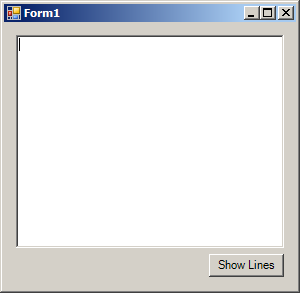
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void showLinesButton_Click(object sender, EventArgs e)
{
StringBuilder lineInfo = new StringBuilder();
lineInfo.Append("There are " + multiLineBox.Lines.Length.ToString() + " lines.\n");
foreach (string line in multiLineBox.Lines)
{
lineInfo.Append(line + "\n");
}
MessageBox.Show(lineInfo.ToString());
}
}
partial class Form1
{
private void InitializeComponent()
{
this.multiLineBox = new System.Windows.Forms.TextBox();
this.showLinesButton = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// multiLineBox
//
this.multiLineBox.Location = new System.Drawing.Point(12, 12);
this.multiLineBox.Multiline = true;
this.multiLineBox.Name = "multiLineBox";
this.multiLineBox.Size = new System.Drawing.Size(268, 213);
this.multiLineBox.TabIndex = 0;
//
// showLinesButton
//
this.showLinesButton.Location = new System.Drawing.Point(205, 231);
this.showLinesButton.Name = "showLinesButton";
this.showLinesButton.Size = new System.Drawing.Size(75, 23);
this.showLinesButton.TabIndex = 1;
this.showLinesButton.Text = "Show Lines";
this.showLinesButton.Click += new System.EventHandler(this.showLinesButton_Click);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.Add(this.showLinesButton);
this.Controls.Add(this.multiLineBox);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
this.PerformLayout();
}
private System.Windows.Forms.TextBox multiLineBox;
private System.Windows.Forms.Button showLinesButton;
}
public class TextBoxLineCountText
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.Run(new Form1());
}
}