A Full Struts Application
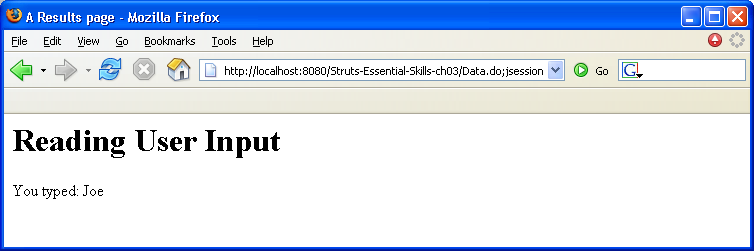
/*
Title: Struts : Essential Skills (Essential Skills)
Authors: Steven Holzner
Publisher: McGraw-Hill Osborne Media
ISBN: 0072256591
*/
//index.jsp
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<%@ taglib uri="/tags/struts-logic" prefix="logic" %>
<html:html locale="true">
<head>
<title>A Welcome Page</title>
<html:base/>
</head>
<body bgcolor="white">
<logic:notPresent name="org.apache.struts.action.MESSAGE" scope="application">
<font color="red">
ERROR: Application resources not loaded -- check servlet container
logs for error messages.
</font>
</logic:notPresent>
<h1>Reading User Input</h1>
<html:form action="Data">
Please type your name: <html:text property="text" />
<html:submit />
</html:form>
</body>
</html:html>
//ch03_01.jsp
<%@ taglib uri="/tags/struts-bean" prefix="bean" %>
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<%@ taglib uri="/tags/struts-logic" prefix="logic" %>
<%@ taglib uri="/ch03" prefix="ch03" %>
<HTML>
<HEAD>
<TITLE>The Struts Cafe</TITLE>
</HEAD>
<BODY>
<H1>The Struts Cafe</H1>
<html:errors/>
<ch03:items/>
<ch03:toppings/>
<html:form action="ch03_04.do">
<TABLE>
<TR>
<TD ALIGN="LEFT" VALIGN="TOP">
<bean:message key="toppings"/>
<BR>
<logic:iterate id="toppings1" name="toppings">
<html:multibox property="toppings">
<%= toppings1 %>
</html:multibox>
<%= toppings1 %>
<BR>
</logic:iterate>
</TD>
<TD ALIGN="LEFT" VALIGN="TOP">
<bean:message key="items"/>
<BR>
<html:select property="items">
<html:options name="items"/>
</html:select>
</TD>
</TR>
<TR>
<TD ALIGN="LEFT">
<BR>
<bean:message key="email"/>
<html:text property="email"/>
</TD>
<TR>
</TABLE>
<BR>
<html:submit value="Place Your Order!"/>
</html:form>
</BODY>
</html>
package ch03;
import ch03.DataForm;
import java.io.IOException;
import javax.servlet.*;
import javax.servlet.http.*;
import org.apache.struts.action.*;
public class DataAction extends Action {
public ActionForward execute(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
String text = null;
String target = new String("success");
if ( form != null ) {
DataForm dataForm = (DataForm)form;
text = dataForm.getText();
}
return (mapping.findForward(target));
}
}
package ch03;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.action.ActionError;
import org.apache.struts.action.ActionErrors;
public class DataForm extends ActionForm {
private String text = null;
public String getText() {
return (text);
}
public void setText(String text) {
this.text = text;
}
public void reset(ActionMapping mapping,
HttpServletRequest request) {
this.text = null;
}
// public ActionErrors validate(ActionMapping mapping,
// HttpServletRequest request) {
// ActionErrors errors = new ActionErrors();
// if ( (symbol == null ) || (symbol.length() == 0) ) {
// errors.add("symbol",
// new ActionError("errors.data.symbol.required"));
// }
// return errors;
// }
}
package ch03;
import java.util.*;
import javax.servlet.jsp.tagext.TagSupport;
public class ch03_02 extends TagSupport
{
public int doStartTag()
{
String[] itemsArray = {"", "Pizza", "Calzone", "Sandwich"};
pageContext.setAttribute("items", itemsArray);
return SKIP_BODY;
}
}
package ch03;
import java.util.*;
import javax.servlet.jsp.tagext.TagSupport;
public class ch03_03 extends TagSupport
{
public int doStartTag()
{
String[] toppingsArray = {"Pepperoni", "Hamburger", "Sausage", "Ham", "Cheese"};
pageContext.setAttribute("toppings", toppingsArray);
return SKIP_BODY;
}
}
package ch03;
import java.io.*;
import java.util.*;
import ch03.ch03_06;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.ServletException;
import org.apache.struts.action.*;
public class ch03_04 extends Action
{
public ActionForward execute(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
ActionErrors actionerrors = new ActionErrors();
ch03_06 orderForm = (ch03_06)form;
String email = orderForm.getEmail();
if(email.trim().equals("")) {
actionerrors.add(ActionErrors.GLOBAL_ERROR, new ActionError("error.noemail"));
}
String items = orderForm.getItems();
if(items.trim().equals("")) {
actionerrors.add("ActionErrors.GLOBAL_ERROR", new ActionError("error.noitems"));
}
String[] toppings = orderForm.getToppings();
if(toppings == null) {
actionerrors.add("ActionErrors.GLOBAL_ERROR", new ActionError("error.notoppings"));
}
if(actionerrors.size() != 0) {
saveErrors(request, actionerrors);
return new ActionForward(mapping.getInput());
}
return mapping.findForward("OK");
}
}
Struts-Essential-Skills-ch03.zip( 1,446 k)Related examples in the same category