React to menu action and checkbox menu
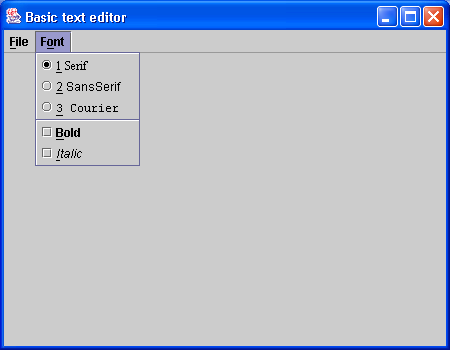
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
import java.io.File;
import javax.swing.ButtonGroup;
import javax.swing.ImageIcon;
import javax.swing.JCheckBoxMenuItem;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JRadioButtonMenuItem;
public class MenuDemo extends JFrame {
public static final String FontNames[] = { "Serif", "SansSerif", "Courier" };
protected Font fontArray[];
protected JMenuItem[] menus;
protected JCheckBoxMenuItem boldMenuItem;
protected JCheckBoxMenuItem italicMenuItem;
protected JFileChooser fileChooser;
public MenuDemo() {
super("Basic text editor");
setSize(450, 350);
fontArray = new Font[FontNames.length];
for (int i = 0; i < FontNames.length; i++)
fontArray[i] = new Font(FontNames[i], Font.PLAIN, 12);
JMenuBar menuBar = createMenuBar();
setJMenuBar(menuBar);
fileChooser = new JFileChooser();
fileChooser.setCurrentDirectory(new File("."));
WindowListener exitEvent = new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
};
addWindowListener(exitEvent);
updateMonitor();
setVisible(true);
}
protected JMenuBar createMenuBar() {
final JMenuBar menuBar = new JMenuBar();
JMenu menuFile = new JMenu("File");
menuFile.setMnemonic('f');
JMenuItem menuItem = new JMenuItem("New");
menuItem.setIcon(new ImageIcon("file_new.gif"));
menuItem.setMnemonic('n');
ActionListener lst = new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("New");
}
};
menuItem.addActionListener(lst);
menuFile.add(menuItem);
menuItem = new JMenuItem("Open...");
menuItem.setIcon(new ImageIcon("file_open.gif"));
menuItem.setMnemonic('o');
lst = new ActionListener() {
public void actionPerformed(ActionEvent e) {
MenuDemo.this.repaint();
if (fileChooser.showOpenDialog(MenuDemo.this) != JFileChooser.APPROVE_OPTION)
return;
System.out.println(fileChooser.getSelectedFile());
}
};
menuItem.addActionListener(lst);
menuFile.add(menuItem);
menuItem = new JMenuItem("Save...");
menuItem.setIcon(new ImageIcon("file_save.gif"));
menuItem.setMnemonic('s');
lst = new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Save...");
}
};
menuItem.addActionListener(lst);
menuFile.add(menuItem);
menuFile.addSeparator();
menuItem = new JMenuItem("Exit");
menuItem.setMnemonic('x');
lst = new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
};
menuItem.addActionListener(lst);
menuFile.add(menuItem);
menuBar.add(menuFile);
ActionListener fontListener = new ActionListener() {
public void actionPerformed(ActionEvent e) {
updateMonitor();
}
};
JMenu mFont = new JMenu("Font");
mFont.setMnemonic('o');
ButtonGroup group = new ButtonGroup();
menus = new JMenuItem[FontNames.length];
for (int i = 0; i < FontNames.length; i++) {
int m = i + 1;
menus[i] = new JRadioButtonMenuItem(m + " " + FontNames[i]);
boolean selected = (i == 0);
menus[i].setSelected(selected);
menus[i].setMnemonic('1' + i);
menus[i].setFont(fontArray[i]);
menus[i].addActionListener(fontListener);
group.add(menus[i]);
mFont.add(menus[i]);
}
mFont.addSeparator();
boldMenuItem = new JCheckBoxMenuItem("Bold");
boldMenuItem.setMnemonic('b');
Font fn = fontArray[1].deriveFont(Font.BOLD);
boldMenuItem.setFont(fn);
boldMenuItem.setSelected(false);
boldMenuItem.addActionListener(fontListener);
mFont.add(boldMenuItem);
italicMenuItem = new JCheckBoxMenuItem("Italic");
italicMenuItem.setMnemonic('i');
fn = fontArray[1].deriveFont(Font.ITALIC);
italicMenuItem.setFont(fn);
italicMenuItem.setSelected(false);
italicMenuItem.addActionListener(fontListener);
mFont.add(italicMenuItem);
menuBar.add(mFont);
return menuBar;
}
protected void updateMonitor() {
int index = -1;
for (int k = 0; k < menus.length; k++) {
if (menus[k].isSelected()) {
index = k;
break;
}
}
if (index == -1)
return;
if (index == 2) // Courier
{
boldMenuItem.setSelected(false);
boldMenuItem.setEnabled(false);
italicMenuItem.setSelected(false);
italicMenuItem.setEnabled(false);
} else {
boldMenuItem.setEnabled(true);
italicMenuItem.setEnabled(true);
}
int style = Font.PLAIN;
if (boldMenuItem.isSelected())
style |= Font.BOLD;
if (italicMenuItem.isSelected())
style |= Font.ITALIC;
}
public static void main(String argv[]) {
new MenuDemo();
}
}
Related examples in the same category