHandler;
import javax.swing.UIManager;
/**
* The ListCutPaste example illustrates cut, copy, paste and drag and drop using
* three instances of JList. The TransferActionListener class listens for one of
* the CCP actions and, when one occurs, forwards the action to the component
* which currently has the focus.
*/
public class ListCutPaste extends JPanel {
ListTransferHandler lh;
public ListCutPaste() {
super(new BorderLayout());
lh = new ListTransferHandler();
JPanel panel = new JPanel(new GridLayout(1, 3));
DefaultListModel list1Model = new DefaultListModel();
list1Model.addElement("alpha");
list1Model.addElement("beta");
list1Model.addElement("gamma");
list1Model.addElement("delta");
list1Model.addElement("epsilon");
list1Model.addElement("zeta");
JList list1 = new JList(list1Model);
list1.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
JScrollPane sp1 = new JScrollPane(list1);
sp1.setPreferredSize(new Dimension(400, 100));
list1.setDragEnabled(true);
list1.setTransferHandler(lh);
list1.setDropMode(DropMode.ON_OR_INSERT);
setMappings(list1);
JPanel pan1 = new JPanel(new BorderLayout());
pan1.add(sp1, BorderLayout.CENTER);
pan1.setBorder(BorderFactory.createTitledBorder("Greek Alphabet"));
panel.add(pan1);
DefaultListModel list2Model = new DefaultListModel();
list2Model.addElement("uma");
list2Model.addElement("dois");
list2Model.addElement("tres");
list2Model.addElement("quatro");
list2Model.addElement("cinco");
list2Model.addElement("seis");
JList list2 = new JList(list2Model);
list2.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
list2.setDragEnabled(true);
JScrollPane sp2 = new JScrollPane(list2);
sp2.setPreferredSize(new Dimension(400, 100));
list2.setTransferHandler(lh);
list2.setDropMode(DropMode.INSERT);
setMappings(list2);
JPanel pan2 = new JPanel(new BorderLayout());
pan2.add(sp2, BorderLayout.CENTER);
pan2.setBorder(BorderFactory.createTitledBorder("Portuguese Numbers"));
panel.add(pan2);
DefaultListModel list3Model = new DefaultListModel();
list3Model.addElement("adeen");
list3Model.addElement("dva");
list3Model.addElement("tri");
list3Model.addElement("chyetirye");
list3Model.addElement("pyat");
list3Model.addElement("shest");
JList list3 = new JList(list3Model);
list3.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
list3.setDragEnabled(true);
JScrollPane sp3 = new JScrollPane(list3);
sp3.setPreferredSize(new Dimension(400, 100));
list3.setTransferHandler(lh);
list3.setDropMode(DropMode.ON);
setMappings(list3);
JPanel pan3 = new JPanel(new BorderLayout());
pan3.add(sp3, BorderLayout.CENTER);
pan3.setBorder(BorderFactory.createTitledBorder("Russian Numbers"));
panel.add(pan3);
setPreferredSize(new Dimension(500, 200));
add(panel, BorderLayout.CENTER);
}
/**
* Create an Edit menu to support cut/copy/paste.
*/
public JMenuBar createMenuBar() {
JMenuItem menuItem = null;
JMenuBar menuBar = new JMenuBar();
JMenu mainMenu = new JMenu("Edit");
mainMenu.setMnemonic(KeyEvent.VK_E);
TransferActionListener actionListener = new TransferActionListener();
menuItem = new JMenuItem("Cut");
menuItem.setActionCommand((String) TransferHandler.getCutAction().getValue(
Action.NAME));
menuItem.addActionListener(actionListener);
menuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_X,
ActionEvent.CTRL_MASK));
menuItem.setMnemonic(KeyEvent.VK_T);
mainMenu.add(menuItem);
menuItem = new JMenuItem("Copy");
menuItem.setActionCommand((String) TransferHandler.getCopyAction()
.getValue(Action.NAME));
menuItem.addActionListener(actionListener);
menuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_C,
ActionEvent.CTRL_MASK));
menuItem.setMnemonic(KeyEvent.VK_C);
mainMenu.add(menuItem);
menuItem = new JMenuItem("Paste");
menuItem.setActionCommand((String) TransferHandler.getPasteAction()
.getValue(Action.NAME));
menuItem.addActionListener(actionListener);
menuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_V,
ActionEvent.CTRL_MASK));
menuItem.setMnemonic(KeyEvent.VK_P);
mainMenu.add(menuItem);
menuBar.add(mainMenu);
return menuBar;
}
/**
* Add the cut/copy/paste actions to the action map.
