/**
* This is an example of a component, which serves as a DragSource as
* well as Drop Target.
* To illustrate the concept, JList has been used as a droppable target
* and a draggable source.
* Any component can be used instead of a JList.
* The code also contains debugging messages which can be used for
* diagnostics and understanding the flow of events.
*
* @version 1.0
*/
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.StringSelection;
import java.awt.datatransfer.Transferable;
import java.awt.dnd.DnDConstants;
import java.awt.dnd.DragGestureEvent;
import java.awt.dnd.DragGestureListener;
import java.awt.dnd.DragSource;
import java.awt.dnd.DragSourceDragEvent;
import java.awt.dnd.DragSourceDropEvent;
import java.awt.dnd.DragSourceEvent;
import java.awt.dnd.DragSourceListener;
import java.awt.dnd.DropTarget;
import java.awt.dnd.DropTargetDragEvent;
import java.awt.dnd.DropTargetDropEvent;
import java.awt.dnd.DropTargetEvent;
import java.awt.dnd.DropTargetListener;
import javax.swing.DefaultListModel;
import javax.swing.JList;
import javax.swing.ListModel;
public class DNDList extends JList implements DropTargetListener, DragSourceListener, DragGestureListener
{
/**
* enables this component to be a dropTarget
*/
DropTarget dropTarget = null;
/**
* enables this component to be a Drag Source
*/
DragSource dragSource = null;
/**
* constructor - initializes the DropTarget and DragSource.
*/
public DNDList( ListModel dataModel )
{
super( dataModel );
dropTarget = new DropTarget( this, this );
dragSource = new DragSource();
dragSource.createDefaultDragGestureRecognizer( this, DnDConstants.ACTION_MOVE, this );
}
/**
* is invoked when you are dragging over the DropSite
*
*/
public void dragEnter( DropTargetDragEvent event )
{
// debug messages for diagnostics
System.out.println( "dragEnter" );
event.acceptDrag( DnDConstants.ACTION_MOVE );
}
/**
* is invoked when you are exit the DropSite without dropping
*
*/
public void dragExit( DropTargetEvent event )
{
System.out.println( "dragExit" );
}
/**
* is invoked when a drag operation is going on
*
*/
public void dragOver( DropTargetDragEvent event )
{
System.out.println( "dragOver" );
}
/**
* a drop has occurred
*
*/
public void drop( DropTargetDropEvent event )
{
try
{
Transferable transferable = event.getTransferable();
// we accept only Strings
if( transferable.isDataFlavorSupported( DataFlavor.stringFlavor ) )
{
event.acceptDrop( DnDConstants.ACTION_MOVE );
String s = ( String )transferable.getTransferData( DataFlavor.stringFlavor );
addElement( s );
event.getDropTargetContext().dropComplete( true );
}
else
{
event.rejectDrop();
}
}
catch( Exception exception )
{
System.err.println( "Exception" + exception.getMessage() );
event.rejectDrop();
}
}
/**
* is invoked if the use modifies the current drop gesture
*
*/
public void dropActionChanged( DropTargetDragEvent event )
{
}
/**
* a drag gesture has been initiated
*
*/
public void dragGestureRecognized( DragGestureEvent event )
{
Object selected = getSelectedValue();
if( selected != null )
{
StringSelection text = new StringSelection( selected.toString() );
// as the name suggests, starts the dragging
dragSource.startDrag( event, DragSource.DefaultMoveDrop, text, this );
}
else
{
System.out.println( "nothing was selected" );
}
}
/**
