The Struts and Tags
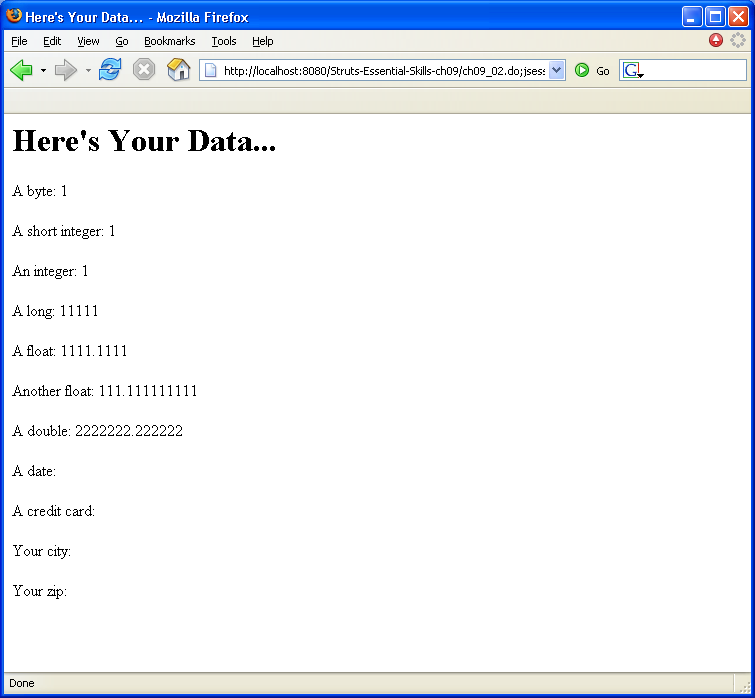
/*
Title: Struts : Essential Skills (Essential Skills)
Authors: Steven Holzner
Publisher: McGraw-Hill Osborne Media
ISBN: 0072256591
*/
//ch09_01.jsp
<%@ taglib uri="/WEB-INF/struts-bean.tld" prefix="bean" %>
<%@ taglib uri="/WEB-INF/struts-html.tld" prefix="html" %>
<%@ taglib uri="/WEB-INF/struts-logic.tld" prefix="logic" %>
<html:html>
<head>
<title>Using the Struts Validator</title>
<html:base/>
</head>
<body>
<h1>Using the Struts Validator</h1>
<logic:messagesPresent>
<bean:message key="errors.header"/>
<ul>
<html:messages id="error" property="byte">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="short">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="integer">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="long">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="float">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="floatRange">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="double">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="date">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="creditCard">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="city">
<li><bean:write name="error"/></li>
</html:messages>
<html:messages id="error" property="zip">
<li><bean:write name="error"/></li>
</html:messages>
</ul>
<hr>
</logic:messagesPresent>
<html:form action="ch09_02">
<bean:message key="ch09_03.byte.text"/>
<html:text property="byte" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.short.text"/>
<html:text property="short" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.integer.text"/>
<html:text property="integer" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.long.text"/>
<html:text property="long" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.float.text"/>
<html:text property="float" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.floatRange.text"/>
<html:text property="floatRange" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.double.text"/>
<html:text property="double" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.date.text"/>
<html:text property="date" size="15" maxlength="15"/>
<br>
<br>
<bean:message key="ch09_03.creditCard.text"/>
<html:text property="creditCard" size="16" maxlength="16"/>
<br>
<br>
<bean:message key="ch09_03.city.text"/>
<html:text property="city" size="16" maxlength="16"/>
<br>
<br>
<bean:message key="ch09_03.zip.text"/>
<html:text property="zip" size="5" maxlength="5"/>
<br>
<br>
<html:submit property="submit">
Submit
</html:submit>
<html:reset>
Reset
</html:reset>
</html:form>
</body>
</html:html>
//ch09_04.jsp
<%@ taglib uri="/WEB-INF/struts-bean.tld" prefix="bean" %>
<HTML>
<HEAD>
<TITLE>Here's Your Data...</TITLE>
</HEAD>
<BODY>
<H1>Here's Your Data...</H1>
<bean:message key="ch09_03.byte.text"/>:
<bean:write name="ch09_03" property="byte"/>
<BR>
<BR>
<bean:message key="ch09_03.short.text"/>:
<bean:write name="ch09_03" property="short"/>
<BR>
<BR>
<bean:message key="ch09_03.integer.text"/>:
<bean:write name="ch09_03" property="integer"/>
<BR>
<BR>
<bean:message key="ch09_03.long.text"/>:
<bean:write name="ch09_03" property="long"/>