*/
private void setMappings(JList list) {
ActionMap map = list.getActionMap();
map.put(TransferHandler.getCutAction().getValue(Action.NAME),
TransferHandler.getCutAction());
map.put(TransferHandler.getCopyAction().getValue(Action.NAME),
TransferHandler.getCopyAction());
map.put(TransferHandler.getPasteAction().getValue(Action.NAME),
TransferHandler.getPasteAction());
}
/**
* Create the GUI and show it. For thread safety, this method should be
* invoked from the event-dispatching thread.
*/
private static void createAndShowGUI() {
// Create and set up the window.
JFrame frame = new JFrame("ListCutPaste");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create and set up the menu bar and content pane.
ListCutPaste demo = new ListCutPaste();
frame.setJMenuBar(demo.createMenuBar());
demo.setOpaque(true); // content panes must be opaque
frame.setContentPane(demo);
// Display the window.
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
// Schedule a job for the event-dispatching thread:
// creating and showing this application's GUI.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
// Turn off metal's use of bold fonts
UIManager.put("swing.boldMetal", Boolean.FALSE);
createAndShowGUI();
}
});
}
}/*
* Copyright (c) 1995 - 2008 Sun Microsystems, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* - Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* - Neither the name of Sun Microsystems nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
/*
* ListTransferHandler.java is used by the ListCutPaste example.
*/
class ListTransferHandler extends TransferHandler {
/**
* Perform the actual data import.
*/
public boolean importData(TransferHandler.TransferSupport info) {
String data = null;
// If we can't handle the import, bail now.
if (!canImport(info)) {
return false;
}
JList list = (JList) info.getComponent();
DefaultListModel model = (DefaultListModel) list.getModel();
// Fetch the data -- bail if this fails
try {
data = (String) info.getTransferable().getTransferData(
DataFlavor.stringFlavor);
} catch (UnsupportedFlavorException ufe) {
System.out.println("importData: unsupported data flavor");
return false;
} catch (IOException ioe) {
System.out.println("importData: I/O exception");
return false;
}
if (info.isDrop()) { // This is a drop
JList.DropLocation dl = (JList.DropLocation) info.getDropLocation();
int index = dl.getIndex();
if (dl.isInsert()) {
model.add(index, data);
return true;
} else {
model.set(index, data);
return true;
}
} else { // This is a paste
int index = list.getSelectedIndex();
// if there is a valid selection,
// insert data after the selection
if (index >= 0) {
model.add(list.getSelectedIndex() + 1, data);
// else append to the end of the list
} else {
model.addElement(data);
}
return true;
}
}
/**
* Bundle up the data for export.
*/
protected Transferable createTransferable(JComponent c) {
JList list = (JList) c;
int index = list.getSelectedIndex();
String value = (String) list.getSelectedValue();
return new StringSelection(value);
}
/**
* The list handles both copy and move actions.
*/
public int getSourceActions(JComponent c) {
return COPY_OR_MOVE;
}
/**
* When the export is complete, remove the old list entry if the action was a
* move.
*/
protected void exportDone(JComponent c, Transferable data, int action) {
if (action != MOVE) {
return;
}
JList list = (JList) c;
DefaultListModel model = (DefaultListModel) list.getModel();
int index = list.getSelectedIndex();
model.remove(index);
}
/**
* We only support importing strings.
*/
public boolean canImport(TransferHandler.TransferSupport support) {
// we only import Strings
if (!support.isDataFlavorSupported(DataFlavor.stringFlavor)) {
return false;
}
return true;
}
}
/*
* Copyright (c) 1995 - 2008 Sun Microsystems, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* - Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* - Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* - Neither the name of Sun Microsystems nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
/*
* TransferActionListener.java is used by the ListCutPaste example.
