Convert the contents of a ListBoxItem to an instance of GridLength by using GridLengthConverter
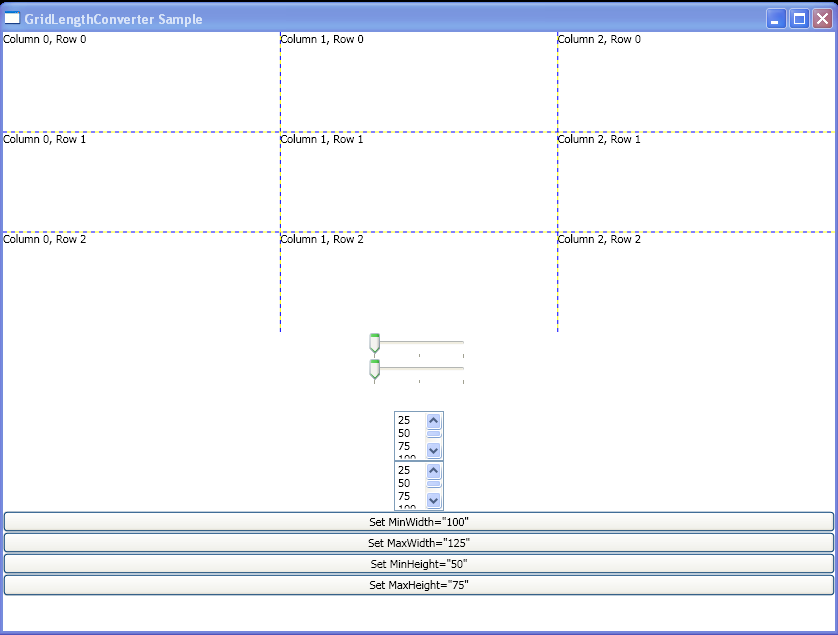
<Window
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="GridLengthConverter_grid.Window1"
Title="GridLengthConverter Sample"
WindowState="Maximized">
<StackPanel>
<Grid Name="grid1" ShowGridLines="True" Height="300">
<Grid.ColumnDefinitions>
<ColumnDefinition Name="col1" />
<ColumnDefinition Name="col2" />
<ColumnDefinition Name="col3" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Name="row1" />
<RowDefinition Name="row2" />
<RowDefinition Name="row3" />
</Grid.RowDefinitions>
<TextBlock Grid.Row="0" Grid.Column="0" TextWrapping="Wrap">Column 0, Row 0</TextBlock>
<TextBlock Grid.Row="0" Grid.Column="1" TextWrapping="Wrap">Column 1, Row 0</TextBlock>
<TextBlock Grid.Row="0" Grid.Column="2" TextWrapping="Wrap">Column 2, Row 0</TextBlock>
<TextBlock Grid.Row="1" Grid.Column="0" TextWrapping="Wrap">Column 0, Row 1</TextBlock>
<TextBlock Grid.Row="1" Grid.Column="1" TextWrapping="Wrap">Column 1, Row 1</TextBlock>
<TextBlock Grid.Row="1" Grid.Column="2" TextWrapping="Wrap">Column 2, Row 1</TextBlock>
<TextBlock Grid.Row="2" Grid.Column="0" TextWrapping="Wrap">Column 0, Row 2</TextBlock>
<TextBlock Grid.Row="2" Grid.Column="1" TextWrapping="Wrap">Column 1, Row 2</TextBlock>
<TextBlock Grid.Row="2" Grid.Column="2" TextWrapping="Wrap">Column 2, Row 2</TextBlock>
</Grid>
<Slider Name="hs1" Width="100" IsSnapToTickEnabled="True" Maximum="2" TickPlacement="BottomRight" TickFrequency="1" ValueChanged="changeColVal"/>
<Slider Orientation="Horizontal" Name="hs2" Width="100" IsSnapToTickEnabled="True" Maximum="2" TickPlacement="BottomRight" TickFrequency="1" ValueChanged="changeRowVal" />
<TextBlock Name="txt1" TextWrapping="Wrap"/>
<TextBlock Name="txt2" TextWrapping="Wrap"/>
<ListBox Width="50" Height="50" SelectionChanged="changeCol">
<ListBoxItem>25</ListBoxItem>
<ListBoxItem>50</ListBoxItem>
<ListBoxItem>75</ListBoxItem>
<ListBoxItem>100</ListBoxItem>
<ListBoxItem>125</ListBoxItem>
<ListBoxItem>150</ListBoxItem>
</ListBox>
<ListBox Width="50" Height="50" SelectionChanged="changeRow">
<ListBoxItem>25</ListBoxItem>
<ListBoxItem>50</ListBoxItem>
<ListBoxItem>75</ListBoxItem>
<ListBoxItem>100</ListBoxItem>
<ListBoxItem>125</ListBoxItem>
<ListBoxItem>150</ListBoxItem>
</ListBox>
<Button Name="btn1" Click="setMinWidth">Set MinWidth="100"</Button>
