Viewing RTF format
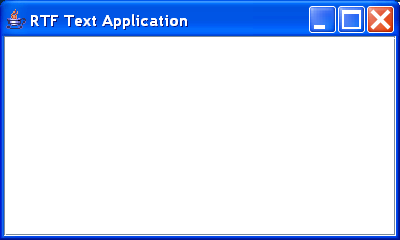
import java.awt.BorderLayout;
import java.awt.Color;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import javax.swing.JEditorPane;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.text.BadLocationException;
import javax.swing.text.rtf.RTFEditorKit;
public class RTFView extends JFrame {
public RTFView() {
setTitle("RTF Text Application");
setSize(400, 240);
setBackground(Color.gray);
getContentPane().setLayout(new BorderLayout());
JPanel topPanel = new JPanel();
topPanel.setLayout(new BorderLayout());
getContentPane().add(topPanel, BorderLayout.CENTER);
// Create an RTF editor window
RTFEditorKit rtf = new RTFEditorKit();
JEditorPane editor = new JEditorPane();
editor.setEditorKit(rtf);
editor.setBackground(Color.white);
// This text could be big so add a scroll pane
JScrollPane scroller = new JScrollPane();
scroller.getViewport().add(editor);
topPanel.add(scroller, BorderLayout.CENTER);
// Load an RTF file into the editor
try {
FileInputStream fi = new FileInputStream("test.rtf");
rtf.read(fi, editor.getDocument(), 0);
} catch (FileNotFoundException e) {
System.out.println("File not found");
} catch (IOException e) {
System.out.println("I/O error");
} catch (BadLocationException e) {
}
}
public static void main(String args[]) {
// Create an instance of the test application
RTFView mainFrame = new RTFView();
mainFrame.setVisible(true);
}
}
Related examples in the same category