Rolling Ball Animation
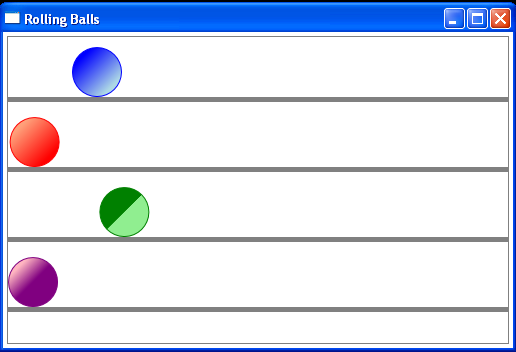
<Window x:Class="WpfApplication1.RollingBall"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Rolling Balls" Height="350" Width="518">
<Border BorderBrush="Gray" BorderThickness="1" Margin="4">
<Canvas>
<Rectangle Fill="Gray" Width="500" Height="5"
Canvas.Top="60" />
<Ellipse x:Name="ellipse1" Width="50" Height="50"
Stroke="Blue" Canvas.Top="10" Canvas.Left="0">
<Ellipse.Fill>
<LinearGradientBrush>
<GradientStop Color="Blue" Offset="0.2" />
<GradientStop Color="LightBlue" Offset="0.8" />
</LinearGradientBrush>
</Ellipse.Fill>
<Ellipse.RenderTransform>
<RotateTransform x:Name="ellipse1Rotate"
CenterX="25" CenterY="25" />
</Ellipse.RenderTransform>
</Ellipse>
<Rectangle Fill="Gray" Width="500" Height="5"
Canvas.Top="130" />
<Ellipse x:Name="ellipse2" Width="50" Height="50"
Stroke="Red" Canvas.Top="80" Canvas.Left="0">
<Ellipse.Fill>
<LinearGradientBrush>
<GradientStop Color="Red" Offset="0.8" />
<GradientStop Color="LightSalmon" Offset="0.2" />
</LinearGradientBrush>
</Ellipse.Fill>
<Ellipse.RenderTransform>
<RotateTransform x:Name="ellipse2Rotate"
CenterX="25" CenterY="25" />
</Ellipse.RenderTransform>
</Ellipse>
<Rectangle Fill="Gray" Width="500" Height="5"
Canvas.Top="200" />
<Ellipse x:Name="ellipse3" Width="50" Height="50"
Stroke="Green" Canvas.Top="150" Canvas.Left="0">
<Ellipse.Fill>
<LinearGradientBrush>
<GradientStop Color="Green" Offset="0.5" />
<GradientStop Color="LightGreen" Offset="0.5" />
</LinearGradientBrush>
</Ellipse.Fill>
<Ellipse.RenderTransform>
<RotateTransform x:Name="ellipse3Rotate"
CenterX="25" CenterY="25" />
</Ellipse.RenderTransform>
</Ellipse>
<Rectangle Fill="Gray" Width="500" Height="5" Canvas.Top="270" />
<Ellipse x:Name="ellipse4" Width="50" Height="50" Stroke="Purple" Canvas.Top="220" Canvas.Left="0">
<Ellipse.Fill>
<LinearGradientBrush>
<GradientStop Color="Purple" Offset="0.5" />
<GradientStop Color="LightPink" Offset="0.2" />
</LinearGradientBrush>
</Ellipse.Fill>
<Ellipse.RenderTransform>
<RotateTransform x:Name="ellipse4Rotate" CenterX="25" CenterY="25" />
</Ellipse.RenderTransform>
</Ellipse>
</Canvas>
</Border>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace WpfApplication1
{
public partial class RollingBall : Window
{
public RollingBall()
{
InitializeComponent();
double nRotation = 2;
DoubleAnimation da = new DoubleAnimation(0, 450,TimeSpan.FromSeconds(5));
da.RepeatBehavior = RepeatBehavior.Forever;
da.AutoReverse = true;
ellipse1.BeginAnimation(Canvas.LeftProperty, da);
da = new DoubleAnimation(0, nRotation,TimeSpan.FromSeconds(15));
da.RepeatBehavior = RepeatBehavior.Forever;
da.AutoReverse = true;
ellipse1Rotate.BeginAnimation(RotateTransform.AngleProperty, da);
da = new DoubleAnimation(0, 450,TimeSpan.FromSeconds(15));
da.AccelerationRatio = 0.4;
da.RepeatBehavior = RepeatBehavior.Forever;
da.AutoReverse = true;
ellipse2.BeginAnimation(Canvas.LeftProperty, da);
da = new DoubleAnimation(0, nRotation,TimeSpan.FromSeconds(15));
da.AccelerationRatio = 0.4;
da.RepeatBehavior = RepeatBehavior.Forever;
da.AutoReverse = true;
ellipse2Rotate.BeginAnimation(RotateTransform.AngleProperty, da);
da = new DoubleAnimation(0, 450,TimeSpan.FromSeconds(5));
da.DecelerationRatio = 0.6;
da.RepeatBehavior = RepeatBehavior.Forever;
da.AutoReverse = true;
ellipse3.BeginAnimation(Canvas.LeftProperty, da);
da = new DoubleAnimation(0, nRotation,TimeSpan.FromSeconds(15));
da.DecelerationRatio = 0.6;
da.RepeatBehavior = RepeatBehavior.Forever;
da.AutoReverse = true;
ellipse3Rotate.BeginAnimation(RotateTransform.AngleProperty, da);
}
}
}
Related examples in the same category