The default GroupStyle indents the items in a group
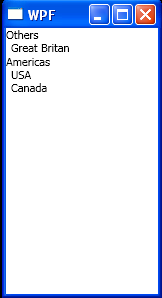
<Window
x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:WpfApplication1="clr-namespace:WpfApplication1"
Title="WPF" Height="300" Width="160">
<Window.Resources>
<WpfApplication1:Countries x:Key="countries"/>
<WpfApplication1:GroupByContinentConverter x:Key="GroupByContinentConverter"/>
<CollectionViewSource
x:Key="cvs"
Source="{Binding Source={StaticResource countries}}">
<CollectionViewSource.GroupDescriptions>
<PropertyGroupDescription Converter="{StaticResource GroupByContinentConverter}" />
</CollectionViewSource.GroupDescriptions>
</CollectionViewSource>
</Window.Resources>
<Grid>
<ItemsControl ItemsSource="{Binding Source={StaticResource cvs}}" DisplayMemberPath="Name" >
<ItemsControl.GroupStyle>
<x:Static Member="GroupStyle.Default"/>
</ItemsControl.GroupStyle>
</ItemsControl>
</Grid>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Globalization
Imports System.Windows.Data
Imports System.Collections.ObjectModel
Namespace WpfApplication1
Public Class GroupByContinentConverter
Implements IValueConverter
Public Function Convert(value As Object, targetType As Type, parameter As Object, culture As CultureInfo) As Object Implements IValueConverter.Convert
Dim country As Country = DirectCast(value, Country)
' Decide which group the country belongs in
Select Case country.Continent
Case Continent.NorthAmerica
Return "Americas"
Case Else
Return "Others"
End Select
End Function
Public Function ConvertBack(value As Object, targetType As Type, parameter As Object, culture As CultureInfo) As Object Implements IValueConverter.ConvertBack
Throw New NotImplementedException()
End Function
End Class
Public Class Country
Private m_name As String
Private m_continent As Continent
Public Sub New(name As String, continent As Continent)
Me.m_name = name
Me.m_continent = continent
End Sub
Public Property Name() As String
Get
Return m_name
End Get
Set
m_name = value
End Set
End Property
Public Property Continent() As Continent
Get
Return m_continent
End Get
Set
m_continent = value
End Set
End Property
End Class
Public Enum Continent
Europe
NorthAmerica
End Enum
Public Class Countries
Inherits Collection(Of Country)
Public Sub New()
Me.Add(New Country("Great Britan", Continent.Europe))
Me.Add(New Country("USA", Continent.NorthAmerica))
Me.Add(New Country("Canada", Continent.NorthAmerica))
End Sub
End Class
End Namespace
Related examples in the same category