To transfer something other than a simple property, you need to create an
implementation of the Transferable interface,
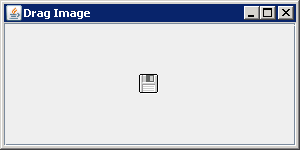
import java.awt.BorderLayout;
import java.awt.Image;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.io.IOException;
import javax.swing.AbstractButton;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JScrollPane;
import javax.swing.TransferHandler;
class ImageSelection extends TransferHandler implements Transferable {
private static final DataFlavor flavors[] = { DataFlavor.imageFlavor };
private Image image;
public int getSourceActions(JComponent c) {
return TransferHandler.COPY;
}
public boolean canImport(JComponent comp, DataFlavor flavor[]) {
if (!(comp instanceof JLabel) && !(comp instanceof AbstractButton)) {
return false;
}
for (int i = 0, n = flavor.length; i < n; i++) {
for (int j = 0, m = flavors.length; j < m; j++) {
if (flavor[i].equals(flavors[j])) {
return true;
}
}
}
return false;
}
public Transferable createTransferable(JComponent comp) {
// Clear
image = null;
if (comp instanceof JLabel) {
JLabel label = (JLabel) comp;
Icon icon = label.getIcon();
if (icon instanceof ImageIcon) {
image = ((ImageIcon) icon).getImage();
return this;
}
} else if (comp instanceof AbstractButton) {
AbstractButton button = (AbstractButton) comp;
Icon icon = button.getIcon();
if (icon instanceof ImageIcon) {
image = ((ImageIcon) icon).getImage();
return this;
}
}
return null;
}
public boolean importData(JComponent comp, Transferable t) {
if (comp instanceof JLabel) {
JLabel label = (JLabel) comp;
if (t.isDataFlavorSupported(flavors[0])) {
try {
image = (Image) t.getTransferData(flavors[0]);
ImageIcon icon = new ImageIcon(image);
label.setIcon(icon);
return true;
} catch (UnsupportedFlavorException ignored) {
} catch (IOException ignored) {
}
}
} else if (comp instanceof AbstractButton) {
AbstractButton button = (AbstractButton) comp;
if (t.isDataFlavorSupported(flavors[0])) {
try {
image = (Image) t.getTransferData(flavors[0]);
ImageIcon icon = new ImageIcon(image);
button.setIcon(icon);
return true;
} catch (UnsupportedFlavorException ignored) {
} catch (IOException ignored) {
}
}
}
return false;
}
// Transferable
public Object getTransferData(DataFlavor flavor) {
if (isDataFlavorSupported(flavor)) {
return image;
}
return null;
}
public DataFlavor[] getTransferDataFlavors() {
return flavors;
}
public boolean isDataFlavorSupported(DataFlavor flavor) {
return flavors[0].equals(flavor);
}
}
public class DragImage {
public static void main(String args[]) {
JFrame frame = new JFrame("Drag Image");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Icon icon = new ImageIcon("yourFile.gif");
JLabel label = new JLabel(icon);
label.setTransferHandler(new ImageSelection());
MouseListener listener = new MouseAdapter() {
public void mousePressed(MouseEvent me) {
JComponent comp = (JComponent) me.getSource();
TransferHandler handler = comp.getTransferHandler();
handler.exportAsDrag(comp, me, TransferHandler.COPY);
}
};
label.addMouseListener(listener);
frame.add(new JScrollPane(label), BorderLayout.CENTER);
frame.setSize(300, 150);
frame.setVisible(true);
}
}
14.112.Drag Drop |
| 14.112.1. | Basic drag and drop |
| 14.112.2. | Dragging Text from a JLabel | 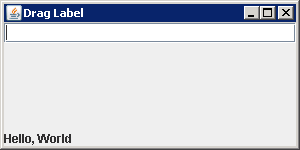 |
| 14.112.3. | Drag-and-Drop Support for Images | 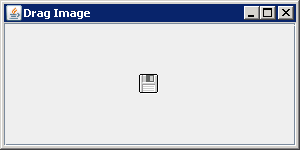 |
| 14.112.4. | Drag and drop icons: use an icon property. |
| 14.112.5. | implements DragGestureListener, Transferable |
| 14.112.6. | Dragging and dropping text between a text area, a list, and a table | 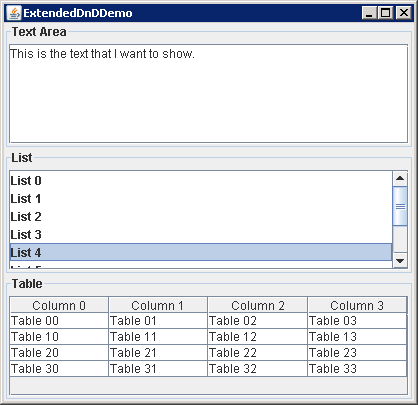 |
| 14.112.7. | Drag and drop between JTextArea and JTextField |
| 14.112.8. | Transfer both Text and Color between JTextField and JTextArea |
| 14.112.9. | Drag and drop between JList and JTextField |
| 14.112.10. | DropMode.ON |
| 14.112.11. | DropMode.INSERT |
| 14.112.12. | DropMode.ON_OR_INSERT |
| 14.112.13. | Set tree DropMode to DropMode.USE_SELECTION |
| 14.112.14. | Set tree drag mode to DropMode.ON |
| 14.112.15. | Set tree drag mode to DropMode.INSERT |
| 14.112.16. | Set tree drag mode to DropMode.ON_OR_INSERT |
| 14.112.17. | Choose Drop Action |
| 14.112.18. | Various drop actions |
| 14.112.19. | JTable drag and drop |
| 14.112.20. | Create a drag source a drop target and a transferable object. |
| 14.112.21. | Making a Component Draggable |
| 14.112.22. | Detect a drag initiating gesture in your application |
| 14.112.23. | Illustrates cut, copy, paste and drag and drop using three instances of JList |
| 14.112.24. | Location sensitive drag and drop |
| 14.112.25. | Demonstration of the top-level TransferHandler support on JFrame |
| 14.112.26. | Drag-and-Drop customization: drag the foreground color from the first label and drop it as the background color into the second one |
| 14.112.27. | Demonstrates how to add copy and drag support to a Swing component with TransferHandler |
| 14.112.28. | ScribblePane allows individual PolyLine lines to be selected, cut, copied, pasted, dragged, and dropped |
| 14.112.29. | Built-in drag and drop support: utilize a TransferHandler class |
| 14.112.30. | DND Drag and drop List |