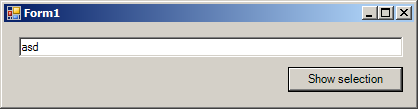
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void showButton_Click(object sender, EventArgs e)
{
if (inputBox.SelectionLength == 0)
{
MessageBox.Show("You need to select something inside the text box first");
}
else
{
System.Text.StringBuilder builder = new System.Text.StringBuilder();
builder.Append(String.Format("The input box contains {0}\n", inputBox.Text));
builder.Append(String.Format("You have selected {0} characters, starting at {1}\n", inputBox.SelectionLength, inputBox.SelectionStart));
builder.Append(String.Format("The selection is {0}", inputBox.SelectedText));
MessageBox.Show(builder.ToString());
}
}
}
partial class Form1
{
private void InitializeComponent()
{
this.inputBox = new System.Windows.Forms.TextBox();
this.showButton = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// inputBox
//
this.inputBox.Location = new System.Drawing.Point(16, 15);
this.inputBox.Name = "inputBox";
this.inputBox.Size = new System.Drawing.Size(421, 22);
this.inputBox.TabIndex = 0;
//
// showButton
//
this.showButton.Location = new System.Drawing.Point(311, 46);
this.showButton.Name = "showButton";
this.showButton.Size = new System.Drawing.Size(126, 27);
this.showButton.TabIndex = 1;
this.showButton.Text = "Show selection";
this.showButton.Click += new System.EventHandler(this.showButton_Click);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(6, 15);
this.ClientSize = new System.Drawing.Size(449, 87);
this.Controls.Add(this.showButton);
this.Controls.Add(this.inputBox);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
this.PerformLayout();
}
private System.Windows.Forms.TextBox inputBox;
private System.Windows.Forms.Button showButton;
}
public class TextBoxSelection
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.Run(new Form1());
}
}