Color Util
/*
* Copyright (C) 2004 NNL Technology AB
* Visit www.infonode.net for information about InfoNode(R)
* products and how to contact NNL Technology AB.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston,
* MA 02111-1307, USA.
*/
// $Id: ColorUtil.java,v 1.10 2005/02/16 11:28:14 jesper Exp $
import java.awt.*;
public final class ColorUtil {
private ColorUtil() {
}
public static Color getOpposite(Color c) {
return isDark(c) ? Color.WHITE : Color.BLACK;
}
public static Color shade(Color c, double amount) {
return blend(c, getOpposite(c), amount);
}
public static final Color mult(Color c, double amount) {
return c == null ? null : new Color(Math.min(255, (int) (c.getRed() * amount)),
Math.min(255, (int) (c.getGreen() * amount)),
Math.min(255, (int) (c.getBlue() * amount)),
c.getAlpha());
}
public static Color setAlpha(Color c, int alpha) {
return c == null ? null : new Color(c.getRed(), c.getGreen(), c.getBlue(), alpha);
}
public static final Color add(Color c1, Color c2) {
return c1 == null ? c2 :
c2 == null ? c1 :
new Color(Math.min(255, c1.getRed() + c2.getRed()),
Math.min(255, c1.getGreen() + c2.getGreen()),
Math.min(255, c1.getBlue() + c2.getBlue()),
c1.getAlpha());
}
public static Color blend(Color c1, Color c2, double v) {
double v2 = 1 - v;
return c1 == null ? (c2 == null ? null : c2) :
c2 == null ? c1 :
new Color(Math.min(255, (int) (c1.getRed() * v2 + c2.getRed() * v)),
Math.min(255, (int) (c1.getGreen() * v2 + c2.getGreen() * v)),
Math.min(255, (int) (c1.getBlue() * v2 + c2.getBlue() * v)),
Math.min(255, (int) (c1.getAlpha() * v2 + c2.getAlpha() * v)));
}
public static boolean isDark(Color c) {
return c.getRed() + c.getGreen() + c.getBlue() < 3 * 180;
}
public static Color highlight(Color c) {
return mult(c, isDark(c) ? 1.5F : 0.67F);
}
public static Color copy(Color c) {
return c == null ? null : new Color(c.getRed(), c.getGreen(), c.getBlue(), c.getAlpha());
}
}
Related examples in the same category
1. | Color class is used to work with colors in Java 2D | | |
2. | Color Utilities: common color operations | | |
3. | Color Difference | | |
4. | Rainbow Color | | 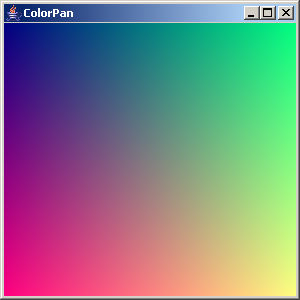 |
5. | XOR color | | 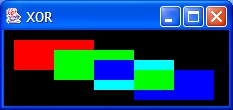 |
6. | Color Gradient | | |
7. | Common color utilities | | |
8. | Drawing with Color | | |
9. | Color fading animation | | |
10. | 140 colors - defined for X Window System listed in O'Reilly html pocket reference 87pp | | |
11. | Color Factory | | |
12. | An efficient color quantization algorithm | | |
13. | Utility for checking colors given either hexa or natural language string descriptions. | | |
14. | Derives a color by adding the specified offsets to the base color's hue, saturation, and brightness values | | |
15. | Map colors into names and vice versa. | | |
16. | Converts a given string into a color. | | |
17. | If the color is equal to one of the defined constant colors, that name is returned instead. | | |
18. | Converts the String representation of a color to an actual Color object. | | |
19. | Returns blue-yellow-red color scale | | |
20. | Returns green-yellow-red-black color scale | | |
21. | Returns black-red-yellow-green color scale | | |
22. | Returns color based on 0-9 scale ranging from green to yellow | | |
23. | Returns color based on 0-9 scale ranging from yellow to red | | |
24. | Returns color based on 0-9 scale ranging from black to green | | |
25. | Returns n-dimensional array of colors for given nx3 integer array of RGB values | | |
26. | Web color enum | | |
27. | Utility class for managing resources such as colors, fonts, images, etc. | | |
28. | Make a color transparent | | |
29. | Return a Color object given a string representation of it | | |
30. | Return a string representation of a color | | |
31. | Serializes a color to its HTML markup (e.g. "#ff0000" for red) | | |
32. | Parses a java.awt.Color from an HTML color string in the form '#RRGGBB' where RR, GG, and BB are the red, green, and blue bytes in hexadecimal form | | |
33. | Performs a somewhat subjective analysis of a color to determine how dark it looks to a user | | |
34. | Lightens a color by a given amount | | |
35. | Darkens a color by a given amount | | |
36. | Blend two colors | | |
37. | Utility for working with natively-ordered integer-packed RGBA-format colours. | | |
38. | HSV to RGB | | |
39. | A widget to manipulate an RGBA colour. | | |