<Button Name="btn2" Click="setMaxWidth">Set MaxWidth="125"</Button>
<Button Name="btn3" Click="setMinHeight">Set MinHeight="50"</Button>
<Button Name="btn4" Click="setMaxHeight">Set MaxHeight="75"</Button>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
namespace GridLengthConverter_grid
{
public partial class Window1 : Window
{
public void changeRowVal(object sender, RoutedEventArgs e)
{
txt2.Text = "Current Grid Row is " + hs2.Value.ToString();
}
public void changeColVal(object sender, RoutedEventArgs e)
{
txt1.Text = "Current Grid Column is " + hs1.Value.ToString();
}
public void changeCol(object sender, SelectionChangedEventArgs args)
{
ListBoxItem li = ((sender as ListBox).SelectedItem as ListBoxItem);
GridLengthConverter myGridLengthConverter = new GridLengthConverter();
if (hs1.Value == 0)
{
GridLength gl1 = (GridLength)myGridLengthConverter.ConvertFromString(li.Content.ToString());
col1.Width = gl1;
}
else if (hs1.Value == 1)
{
GridLength gl2 = (GridLength)myGridLengthConverter.ConvertFromString(li.Content.ToString());
col2.Width = gl2;
}
else if (hs1.Value == 2)
{
GridLength gl3 = (GridLength)myGridLengthConverter.ConvertFromString(li.Content.ToString());
col3.Width = gl3;
}
}
public void changeRow(object sender, SelectionChangedEventArgs args)
{
ListBoxItem li2 = ((sender as ListBox).SelectedItem as ListBoxItem);
GridLengthConverter myGridLengthConverter2 = new GridLengthConverter();
if (hs2.Value == 0)
{
GridLength gl4 = (GridLength)myGridLengthConverter2.ConvertFromString(li2.Content.ToString());
row1.Height = gl4;
}
else if (hs2.Value == 1)
{
GridLength gl5 = (GridLength)myGridLengthConverter2.ConvertFromString(li2.Content.ToString());
row2.Height = gl5;
}
else if (hs2.Value == 2)
{
GridLength gl6 = (GridLength)myGridLengthConverter2.ConvertFromString(li2.Content.ToString());
row3.Height = gl6;
}
}
public void setMinWidth(object sender, RoutedEventArgs e)
{
col1.MinWidth = 100;
col2.MinWidth = 100;
col3.MinWidth = 100;
}
public void setMaxWidth(object sender, RoutedEventArgs e)
{
col1.MaxWidth = 125;
col2.MaxWidth = 125;
col3.MaxWidth = 125;
}
public void setMinHeight(object sender, RoutedEventArgs e)
{
row1.MinHeight = 50;
row2.MinHeight = 50;
row3.MinHeight = 50;
}
public void setMaxHeight(object sender, RoutedEventArgs e)
{
row1.MaxHeight = 75;
row2.MaxHeight = 75;
row3.MaxHeight = 75;
}
}
}
Related examples in the same category