Colors and Brushes
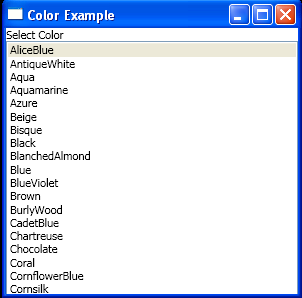
<Window x:Class="WpfApplication1.ColorExample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Color Example" Height="300" Width="300">
<StackPanel>
<TextBlock Text="Select Color"/>
<ListBox Name="listBox1" SelectionChanged="listBox1SelectionChanged"/>
<TextBlock Text="Show selected color:" Margin="5,5,5,0" />
<Rectangle x:Name="rect1" Stroke="Blue" Fill="AliceBlue"/>
<TextBlock Text="Opacity:" Margin="5,5,5,0" />
<TextBox x:Name="textBox" HorizontalAlignment="Left" TextAlignment="Center" Text="1" Width="50" Margin="5,5,5,8" />
<TextBlock FontWeight="Bold" Text="sRGB Information:" Margin="5,5,5,2" />
<TextBlock FontWeight="Bold" Text="ScRGB Information:" Margin="5,5,5,2" />
</StackPanel>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Shapes
Imports System.Reflection
Imports System.Collections.Generic
Namespace WpfApplication1
Public Partial Class ColorExample
Inherits Window
Private color As Color
Private colorBrush As New SolidColorBrush()
Public Sub New()
InitializeComponent()
Dim colorsType As Type = GetType(Colors)
For Each [property] As PropertyInfo In colorsType.GetProperties()
listBox1.Items.Add([property].Name)
color = Colors.AliceBlue
listBox1.SelectedIndex = 0
ColorInfo()
Next
End Sub
Private Sub listBox1SelectionChanged(sender As Object, e As EventArgs)
Dim colorString As String = listBox1.SelectedItem.ToString()
color = CType(ColorConverter.ConvertFromString(colorString), Color)
Dim opacity As Single = Convert.ToSingle(textBox.Text)
If opacity > 1F Then
opacity = 1F
ElseIf opacity < 0F Then
opacity = 0F
End If
color.ScA = opacity
ColorInfo()
End Sub
Private Sub ColorInfo()
rect1.Fill = New SolidColorBrush(color)
Console.WriteLine("Alpha = " & color.A.ToString())
Console.WriteLine("Red = " & color.R.ToString())
Console.WriteLine("Green = " & color.G.ToString())
Console.WriteLine("Blue = " & color.B.ToString())
Dim rgbHex As String = String.Format("{0:X2}{1:X2}{2:X2}{3:X2}", color.A, color.R, color.G, color.B)
Console.WriteLine("ARGB = #" & rgbHex)
Console.WriteLine("ScA = " & color.ScA.ToString())
Console.WriteLine("ScR = " & color.ScR.ToString())
Console.WriteLine("ScG = " & color.ScG.ToString())
Console.WriteLine("ScB = " & color.ScB.ToString())
End Sub
End Class
End Namespace
Related examples in the same category