How to use the check box button
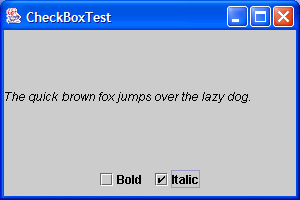
import java.awt.Font;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.JCheckBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class CheckBoxDemo extends JFrame implements ActionListener {
JLabel fontLabel = new JLabel("The quick brown fox jumps over the lazy dog.");
private JCheckBox bold= new JCheckBox("Bold");
private JCheckBox italic = new JCheckBox("Italic");
public CheckBoxDemo() {
setTitle("CheckBoxTest");
setSize(300, 200);
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
JPanel p = new JPanel();
p.add(bold);
p.add(italic);
bold.addActionListener(this);
italic.addActionListener(this);
getContentPane().add(p, "South");
getContentPane().add(fontLabel, "Center");
}
public void actionPerformed(ActionEvent evt) {
int m = (bold.isSelected() ? Font.BOLD : 0)
+ (italic.isSelected() ? Font.ITALIC : 0);
fontLabel.setFont(new Font("SansSerif", m, 12));
}
public static void main(String[] args) {
JFrame frame = new CheckBoxDemo();
frame.show();
}
}
Related examples in the same category