<BR>
<BR>
<bean:message key="ch09_03.float.text"/>:
<bean:write name="ch09_03" property="float"/>
<BR>
<BR>
<bean:message key="ch09_03.floatRange.text"/>:
<bean:write name="ch09_03" property="floatRange"/>
<BR>
<BR>
<bean:message key="ch09_03.double.text"/>:
<bean:write name="ch09_03" property="double"/>
<BR>
<BR>
<bean:message key="ch09_03.date.text"/>:
<bean:write name="ch09_03" property="date"/>
<BR>
<BR>
<bean:message key="ch09_03.creditCard.text"/>:
<bean:write name="ch09_03" property="creditCard"/>
<BR>
<BR>
<bean:message key="ch09_03.city.text"/>:
<bean:write name="ch09_03" property="city"/>
<BR>
<BR>
<bean:message key="ch09_03.zip.text"/>:
<bean:write name="ch09_03" property="zip"/>
<BR>
</BODY>
</HTML>
package ch09;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts.action.*;
public final class ch09_02 extends Action {
public ActionForward execute(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws Exception
{
return mapping.findForward("success");
}
}
package ch09;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.validator.ValidatorForm;
public final class ch09_03 extends ValidatorForm {
private String byteData = null;
private String shortData = null;
private String integerData = null;
private String longData = null;
private String floatData = null;
private String floatDataRange = null;
private String doubleData = null;
private String dateData = null;
private String creditData = null;
private String cityData = null;
private String zipData = null;
public String getByte()
{
return byteData;
}
public void setByte(String byteData)
{
this.byteData = byteData;
}
public String getShort()
{
return shortData;
}
public void setShort(String shortData)
{
this.shortData = shortData;
}
public String getInteger()
{
return integerData;
}
public void setInteger(String integerData)
{
this.integerData = integerData;
}
public String getLong()
{
return longData;
}
public void setLong(String longData)
{
this.longData = longData;
}
public String getFloat()
{
return floatData;
}
public void setFloat(String floatData)
{
this.floatData = floatData;
}
public String getFloatRange()
{
return floatDataRange;
}
public void setFloatRange(String floatDataRange)
{
this.floatDataRange = floatDataRange;
}
public String getDouble()
{
return doubleData;
}
public void setDouble(String doubleData)
{
this.doubleData = doubleData;
}
public String getDate()
{
return dateData;
}
public void setDate(String dateData)
{
this.dateData = dateData;
}
public String getCreditCard()
{
return creditData;
}
public void setCreditCard(String creditData)
{
this.creditData = creditData;
}
public String getCity()
{
return cityData;
}
public void setCity(String cityData)
{
this.cityData = cityData;
}
public String getZip()
{
return zipData;
}
public void setZip(String zipData)
{
this.zipData = zipData;
}
public void reset(ActionMapping mapping, HttpServletRequest request)
{
byteData = null;
shortData = null;
integerData = null;
longData = null;
floatData = null;
floatDataRange = null;
doubleData = null;
dateData = null;
creditData = null;
cityData = null;
zipData = null;
}
}
Struts-Essential-Skills-ch09.zip( 1,439 k)Related examples in the same category