* this message goes to DragSourceListener, informing it that the dragging
* has ended
*
*/
public void dragDropEnd( DragSourceDropEvent event )
{
if( event.getDropSuccess() )
{
removeElement();
}
}
/**
* this message goes to DragSourceListener, informing it that the dragging
* has entered the DropSite
*
*/
public void dragEnter( DragSourceDragEvent event )
{
System.out.println( " dragEnter" );
}
/**
* this message goes to DragSourceListener, informing it that the dragging
* has exited the DropSite
*
*/
public void dragExit( DragSourceEvent event )
{
System.out.println( "dragExit" );
}
/**
* this message goes to DragSourceListener, informing it that the dragging is
* currently ocurring over the DropSite
*
*/
public void dragOver( DragSourceDragEvent event )
{
System.out.println( "dragExit" );
}
/**
* is invoked when the user changes the dropAction
*
*/
public void dropActionChanged( DragSourceDragEvent event )
{
System.out.println( "dropActionChanged" );
}
/**
* adds elements to itself
*
*/
public void addElement( Object s )
{
( ( DefaultListModel )getModel() ).addElement( s.toString() );
}
/**
* removes an element from itself
*/
public void removeElement()
{
( ( DefaultListModel )getModel() ).removeElement( getSelectedValue() );
}
}
14.112.Drag Drop |
| 14.112.1. | Basic drag and drop |
| 14.112.2. | Dragging Text from a JLabel | 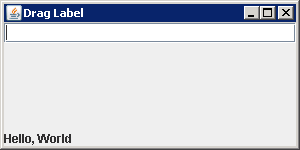 |
| 14.112.3. | Drag-and-Drop Support for Images | 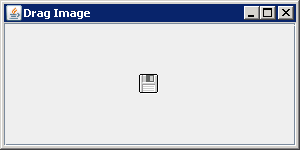 |
| 14.112.4. | Drag and drop icons: use an icon property. |
| 14.112.5. | implements DragGestureListener, Transferable |
| 14.112.6. | Dragging and dropping text between a text area, a list, and a table | 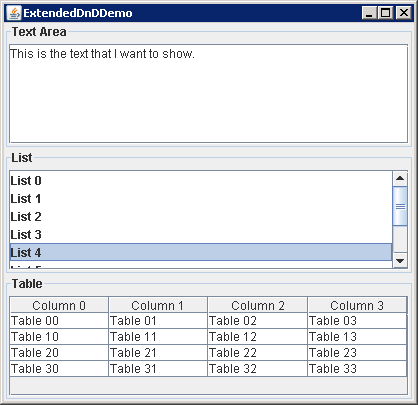 |
| 14.112.7. | Drag and drop between JTextArea and JTextField |
| 14.112.8. | Transfer both Text and Color between JTextField and JTextArea |
| 14.112.9. | Drag and drop between JList and JTextField |
| 14.112.10. | DropMode.ON |
| 14.112.11. | DropMode.INSERT |
| 14.112.12. | DropMode.ON_OR_INSERT |
| 14.112.13. | Set tree DropMode to DropMode.USE_SELECTION |
| 14.112.14. | Set tree drag mode to DropMode.ON |
| 14.112.15. | Set tree drag mode to DropMode.INSERT |
| 14.112.16. | Set tree drag mode to DropMode.ON_OR_INSERT |
| 14.112.17. | Choose Drop Action |
| 14.112.18. | Various drop actions |
| 14.112.19. | JTable drag and drop |
| 14.112.20. | Create a drag source a drop target and a transferable object. |
| 14.112.21. | Making a Component Draggable |
| 14.112.22. | Detect a drag initiating gesture in your application |
| 14.112.23. | Illustrates cut, copy, paste and drag and drop using three instances of JList |
| 14.112.24. | Location sensitive drag and drop |
| 14.112.25. | Demonstration of the top-level TransferHandler support on JFrame |
| 14.112.26. | Drag-and-Drop customization: drag the foreground color from the first label and drop it as the background color into the second one |
| 14.112.27. | Demonstrates how to add copy and drag support to a Swing component with TransferHandler |
| 14.112.28. | ScribblePane allows individual PolyLine lines to be selected, cut, copied, pasted, dragged, and dropped |
| 14.112.29. | Built-in drag and drop support: utilize a TransferHandler class |
| 14.112.30. | DND Drag and drop List |