using system colors to create gradients
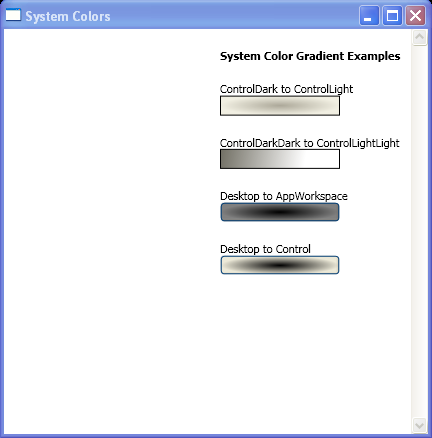
<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="SystemColorsAndBrushes_csharp.Window1"
Title="System Colors" >
<Window.Resources>
<Style TargetType="{x:Type Rectangle}">
<Setter Property="Margin" Value="10,0,10,0"/>
<Setter Property="HorizontalAlignment" Value="Left"/>
<Setter Property="Height" Value="20"/>
<Setter Property="Width" Value="120"/>
<Setter Property="Stroke" Value="Black"/>
<Setter Property="StrokeThickness" Value="1"/>
</Style>
<Style TargetType="{x:Type TextBlock}">
<Setter Property="Margin" Value="10,20,10,0"/>
</Style>
<Style TargetType="{x:Type Button}">
<Setter Property="Margin" Value="10,0,10,0"/>
<Setter Property="HorizontalAlignment" Value="Left"/>
</Style>
</Window.Resources>
<ScrollViewer>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition />
<ColumnDefinition Width="5" />
<ColumnDefinition />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition />
</Grid.RowDefinitions>
<Rectangle Grid.Column="1" Grid.Row="0"
HorizontalAlignment="Stretch" VerticalAlignment="Stretch" Fill="Black"
RadiusX="10" RadiusY="10" />
<StackPanel Name="systemBrushesPanel" Background="White" Grid.Row="0" Grid.Column="0"/>
<StackPanel Name="gradientExamplePanel" Background="White" Grid.Row="0" Grid.Column="2"/>
</Grid>
</ScrollViewer>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Documents
Imports System.Windows.Navigation
Imports System.Windows.Shapes
Imports System.Windows.Data
Namespace SystemColorsAndBrushes_csharp
Public Partial Class Window1
Inherits Window
Public Sub New()
InitializeComponent()
Dim t As New System.Windows.Controls.TextBlock()
t.Text = "System Color Gradient Examples"
t.HorizontalAlignment = System.Windows.HorizontalAlignment.Left
t.FontWeight = System.Windows.FontWeights.Bold
gradientExamplePanel.Children.Add(t)
t = New System.Windows.Controls.TextBlock()
t.Text = "ControlDark to ControlLight"
Dim r As New System.Windows.Shapes.Rectangle()
r.Fill = New System.Windows.Media.RadialGradientBrush(System.Windows.SystemColors.ControlDarkColor, System.Windows.SystemColors.ControlLightColor)
gradientExamplePanel.Children.Add(t)
gradientExamplePanel.Children.Add(r)
t = New System.Windows.Controls.TextBlock()
t.Text = "ControlDarkDark to ControlLightLight"
r = New System.Windows.Shapes.Rectangle()
r.Fill = New System.Windows.Media.LinearGradientBrush(System.Windows.SystemColors.ControlDarkDarkColor, System.Windows.SystemColors.ControlLightLightColor, 45)
gradientExamplePanel.Children.Add(t)
gradientExamplePanel.Children.Add(r)
' Try it out on a button.
t = New System.Windows.Controls.TextBlock()
t.Text = "Desktop to AppWorkspace"
Dim b As New System.Windows.Controls.Button()
b.Width = 120
b.Height = 20
b.Background = New System.Windows.Media.RadialGradientBrush(System.Windows.SystemColors.DesktopColor, System.Windows.SystemColors.AppWorkspaceColor)
gradientExamplePanel.Children.Add(t)
gradientExamplePanel.Children.Add(b)
t = New System.Windows.Controls.TextBlock()
t.Text = "Desktop to Control"
b = New System.Windows.Controls.Button()
b.Width = 120
b.Height = 20
b.Background = New System.Windows.Media.RadialGradientBrush(System.Windows.SystemColors.DesktopColor, System.Windows.SystemColors.ControlColor)
gradientExamplePanel.Children.Add(t)
gradientExamplePanel.Children.Add(b)
End Sub
End Class
End Namespace
Related examples in the same category