Struts Framework: A Sample Struts Application
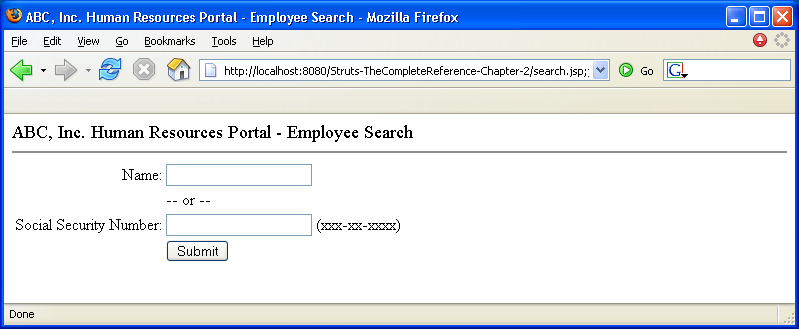
/*
# Title: Struts: The Complete Reference
# Author(s): James Holmes
# Publisher: McGraw-Hill/Osborne, 2004
# ISBN: 0-07-223131-9
*/
//index.jsp
<%@ taglib uri="/WEB-INF/tlds/struts-html.tld" prefix="html" %>
<html>
<head>
<title>ABC, Inc. Human Resources Portal</title>
</head>
<body>
<font size="+1">ABC, Inc. Human Resources Portal</font><br>
<hr width="100%" noshade="true">
• Add an Employee<br>
• <html:link forward="search">Search for Employees</html:link><br>
</body>
</html>
//search.jsp
<%@ taglib uri="/WEB-INF/tlds/struts-bean.tld" prefix="bean" %>
<%@ taglib uri="/WEB-INF/tlds/struts-html.tld" prefix="html" %>
<%@ taglib uri="/WEB-INF/tlds/struts-logic.tld" prefix="logic" %>
<html>
<head>
<title>ABC, Inc. Human Resources Portal - Employee Search</title>
</head>
<body>
<font size="+1">
ABC, Inc. Human Resources Portal - Employee Search
</font><br>
<hr width="100%" noshade="true">
<html:errors/>
<html:form action="/search">
<table>
<tr>
<td align="right"><bean:message key="label.search.name"/>:</td>
<td><html:text property="name"/></td>
</tr>
<tr>
<td></td>
<td>-- or --</td>
</tr>
<tr>
<td align="right"><bean:message key="label.search.ssNum"/>:</td>
<td><html:text property="ssNum"/> (xxx-xx-xxxx)</td>
</tr>
<tr>
<td></td>
<td><html:submit/></td>
</tr>
</table>
</html:form>
<logic:present name="searchForm" property="results">
<hr width="100%" size="1" noshade="true">
<bean:size id="size" name="searchForm" property="results"/>
<logic:equal name="size" value="0">
<center><font color="red"><b>No Employees Found</b></font></center>
</logic:equal>
<logic:greaterThan name="size" value="0">
<table border="1">
<tr>
<th>Name</th>
<th>Social Security Number</th>
</tr>
<logic:iterate id="result" name="searchForm" property="results">
<tr>
<td><bean:write name="result" property="name"/></td>
<td><bean:write name="result" property="ssNum"/></td>
</tr>
</logic:iterate>
</table>
</logic:greaterThan>
</logic:present>
</body>
</html>
package com.jamesholmes.minihr;
public class Employee
{
private String name;
private String ssNum;
public Employee(String name, String ssNum) {
this.name = name;
this.ssNum = ssNum;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setSsNum(String ssNum) {
this.ssNum = ssNum;
}
public String getSsNum() {
return ssNum;
}
}
package com.jamesholmes.minihr;
import java.util.ArrayList;
public class EmployeeSearchService
{
/* Hard-coded sample data. Normally this would come from a real data
source such as a database. */
private static Employee[] employees =
{
new Employee("Bob Davidson", "123-45-6789"),
new Employee("Mary Williams", "987-65-4321"),
new Employee("Jim Smith", "111-11-1111"),
new Employee("Beverly Harris", "222-22-2222"),
new Employee("Thomas Frank", "333-33-3333"),
new Employee("Jim Davidson", "444-44-4444")
};
// Search for employees by name.
public ArrayList searchByName(String name) {
ArrayList resultList = new ArrayList();
for (int i = 0; i < employees.length; i++) {
if (employees[i].getName().toUpperCase().indexOf(name.toUpperCase()) != -1) {
resultList.add(employees[i]);
}
}
return resultList;
}
// Search for employee by social security number.
public ArrayList searchBySsNum(String ssNum) {
ArrayList resultList = new ArrayList();
for (int i = 0; i < employees.length; i++) {
if (employees[i].getSsNum().equals(ssNum)) {
resultList.add(employees[i]);
}
}
return resultList;
}
}
package com.jamesholmes.minihr;
import java.util.ArrayList;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts.action.Action;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
public final class SearchAction extends Action
{
public ActionForward execute(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws Exception
{
EmployeeSearchService service = new EmployeeSearchService();
ArrayList results;
SearchForm searchForm = (SearchForm) form;
// Perform employee search based on what criteria was entered.
String name = searchForm.getName();
if (name != null && name.trim().length() > 0) {
results = service.searchByName(name);
} else {
results = service.searchBySsNum(searchForm.getSsNum().trim());
}
// Place search results in SearchForm for access by JSP.
searchForm.setResults(results);
// Forward control to this Action's input page.
return mapping.getInputForward();
}
}
package com.jamesholmes.minihr;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts.action.ActionError;
import org.apache.struts.action.ActionErrors;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionMapping;
public class SearchForm extends ActionForm
{
private String name = null;
private String ssNum = null;
private List results = null;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setSsNum(String ssNum) {
this.ssNum = ssNum;
}
public String getSsNum() {
return ssNum;
}
public void setResults(List results) {
this.results = results;
}
public List getResults() {
return results;
}
// Reset form fields.
public void reset(ActionMapping mapping, HttpServletRequest request)
{
name = null;
ssNum = null;
results = null;
}
// Validate form data.
public ActionErrors validate(ActionMapping mapping,
HttpServletRequest request)
{
ActionErrors errors = new ActionErrors();
boolean nameEntered = false;
boolean ssNumEntered = false;
// Determine if name has been entered.
if (name != null && name.length() > 0) {
nameEntered = true;
}
// Determine if social security number has been entered.
if (ssNum != null && ssNum.length() > 0) {
ssNumEntered = true;
}
/* Validate that either name or social security number
has been entered. */
if (!nameEntered && !ssNumEntered) {
errors.add(null,
new ActionError("error.search.criteria.missing"));
}
/* Validate format of social security number if
it has been entered. */
if (ssNumEntered && !isValidSsNum(ssNum.trim())) {
errors.add("ssNum",
new ActionError("error.search.ssNum.invalid"));
}
return errors;
}
// Validate format of social security number.
private static boolean isValidSsNum(String ssNum) {
if (ssNum.length() < 11) {
return false;
}
for (int i = 0; i < 11; i++) {
if (i == 3 || i == 6) {
if (ssNum.charAt(i) != '-') {
return false;
}
} else if ("0123456789".indexOf(ssNum.charAt(i)) == -1) {
return false;
}
}
return true;
}
}
Struts-TheCompleteReference-Chapter-2.zip( 1,439 k)Related examples in the same category