User Events
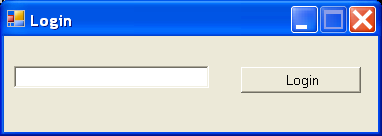
/*
Professional Windows GUI Programming Using C#
by Jay Glynn, Csaba Torok, Richard Conway, Wahid Choudhury,
Zach Greenvoss, Shripad Kulkarni, Neil Whitlow
Publisher: Peer Information
ISBN: 1861007663
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Diagnostics;
using System.Runtime.CompilerServices;
namespace UserEvents
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class UserEvents : System.Windows.Forms.Form
{
private System.Windows.Forms.TextBox txtUsername;
private System.Windows.Forms.Button btnLogin;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
LoginAuditInserter la = new LoginAuditInserter();
public UserEvents()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
la.LoginAudit += new LoginAuditInserter.LoginAuditHandler(AddAuditEntry);
la.LoginAudit += new LoginAuditInserter.LoginAuditHandler(AddEventLogEntry);
}
static public void AddAuditEntry(string username)
{
System.Diagnostics.Debug.WriteLine(username);
}
static public void AddEventLogEntry(string username)
{
string applicationName = "Login Audit";
EventLog ev = new EventLog("Application");
ev.Source = applicationName;
ev.WriteEntry("Login Attempted.", EventLogEntryType.Information);
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
la.LoginAudit -= new LoginAuditInserter.LoginAuditHandler(AddAuditEntry);
la.LoginAudit -= new LoginAuditInserter.LoginAuditHandler(AddEventLogEntry);
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.txtUsername = new System.Windows.Forms.TextBox();
this.btnLogin = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// txtUsername
//
this.txtUsername.Location = new System.Drawing.Point(8, 24);
this.txtUsername.Name = "txtUsername";
this.txtUsername.Size = new System.Drawing.Size(152, 20);
this.txtUsername.TabIndex = 0;
this.txtUsername.Text = "";
//
// btnLogin
//
this.btnLogin.Location = new System.Drawing.Point(184, 24);
this.btnLogin.Name = "btnLogin";
this.btnLogin.Size = new System.Drawing.Size(96, 23);
this.btnLogin.TabIndex = 1;
this.btnLogin.Text = "Login";
this.btnLogin.Click += new System.EventHandler(this.btnLogin_Click);
//
// UserEvents
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 78);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.btnLogin,
this.txtUsername});
this.MaximizeBox = false;
this.Name = "UserEvents";
this.Text = "Login";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new UserEvents());
}
private void btnLogin_Click(object sender, System.EventArgs e)
{
la.AddAuditEntry(txtUsername.Text);
}
}
public class LoginAuditInserter
{
public delegate void LoginAuditHandler(string username);
private AccessorContainer container = new AccessorContainer();
private static int key = 0;
public event LoginAuditHandler LoginAudit
{
[MethodImpl(MethodImplOptions.Synchronized)]
add
{
container.Add(key, value);
}
[MethodImpl(MethodImplOptions.Synchronized)]
remove
{
container.Remove(key, value);
}
}
protected void OnLoginAudit(string username)
{
LoginAuditHandler loginAudit = (LoginAuditHandler)container.Get(key);
if(username!=null)
{
loginAudit(username);
}
}
public void AddAuditEntry(string username)
{
OnLoginAudit(username);
}
}
public class AccessorContainer
{
private ArrayList arrayAccessor = new ArrayList();
public Delegate Get(int key)
{
return ((Delegate)arrayAccessor[key]);
}
public void Add(int key, Delegate ptr)
{
try
{
arrayAccessor[key] = Delegate.Combine((Delegate)arrayAccessor[key], ptr);
}
catch(ArgumentOutOfRangeException)
{
arrayAccessor.Add(ptr);
}
}
public void Remove(int key, Delegate ptr)
{
arrayAccessor.Remove(Delegate.Remove((Delegate)arrayAccessor[key], ptr));
}
}
}
Related examples in the same category