import java.awt.BorderLayout;
import java.awt.Rectangle;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import javax.swing.DefaultListModel;
import javax.swing.DropMode;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextField;
import javax.swing.TransferHandler;
public class DnDDemo3 {
public static void main(String[] args) {
JPanel north = new JPanel();
north.add(new JLabel("Drag from here:"));
JTextField field = new JTextField(10);
field.setDragEnabled(true);
north.add(field);
final DefaultListModel listModel = new DefaultListModel();
listModel.addElement("first");
listModel.addElement("second");
final JList list = new JList(listModel);
list.setDragEnabled(true);
list.setTransferHandler(new TransferHandler() {
public boolean canImport(TransferHandler.TransferSupport support) {
if (!support.isDataFlavorSupported(DataFlavor.stringFlavor)) {
return false;
}
JList.DropLocation dl = (JList.DropLocation) support.getDropLocation();
if (dl.getIndex() == -1) {
return false;
} else {
return true;
}
}
public boolean importData(TransferHandler.TransferSupport support) {
if (!canImport(support)) {
return false;
}
Transferable transferable = support.getTransferable();
String data;
try {
data = (String) transferable.getTransferData(DataFlavor.stringFlavor);
} catch (Exception e) {
return false;
}
JList.DropLocation dl = (JList.DropLocation) support.getDropLocation();
int index = dl.getIndex();
if (dl.isInsert()) {
listModel.add(index, data);
} else {
listModel.set(index, data);
}
Rectangle r = list.getCellBounds(index, index);
list.scrollRectToVisible(r);
return true;
}
});
JScrollPane center = new JScrollPane();
center.setViewportView(list);
list.setDropMode(DropMode.USE_SELECTION);
JPanel cp = new JPanel();
cp.setLayout(new BorderLayout());
cp.add(north, BorderLayout.NORTH);
cp.add(center, BorderLayout.CENTER);
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setContentPane(cp);
frame.pack();
frame.setVisible(true);
}
}
14.112.Drag Drop |
| 14.112.1. | Basic drag and drop |
| 14.112.2. | Dragging Text from a JLabel | 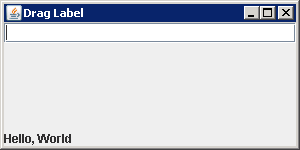 |
| 14.112.3. | Drag-and-Drop Support for Images | 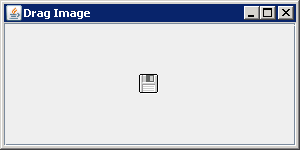 |
| 14.112.4. | Drag and drop icons: use an icon property. |
| 14.112.5. | implements DragGestureListener, Transferable |
| 14.112.6. | Dragging and dropping text between a text area, a list, and a table | 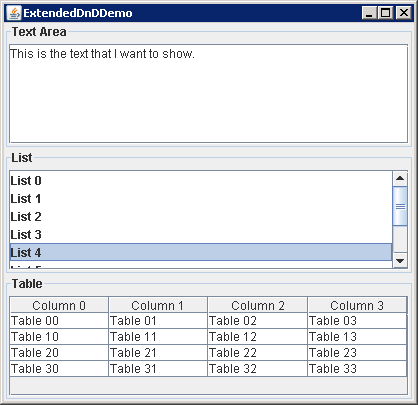 |
| 14.112.7. | Drag and drop between JTextArea and JTextField |
| 14.112.8. | Transfer both Text and Color between JTextField and JTextArea |
| 14.112.9. | Drag and drop between JList and JTextField |
| 14.112.10. | DropMode.ON |
| 14.112.11. | DropMode.INSERT |
| 14.112.12. | DropMode.ON_OR_INSERT |
| 14.112.13. | Set tree DropMode to DropMode.USE_SELECTION |
| 14.112.14. | Set tree drag mode to DropMode.ON |
| 14.112.15. | Set tree drag mode to DropMode.INSERT |
| 14.112.16. | Set tree drag mode to DropMode.ON_OR_INSERT |
| 14.112.17. | Choose Drop Action |
| 14.112.18. | Various drop actions |
| 14.112.19. | JTable drag and drop |
| 14.112.20. | Create a drag source a drop target and a transferable object. |
| 14.112.21. | Making a Component Draggable |
| 14.112.22. | Detect a drag initiating gesture in your application |
| 14.112.23. | Illustrates cut, copy, paste and drag and drop using three instances of JList |
| 14.112.24. | Location sensitive drag and drop |
| 14.112.25. | Demonstration of the top-level TransferHandler support on JFrame |
| 14.112.26. | Drag-and-Drop customization: drag the foreground color from the first label and drop it as the background color into the second one |
| 14.112.27. | Demonstrates how to add copy and drag support to a Swing component with TransferHandler |
| 14.112.28. | ScribblePane allows individual PolyLine lines to be selected, cut, copied, pasted, dragged, and dropped |
| 14.112.29. | Built-in drag and drop support: utilize a TransferHandler class |
| 14.112.30. | DND Drag and drop List |