Set text to TextBlock for selected list item
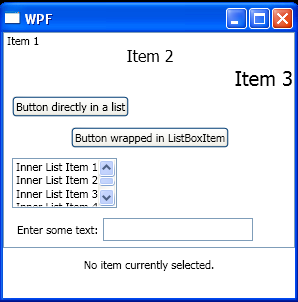
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="300" Width="300">
<StackPanel>
<ListBox SelectionChanged="OuterListBox_SelectionChanged" Name="outerListBox">
<ListBoxItem Content="Item 1" FontFamily="Tahoma" HorizontalContentAlignment="Left" />
<ListBoxItem Content="Item 2" FontFamily="Algerian" FontSize="16" HorizontalContentAlignment="Center" />
<ListBoxItem Content="Item 3" FontSize="20" HorizontalContentAlignment="Right" />
<Button Content="Button directly in a list" Margin="5" />
<ListBoxItem HorizontalContentAlignment="Center" Margin="5">
<Button Content="Button wrapped in ListBoxItem" />
</ListBoxItem>
<ListBox Height="50" Margin="5">
<ListBoxItem Content="Inner List Item 1" Selected="InnerListBoxItem_Selected" />
<ListBoxItem Content="Inner List Item 2" Selected="InnerListBoxItem_Selected" />
<ListBoxItem Content="Inner List Item 3" Selected="InnerListBoxItem_Selected" />
<ListBoxItem Content="Inner List Item 4" Selected="InnerListBoxItem_Selected" />
</ListBox>
<StackPanel Margin="5" Orientation="Horizontal">
<Label Content="Enter some text:" />
<TextBox MinWidth="150" />
</StackPanel>
</ListBox>
<TextBlock Text="No item currently selected." Margin="10" HorizontalAlignment="Center" Name="txtSelectedItem" />
</StackPanel>
</Window>
//File:Window.xaml.cs
using System.Windows;
using System.Windows.Controls;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void InnerListBoxItem_Selected(object sender, RoutedEventArgs e)
{
ListBoxItem item = e.OriginalSource as ListBoxItem;
if (item != null)
{
MessageBox.Show(item.Content + " was selected.", Title);
}
}
private void OuterListBox_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
object item = outerListBox.SelectedItem;
if (item == null)
{
txtSelectedItem.Text = "No item currently selected.";
}
else
{
txtSelectedItem.Text = item.ToString();
}
}
}
}
Related examples in the same category