Returns color based on 0-9 scale ranging from green to yellow
/**
* Utilities for GUI work.
* @author Alex Kurzhanskiy
* @version $Id: UtilGUI.java 38 2010-02-08 22:59:00Z akurzhan $
*/
import java.awt.Color;
public class Util {
/**
* Returns color based on 0-9 scale ranging from green to yellow.
*/
public static Color gyColor(int i) {
int[][] rgb = {
{0, 164, 0},
{19, 174, 0},
{41, 184, 0},
{65, 194, 0},
{91, 204, 0},
{119, 215, 0},
{150, 225, 0},
{183, 235, 0},
{218, 245, 0},
{255, 255, 0}
};
int ii = 0;
if (i > 9)
ii = 9;
else
ii = Math.max(i, ii);
float[] hsb = Color.RGBtoHSB(rgb[ii][0], rgb[ii][1], rgb[ii][2], null);
return Color.getHSBColor(hsb[0], hsb[1], hsb[2]);
}
/**
* Returns n-dimensional array of colors for given nx3 integer array of RGB values.
*/
public static Color[] getColorScale(int[][] rgb) {
if (rgb == null)
return null;
Color[] clr = new Color[rgb.length];
for (int i = 0; i < rgb.length; i++) {
float[] hsb = Color.RGBtoHSB(rgb[i][0], rgb[i][1], rgb[i][2], null);
clr[i] = Color.getHSBColor(hsb[0], hsb[1], hsb[2]);
}
return clr;
}
}
Related examples in the same category
1. | Color class is used to work with colors in Java 2D | | |
2. | Color Utilities: common color operations | | |
3. | Color Difference | | |
4. | Rainbow Color | | 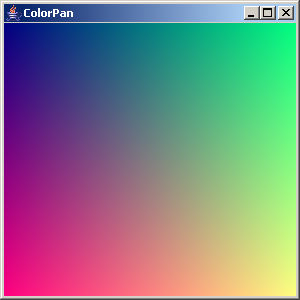 |
5. | XOR color | | 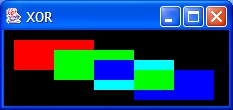 |
6. | Color Gradient | | |
7. | Common color utilities | | |
8. | Drawing with Color | | |
9. | Color fading animation | | |
10. | 140 colors - defined for X Window System listed in O'Reilly html pocket reference 87pp | | |
11. | Color Util | | |
12. | Color Factory | | |
13. | An efficient color quantization algorithm | | |
14. | Utility for checking colors given either hexa or natural language string descriptions. | | |
15. | Derives a color by adding the specified offsets to the base color's hue, saturation, and brightness values | | |
16. | Map colors into names and vice versa. | | |
17. | Converts a given string into a color. | | |
18. | If the color is equal to one of the defined constant colors, that name is returned instead. | | |
19. | Converts the String representation of a color to an actual Color object. | | |
20. | Returns blue-yellow-red color scale | | |
21. | Returns green-yellow-red-black color scale | | |
22. | Returns black-red-yellow-green color scale | | |
23. | Returns color based on 0-9 scale ranging from yellow to red | | |
24. | Returns color based on 0-9 scale ranging from black to green | | |
25. | Returns n-dimensional array of colors for given nx3 integer array of RGB values | | |
26. | Web color enum | | |
27. | Utility class for managing resources such as colors, fonts, images, etc. | | |
28. | Make a color transparent | | |
29. | Return a Color object given a string representation of it | | |
30. | Return a string representation of a color | | |
31. | Serializes a color to its HTML markup (e.g. "#ff0000" for red) | | |
32. | Parses a java.awt.Color from an HTML color string in the form '#RRGGBB' where RR, GG, and BB are the red, green, and blue bytes in hexadecimal form | | |
33. | Performs a somewhat subjective analysis of a color to determine how dark it looks to a user | | |
34. | Lightens a color by a given amount | | |
35. | Darkens a color by a given amount | | |
36. | Blend two colors | | |
37. | Utility for working with natively-ordered integer-packed RGBA-format colours. | | |
38. | HSV to RGB | | |
39. | A widget to manipulate an RGBA colour. | | |