TextBox location
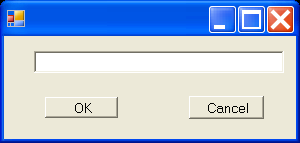
using System;
using System.Drawing;
using System.ComponentModel;
using System.Windows.Forms;
public class frmLogin : System.Windows.Forms.Form {
System.Windows.Forms.TextBox txtUser;
System.Windows.Forms.Button btnOK;
System.Windows.Forms.Button btnCancel;
public frmLogin() {
txtUser = new System.Windows.Forms.TextBox();
txtUser.Location = new Point(30, 15);
txtUser.Size = new Size(250, 20);
txtUser.Text = "";
txtUser.Name = "txtUser";
this.Controls.Add(txtUser);
btnOK = new System.Windows.Forms.Button();
btnOK.Location = new Point(40,(txtUser.Location.Y + txtUser.Size.Height + btnOK.Size.Height));
btnOK.Text = "OK";
btnOK.Name = "btnOK";
this.Controls.Add(btnOK);
btnCancel = new System.Windows.Forms.Button();
btnCancel.Location = new Point((this.Size.Width -
btnCancel.Size.Width) - 40,
(txtUser.Location.Y + txtUser.Size.Height + btnOK.Size.Height));
btnCancel.Text = "Cancel";
btnCancel.Name = "btnCancel";
this.Controls.Add(btnCancel);
this.Size = new Size(this.Size.Width, btnCancel.Location.Y +
btnCancel.Size.Height + 60);
btnCancel.Click += new System.EventHandler(btnCancelHandler);
btnOK.Click += new System.EventHandler(btnEventHandler);
}
private void btnEventHandler(object sender, System.EventArgs e) {
MessageBox.Show(((Button)sender).Name);
}
private void btnCancelHandler(object sender, System.EventArgs e) {
MessageBox.Show("The second handler");
}
[STAThread]
static void Main() {
Application.Run(new frmLogin());
}
}
Related examples in the same category