HSV to RGB
//package org.boticelli.util;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class ColorHelper
{
private final static int H_STEPS = 12;
private final static int S_STEPS = 3;
private final static float S_STEP_VALUE = 0.05f;
private final static float S_MIN_VALUE = 0.15f;
private final static List<String> staticColors ;
static
{
List<String> colors = new ArrayList<String>();
float v = 1.0f;
for (int j = 0; j < H_STEPS; j++)
{
float h = j * 6.0f / H_STEPS;
for (int i = 0; i < S_STEPS; i++)
{
float s = S_MIN_VALUE + (i * S_STEP_VALUE);
float[] rgb = HSVtoRGB(h, s, v);
int r = (int) (rgb[0] * 255.0f);
int g = (int) (rgb[1] * 255.0f);
int b = (int) (rgb[2] * 255.0f);
String col = Integer.toHexString((r << 16) + (g << 8) + b);
col = "000000".substring(col.length()) + col;
colors.add("#"+col);
}
}
staticColors = Collections.unmodifiableList(colors);
}
public int getColorIndexFor(String ident)
{
return Math.abs(ident.hashCode()) % staticColors.size();
}
public static float[] HSVtoRGB(float h, float s, float v)
{
// H is given on [0->6] or -1. S and V are given on [0->1].
// RGB are each returned on [0->1].
float m, n, f;
int i;
float[] hsv = new float[3];
float[] rgb = new float[3];
hsv[0] = h;
hsv[1] = s;
hsv[2] = v;
if (hsv[0] == -1)
{
rgb[0] = rgb[1] = rgb[2] = hsv[2];
return rgb;
}
i = (int) (Math.floor(hsv[0]));
f = hsv[0] - i;
if (i % 2 == 0)
{
f = 1 - f; // if i is even
}
m = hsv[2] * (1 - hsv[1]);
n = hsv[2] * (1 - hsv[1] * f);
switch (i)
{
case 6:
case 0:
rgb[0] = hsv[2];
rgb[1] = n;
rgb[2] = m;
break;
case 1:
rgb[0] = n;
rgb[1] = hsv[2];
rgb[2] = m;
break;
case 2:
rgb[0] = m;
rgb[1] = hsv[2];
rgb[2] = n;
break;
case 3:
rgb[0] = m;
rgb[1] = n;
rgb[2] = hsv[2];
break;
case 4:
rgb[0] = n;
rgb[1] = m;
rgb[2] = hsv[2];
break;
case 5:
rgb[0] = hsv[2];
rgb[1] = m;
rgb[2] = n;
break;
}
return rgb;
}
public static List<String> getColors()
{
return staticColors;
}
}
Related examples in the same category
1. | Color class is used to work with colors in Java 2D | | |
2. | Color Utilities: common color operations | | |
3. | Color Difference | | |
4. | Rainbow Color | | 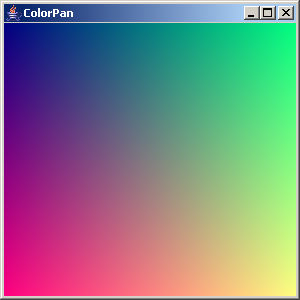 |
5. | XOR color | | 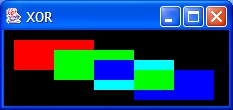 |
6. | Color Gradient | | |
7. | Common color utilities | | |
8. | Drawing with Color | | |
9. | Color fading animation | | |
10. | 140 colors - defined for X Window System listed in O'Reilly html pocket reference 87pp | | |
11. | Color Util | | |
12. | Color Factory | | |
13. | An efficient color quantization algorithm | | |
14. | Utility for checking colors given either hexa or natural language string descriptions. | | |
15. | Derives a color by adding the specified offsets to the base color's hue, saturation, and brightness values | | |
16. | Map colors into names and vice versa. | | |
17. | Converts a given string into a color. | | |
18. | If the color is equal to one of the defined constant colors, that name is returned instead. | | |
19. | Converts the String representation of a color to an actual Color object. | | |
20. | Returns blue-yellow-red color scale | | |
21. | Returns green-yellow-red-black color scale | | |
22. | Returns black-red-yellow-green color scale | | |
23. | Returns color based on 0-9 scale ranging from green to yellow | | |
24. | Returns color based on 0-9 scale ranging from yellow to red | | |
25. | Returns color based on 0-9 scale ranging from black to green | | |
26. | Returns n-dimensional array of colors for given nx3 integer array of RGB values | | |
27. | Web color enum | | |
28. | Utility class for managing resources such as colors, fonts, images, etc. | | |
29. | Make a color transparent | | |
30. | Return a Color object given a string representation of it | | |
31. | Return a string representation of a color | | |
32. | Serializes a color to its HTML markup (e.g. "#ff0000" for red) | | |
33. | Parses a java.awt.Color from an HTML color string in the form '#RRGGBB' where RR, GG, and BB are the red, green, and blue bytes in hexadecimal form | | |
34. | Performs a somewhat subjective analysis of a color to determine how dark it looks to a user | | |
35. | Lightens a color by a given amount | | |
36. | Darkens a color by a given amount | | |
37. | Blend two colors | | |
38. | Utility for working with natively-ordered integer-packed RGBA-format colours. | | |
39. | A widget to manipulate an RGBA colour. | | |