Verify Area Code
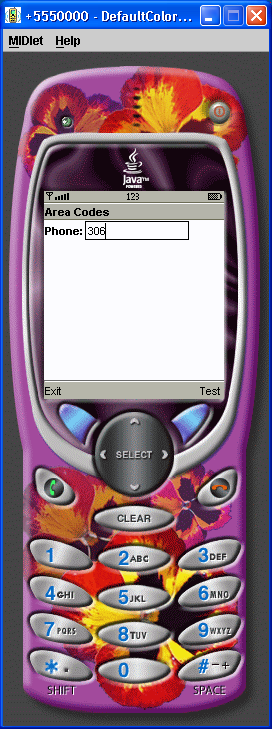
/*--------------------------------------------------
* VerifyAreaCode.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class VerifyAreaCode extends MIDlet implements CommandListener
{
private Display display; // Reference to Display object
private Form fmMain; // The main form
private Command cmTest; // Next label and message
private Command cmExit; // Command to exit the MIDlet
private TextField tfPhone; // Phone number
private String areaCodeTable [][] = {
{"512", "912"}, // Old area code, new area code
{"717", "917"} };
public VerifyAreaCode()
{
display = Display.getDisplay(this);
// Create commands
cmTest = new Command("Test", Command.SCREEN, 1);
cmExit = new Command("Exit", Command.EXIT, 1);
// Textfield for phone number
tfPhone = new TextField("Phone:", "", 10, TextField.PHONENUMBER);
// Create Form, add Commands & textfield, listen for events
fmMain = new Form("Area Codes");
fmMain.addCommand(cmExit);
fmMain.addCommand(cmTest);
fmMain.append(tfPhone);
fmMain.setCommandListener(this);
}
// Called by application manager to start the MIDlet.
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{ }
public void destroyApp(boolean unconditional)
{ }
public void commandAction(Command c, Displayable s)
{
if (c == cmTest)
{
if (tfPhone.size() == 10)
{
char buffer[] = new char[10];
// Get phone number into byte array
tfPhone.getChars(buffer);
// Call method to check the area code table.
// Create a new StringItem to display,
// passing in 'null' as the StringItem
StringItem tmp = new StringItem(null, ("The area code " + (areaCodeLookup(buffer) ? "has" : "has not") + " been updated."));
// Place at the end of the form
if (fmMain.size() == 1) // Only tfPhone on form
fmMain.append(tmp);
else // Replace previous StringItem
fmMain.set(1, tmp);
}
}
else if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
/*--------------------------------------------------
* Compare the area code the user entered with the
* area code table. If a match is found, replace
* the user's code with the new code from the table
*-------------------------------------------------*/
private boolean areaCodeLookup(char [] buffer)
{
// Get the area code (only) from the users entry
String str = new String(buffer, 0, 3);
for (int x = 0; x < areaCodeTable.length; x++)
{
// If we find a match in the table
if (str.equals(areaCodeTable[x][0]))
{
// Delete the area code
tfPhone.delete(0, 3);
// Insert the new area code
tfPhone.insert(areaCodeTable[x][1].toCharArray(), 0, 3, 0);
return true;
}
}
return false;
}
}
Related examples in the same category