*/
/**
* A class that tracks the focused component. This is necessary to delegate the
* menu cut/copy/paste commands to the right component. An instance of this
* class is listening and when the user fires one of these commands, it calls
* the appropriate action on the currently focused component.
*/
class TransferActionListener implements ActionListener, PropertyChangeListener {
private JComponent focusOwner = null;
public TransferActionListener() {
KeyboardFocusManager manager = KeyboardFocusManager
.getCurrentKeyboardFocusManager();
manager.addPropertyChangeListener("permanentFocusOwner", this);
}
public void propertyChange(PropertyChangeEvent e) {
Object o = e.getNewValue();
if (o instanceof JComponent) {
focusOwner = (JComponent) o;
} else {
focusOwner = null;
}
}
public void actionPerformed(ActionEvent e) {
if (focusOwner == null)
return;
String action = (String) e.getActionCommand();
Action a = focusOwner.getActionMap().get(action);
if (a != null) {
a.actionPerformed(new ActionEvent(focusOwner,
ActionEvent.ACTION_PERFORMED, null));
}
}
}
14.112.Drag Drop |
| 14.112.1. | Basic drag and drop |
| 14.112.2. | Dragging Text from a JLabel | 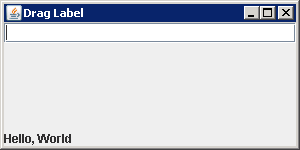 |
| 14.112.3. | Drag-and-Drop Support for Images | 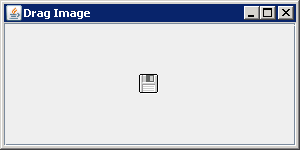 |
| 14.112.4. | Drag and drop icons: use an icon property. |
| 14.112.5. | implements DragGestureListener, Transferable |
| 14.112.6. | Dragging and dropping text between a text area, a list, and a table | 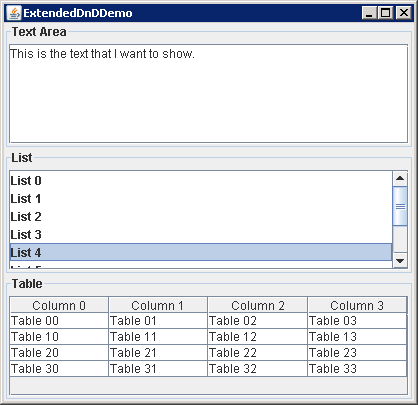 |
| 14.112.7. | Drag and drop between JTextArea and JTextField |
| 14.112.8. | Transfer both Text and Color between JTextField and JTextArea |
| 14.112.9. | Drag and drop between JList and JTextField |
| 14.112.10. | DropMode.ON |
| 14.112.11. | DropMode.INSERT |
| 14.112.12. | DropMode.ON_OR_INSERT |
| 14.112.13. | Set tree DropMode to DropMode.USE_SELECTION |
| 14.112.14. | Set tree drag mode to DropMode.ON |
| 14.112.15. | Set tree drag mode to DropMode.INSERT |
| 14.112.16. | Set tree drag mode to DropMode.ON_OR_INSERT |
| 14.112.17. | Choose Drop Action |
| 14.112.18. | Various drop actions |
| 14.112.19. | JTable drag and drop |
| 14.112.20. | Create a drag source a drop target and a transferable object. |
| 14.112.21. | Making a Component Draggable |
| 14.112.22. | Detect a drag initiating gesture in your application |
| 14.112.23. | Illustrates cut, copy, paste and drag and drop using three instances of JList |
| 14.112.24. | Location sensitive drag and drop |
| 14.112.25. | Demonstration of the top-level TransferHandler support on JFrame |
| 14.112.26. | Drag-and-Drop customization: drag the foreground color from the first label and drop it as the background color into the second one |
| 14.112.27. | Demonstrates how to add copy and drag support to a Swing component with TransferHandler |
| 14.112.28. | ScribblePane allows individual PolyLine lines to be selected, cut, copied, pasted, dragged, and dropped |
| 14.112.29. | Built-in drag and drop support: utilize a TransferHandler class |
| 14.112.30. | DND Drag and drop List |