Return a Color object given a string representation of it
/*
* SSHTools - Java SSH2 API
*
* Copyright (C) 2002-2003 Lee David Painter and Contributors.
*
* Contributions made by:
*
* Brett Smith
* Richard Pernavas
* Erwin Bolwidt
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
import java.awt.Color;
public class Util{
/**
* Return a <code>Color</code> object given a string representation of it
*
* @param color
* @return string representation
* @throws IllegalArgumentException if string in bad format
*/
public static Color stringToColor(String s) {
try {
return new Color(Integer.decode("0x" + s.substring(1, 3)).intValue(),
Integer.decode("0x" + s.substring(3, 5)).intValue(),
Integer.decode("0x" + s.substring(5, 7)).intValue());
} catch (Exception e) {
return null;
}
}
}
Related examples in the same category
1. | Color class is used to work with colors in Java 2D | | |
2. | Color Utilities: common color operations | | |
3. | Color Difference | | |
4. | Rainbow Color | | 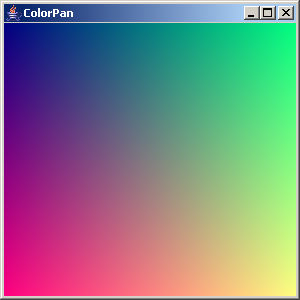 |
5. | XOR color | | 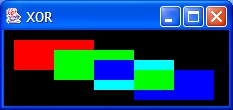 |
6. | Color Gradient | | |
7. | Common color utilities | | |
8. | Drawing with Color | | |
9. | Color fading animation | | |
10. | 140 colors - defined for X Window System listed in O'Reilly html pocket reference 87pp | | |
11. | Color Util | | |
12. | Color Factory | | |
13. | An efficient color quantization algorithm | | |
14. | Utility for checking colors given either hexa or natural language string descriptions. | | |
15. | Derives a color by adding the specified offsets to the base color's hue, saturation, and brightness values | | |
16. | Map colors into names and vice versa. | | |
17. | Converts a given string into a color. | | |
18. | If the color is equal to one of the defined constant colors, that name is returned instead. | | |
19. | Converts the String representation of a color to an actual Color object. | | |
20. | Returns blue-yellow-red color scale | | |
21. | Returns green-yellow-red-black color scale | | |
22. | Returns black-red-yellow-green color scale | | |
23. | Returns color based on 0-9 scale ranging from green to yellow | | |
24. | Returns color based on 0-9 scale ranging from yellow to red | | |
25. | Returns color based on 0-9 scale ranging from black to green | | |
26. | Returns n-dimensional array of colors for given nx3 integer array of RGB values | | |
27. | Web color enum | | |
28. | Utility class for managing resources such as colors, fonts, images, etc. | | |
29. | Make a color transparent | | |
30. | Return a string representation of a color | | |
31. | Serializes a color to its HTML markup (e.g. "#ff0000" for red) | | |
32. | Parses a java.awt.Color from an HTML color string in the form '#RRGGBB' where RR, GG, and BB are the red, green, and blue bytes in hexadecimal form | | |
33. | Performs a somewhat subjective analysis of a color to determine how dark it looks to a user | | |
34. | Lightens a color by a given amount | | |
35. | Darkens a color by a given amount | | |
36. | Blend two colors | | |
37. | Utility for working with natively-ordered integer-packed RGBA-format colours. | | |
38. | HSV to RGB | | |
39. | A widget to manipulate an RGBA colour. | | |