import java.awt.Color;
import java.awt.Cursor;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.awt.dnd.DnDConstants;
import java.awt.dnd.DragGestureEvent;
import java.awt.dnd.DragGestureListener;
import java.awt.dnd.DragSource;
import java.awt.dnd.DropTarget;
import java.awt.dnd.DropTargetAdapter;
import java.awt.dnd.DropTargetDropEvent;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class ComplexExample extends JFrame implements DragGestureListener {
public ComplexExample() {
JPanel left = new JPanel();
left.setBackground(Color.red);
JPanel right = new JPanel();
right.setBackground(Color.white);
new MyDropTargetListener(right);
DragSource ds = new DragSource();
ds.createDefaultDragGestureRecognizer(left, DnDConstants.ACTION_COPY, this);
setLayout(new FlowLayout());
add(left);
add(right);
setSize(40,50);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
public void dragGestureRecognized(DragGestureEvent event) {
Cursor cursor = null;
JPanel panel = (JPanel) event.getComponent();
Color color = panel.getBackground();
if (event.getDragAction() == DnDConstants.ACTION_COPY) {
cursor = DragSource.DefaultCopyDrop;
}
event.startDrag(cursor, new TransferableColor(color));
}
class MyDropTargetListener extends DropTargetAdapter {
private DropTarget dropTarget;
private JPanel panel;
public MyDropTargetListener(JPanel panel) {
this.panel = panel;
dropTarget = new DropTarget(panel, DnDConstants.ACTION_COPY, this, true, null);
}
public void drop(DropTargetDropEvent event) {
try {
Transferable tr = event.getTransferable();
Color color = (Color) tr.getTransferData(TransferableColor.colorFlavor);
if (event.isDataFlavorSupported(TransferableColor.colorFlavor)) {
event.acceptDrop(DnDConstants.ACTION_COPY);
this.panel.setBackground(color);
event.dropComplete(true);
return;
}
event.rejectDrop();
} catch (Exception e) {
e.printStackTrace();
event.rejectDrop();
}
}
}
public static void main(String[] args) {
new ComplexExample();
}
}
class TransferableColor implements Transferable {
protected static DataFlavor colorFlavor = new DataFlavor(Color.class, "A Color Object");
protected static DataFlavor[] supportedFlavors = { colorFlavor };
Color color;
public TransferableColor(Color color) {
this.color = color;
}
public DataFlavor[] getTransferDataFlavors() {
return supportedFlavors;
}
public boolean isDataFlavorSupported(DataFlavor flavor) {
if (flavor.equals(colorFlavor) || flavor.equals(DataFlavor.stringFlavor))
return true;
return false;
}
public Object getTransferData(DataFlavor flavor) throws UnsupportedFlavorException {
if (flavor.equals(colorFlavor))
return color;
else if (flavor.equals(DataFlavor.stringFlavor))
return color.toString();
else
throw new UnsupportedFlavorException(flavor);
}
}
14.112.Drag Drop |
| 14.112.1. | Basic drag and drop |
| 14.112.2. | Dragging Text from a JLabel | 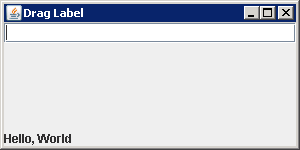 |
| 14.112.3. | Drag-and-Drop Support for Images | 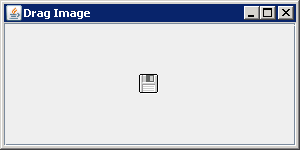 |
| 14.112.4. | Drag and drop icons: use an icon property. |
| 14.112.5. | implements DragGestureListener, Transferable |
| 14.112.6. | Dragging and dropping text between a text area, a list, and a table | 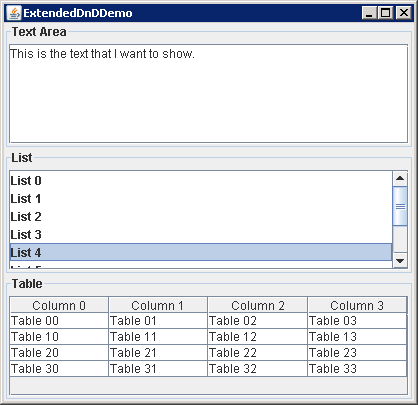 |
| 14.112.7. | Drag and drop between JTextArea and JTextField |
| 14.112.8. | Transfer both Text and Color between JTextField and JTextArea |
| 14.112.9. | Drag and drop between JList and JTextField |
| 14.112.10. | DropMode.ON |
| 14.112.11. | DropMode.INSERT |
| 14.112.12. | DropMode.ON_OR_INSERT |
| 14.112.13. | Set tree DropMode to DropMode.USE_SELECTION |
| 14.112.14. | Set tree drag mode to DropMode.ON |
| 14.112.15. | Set tree drag mode to DropMode.INSERT |
| 14.112.16. | Set tree drag mode to DropMode.ON_OR_INSERT |
| 14.112.17. | Choose Drop Action |
| 14.112.18. | Various drop actions |
| 14.112.19. | JTable drag and drop |
| 14.112.20. | Create a drag source a drop target and a transferable object. |
| 14.112.21. | Making a Component Draggable |
| 14.112.22. | Detect a drag initiating gesture in your application |
| 14.112.23. | Illustrates cut, copy, paste and drag and drop using three instances of JList |
| 14.112.24. | Location sensitive drag and drop |
| 14.112.25. | Demonstration of the top-level TransferHandler support on JFrame |
| 14.112.26. | Drag-and-Drop customization: drag the foreground color from the first label and drop it as the background color into the second one |
| 14.112.27. | Demonstrates how to add copy and drag support to a Swing component with TransferHandler |
| 14.112.28. | ScribblePane allows individual PolyLine lines to be selected, cut, copied, pasted, dragged, and dropped |
| 14.112.29. | Built-in drag and drop support: utilize a TransferHandler class |
| 14.112.30. | DND Drag and drop List |