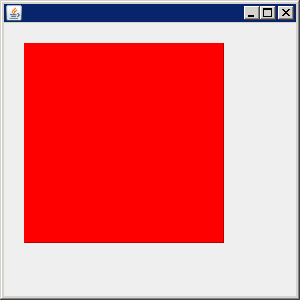
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JComponent;
import javax.swing.JFrame;
class MyCanvas extends JComponent {
public void paint(Graphics g) {
g.setColor(Color.RED);
g.fill3DRect(20, 20, 200, 200,true);
}
}
public class Fill3DRect {
public static void main(String[] a) {
JFrame window = new JFrame();
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
window.setBounds(30, 30, 300, 300);
window.getContentPane().add(new MyCanvas());
window.setVisible(true);
}
}
16.41.Rectangle |
| 16.41.1. | java.awt.Rectangle |
| 16.41.2. | Draw Rectangle | 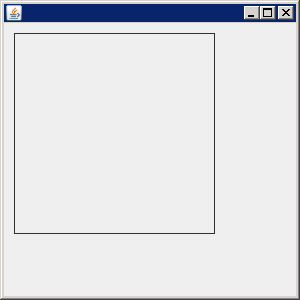 |
| 16.41.3. | drawRect (int, int, int, int) to draw a rectangle outline |
| 16.41.4. | drawRoundRect (int, int, int, int, int, int) to draw a rounded rectangle outline |
| 16.41.5. | Draw a three-dimensional rectangle outline |
| 16.41.6. | How do I...Fill shapes? | 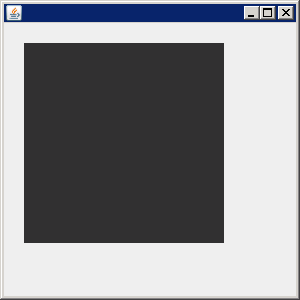 |
| 16.41.7. | Fill 3D Rectangle | 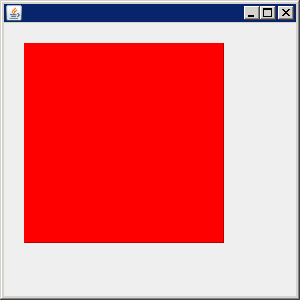 |
| 16.41.8. | Draw Rectangle with Rectangle2D.Float |
| 16.41.9. | Draw RoundRectangle2D.Float |
| 16.41.10. | Ractangle with rounded corners drawn using Java 2D Graphics API |
| 16.41.11. | Intersection between rectangles |
| 16.41.12. | Grow a rectangle |
| 16.41.13. | Union two Rectangles |
| 16.41.14. | Clips the specified line to the given rectangle. |
| 16.41.15. | Returns a point based on (x, y) but constrained to be within the bounds of a given rectangle. |
| 16.41.16. | Checks, whether the given rectangle1 fully contains rectangle 2 (even if rectangle 2 has a height or width of zero!). |