Label, TextBox and Button
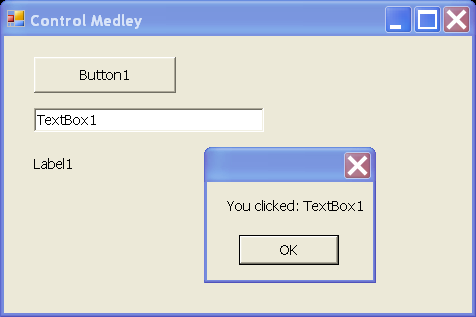
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace ControlMedley
{
/// <summary>
/// Summary description for ControlMedley.
/// </summary>
public class ControlMedley : System.Windows.Forms.Form
{
internal System.Windows.Forms.Label Label1;
internal System.Windows.Forms.TextBox TextBox1;
internal System.Windows.Forms.Button Button1;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public ControlMedley()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Label1 = new System.Windows.Forms.Label();
this.TextBox1 = new System.Windows.Forms.TextBox();
this.Button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// Label1
//
this.Label1.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Label1.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Label1.Location = new System.Drawing.Point(20, 92);
this.Label1.Name = "Label1";
this.Label1.Size = new System.Drawing.Size(112, 24);
this.Label1.TabIndex = 5;
this.Label1.Text = "Label1";
this.Label1.Click += new System.EventHandler(this.ctrlClick);
//
// TextBox1
//
this.TextBox1.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.TextBox1.Location = new System.Drawing.Point(20, 56);
this.TextBox1.Name = "TextBox1";
this.TextBox1.Size = new System.Drawing.Size(156, 21);
this.TextBox1.TabIndex = 4;
this.TextBox1.Text = "TextBox1";
this.TextBox1.Click += new System.EventHandler(this.ctrlClick);
//
// Button1
//
this.Button1.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button1.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Button1.Location = new System.Drawing.Point(20, 16);
this.Button1.Name = "Button1";
this.Button1.Size = new System.Drawing.Size(96, 28);
this.Button1.TabIndex = 3;
this.Button1.Text = "Button1";
this.Button1.Click += new System.EventHandler(this.ctrlClick);
//
// ControlMedley
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 14);
this.ClientSize = new System.Drawing.Size(316, 214);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.Label1,
this.TextBox1,
this.Button1});
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Name = "ControlMedley";
this.Text = "Control Medley";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new ControlMedley());
}
private void ctrlClick(System.Object sender, EventArgs e)
{
Control ctrl = (Control)sender;
MessageBox.Show("You clicked: " + ctrl.Name);
}
}
}
Related examples in the same category