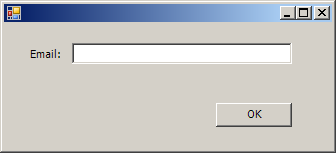
using System;
using System.Windows.Forms;
using System.Text.RegularExpressions;
public class TextBoxValidationRegex : Form
{
public TextBoxValidationRegex()
{
this.components = new System.ComponentModel.Container();
this.Button1 = new System.Windows.Forms.Button();
this.errProvider = new System.Windows.Forms.ErrorProvider(this.components);
this.Label3 = new System.Windows.Forms.Label();
this.txtEmail = new System.Windows.Forms.TextBox();
((System.ComponentModel.ISupportInitialize)(this.errProvider)).BeginInit();
this.SuspendLayout();
this.Button1.Location = new System.Drawing.Point(212, 80);
this.Button1.Name = "Button1";
this.Button1.Size = new System.Drawing.Size(76, 24);
this.Button1.TabIndex = 12;
this.Button1.Text = "OK";
this.Button1.Click += new System.EventHandler(this.Button1_Click);
this.errProvider.ContainerControl = this;
this.errProvider.DataMember = "";
this.Label3.Location = new System.Drawing.Point(24, 24);
this.Label3.Size = new System.Drawing.Size(40, 16);
this.Label3.Text = "Email:";
this.txtEmail.Location = new System.Drawing.Point(68, 20);
this.txtEmail.Size = new System.Drawing.Size(220, 21);
this.txtEmail.Leave += new System.EventHandler(this.txtEmail_Leave);
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(328, 126);
this.Controls.Add(this.Label3);
this.Controls.Add(this.txtEmail);
this.Controls.Add(this.Button1);
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
((System.ComponentModel.ISupportInitialize)(this.errProvider)).EndInit();
this.ResumeLayout(false);
this.PerformLayout();
}
private void Button1_Click(object sender, EventArgs e)
{
string errorText = "";
foreach (Control ctrl in this.Controls)
{
if (errProvider.GetError(ctrl) != "")
{
errorText += " * " + errProvider.GetError(ctrl) + "\n";
}
MessageBox.Show("Errors:" +errorText, "Invalid Input", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
}
private void txtEmail_Leave(object sender, EventArgs e)
{
Regex regex;
regex = new Regex(@"^[\w-]+@([\w-]+\.)+[\w-]+$");
Control ctrl = (Control)sender;
if (regex.IsMatch(ctrl.Text) || ctrl.Text == "")
{
errProvider.SetError(ctrl, "");
}
else
{
errProvider.SetError(ctrl, "This is not a valid email address.");
}
}
[STAThread]
public static void Main(string[] args)
{
Application.Run(new TextBoxValidationRegex());
}
private System.Windows.Forms.Button Button1;
private System.Windows.Forms.ErrorProvider errProvider;
private System.Windows.Forms.Label Label3;
private System.Windows.Forms.TextBox txtEmail;
private System.ComponentModel.IContainer components = null;
}