Struts: Creating the Model
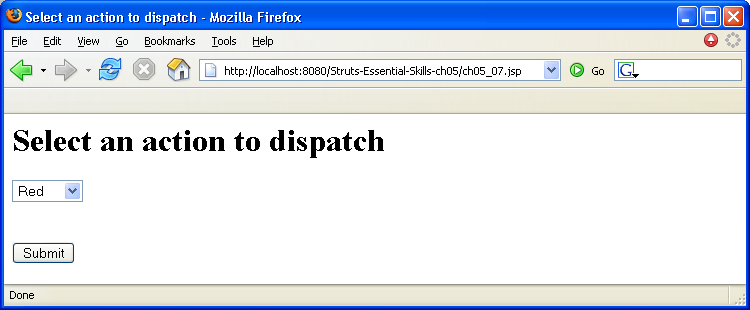
/*
Title: Struts : Essential Skills (Essential Skills)
Authors: Steven Holzner
Publisher: McGraw-Hill Osborne Media
ISBN: 0072256591
*/
//ch05_03.jsp
<%@ taglib uri="/tags/struts-bean" prefix="bean" %>
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<HTML>
<HEAD>
<TITLE>Using Include Actions</TITLE>
</HEAD>
<BODY>
<H1>Using Include Actions</H1>
<html:form action="ch05_04.do">
Enter some text
<html:text property="text"/>
<html:submit value="Submit"/>
</html:form>
</BODY>
</HTML>
//ch05_07.jsp
<%@ taglib uri="/tags/struts-bean" prefix="bean" %>
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<HTML>
<HEAD>
<TITLE>Select an action to dispatch</TITLE>
</HEAD>
<BODY>
<H1>Select an action to dispatch</H1>
<html:form action="ch05_05.do">
<html:select property="method">
<html:option value="red">Red</html:option>
<html:option value="yellow">Yellow</html:option>
<html:option value="green">Green</html:option>
</html:select>
<BR>
<BR>
<BR>
<html:submit />
</html:form>
</BODY>
</html>
//ch05_11.jsp
<%@ taglib uri="/tags/struts-bean" prefix="bean" %>
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<HTML>
<HEAD>
<TITLE>Using LookupDispatchAction</TITLE>
</HEAD>
<BODY>
<H1>Using LookupDispatchAction</H1>
<html:form action="ch05_09.do">
Select an action to dispatch:
<html:select property="method">
<html:option value="red">Red</html:option>
<html:option value="yellow">Yellow</html:option>
<html:option value="green">Green</html:option>
</html:select>
<BR>
<BR>
<BR>
<html:submit />
</html:form>
</BODY>
</html>
//ch05_08.jsp
<HTML>
<HEAD>
<TITLE>Success</TITLE>
</HEAD>
<BODY>
<H1>Success</H1>
The action has been dispatched.
</BODY>
</html>
//ch05_12.jsp
<HTML>
<HEAD>
<TITLE>Success</TITLE>
</HEAD>
<BODY>
<H1>Success</H1>
The action has been dispatched.
</BODY>
</HTML>
package ch05;
import java.io.*;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.ServletException;
import org.apache.struts.action.*;
import org.apache.struts.actions.DispatchAction;
public class ch05_05 extends DispatchAction
{
public ActionForward red(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
System.out.println("Stop.");
return mapping.findForward("success");
}
public ActionForward yellow(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
System.out.println("Caution.");
return mapping.findForward("success");
}
public ActionForward green(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
System.out.println("Go.");
return mapping.findForward("success");
}
}
package ch05;
import org.apache.struts.action.ActionForm;
public class ch05_02 extends ActionForm
{
private String text = "Hello";
public String getText()
{
return text;
}
public void setText(String text)
{
this.text = text;
}
}
package ch05;
import org.apache.struts.action.ActionForm;
public class ch05_10 extends ActionForm
{
private String method = "";
public String getmethod()
{
return method;
}
public void setMethod(String method)
{
this.method = method;
}
}
package ch05;
import java.io.*;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.ServletException;
import org.apache.struts.action.*;
import org.apache.struts.actions.LookupDispatchAction;
public class ch05_09 extends LookupDispatchAction
{
public ActionForward red(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
System.out.println("Stop.");
return mapping.findForward("success");
}
public ActionForward yellow(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
System.out.println("Caution.");
return mapping.findForward("success");
}
public ActionForward green(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
System.out.println("Go.");
return mapping.findForward("success");
}
protected Map getKeyMethodMap()
{
Map map = new HashMap();
map.put("ch05.red", "red");
map.put("ch05.yellow", "yellow");
map.put("ch05.green", "green");
return map;
}
}
package ch05;
import org.apache.struts.action.ActionForm;
public class ch05_06 extends ActionForm
{
private String method = "";
public String getmethod()
{
return method;
}
public void setMethod(String method)
{
this.method = method;
}
}
Struts-Essential-Skills-ch05.zip( 1,444 k)Related examples in the same category