Form Scroll
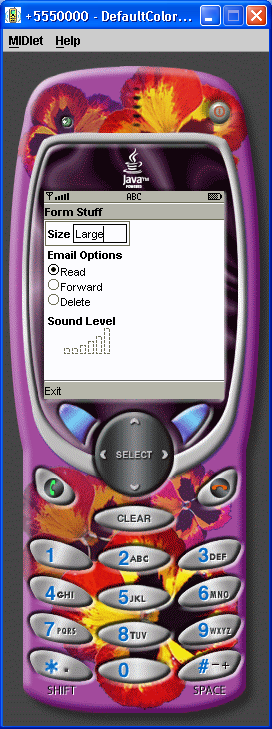
/*--------------------------------------------------
* FormScroll.java
*
* Show how a Form component handles scrolling
* when multiple Items are on the display
*
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class FormScroll extends MIDlet implements CommandListener
{
private Display display; // Reference to display object
private Form frmMain; // The main form
private Command cmdExit; // Command to exit
private TextField txfSize; // Product size
private ChoiceGroup chgEmail; // Choice group
private Gauge gauVolume; // Volume
public FormScroll()
{
display = Display.getDisplay(this);
// Create TextField
txfSize = new TextField("Size", "Large", 5, TextField.ANY);
// Create an exclusive (radio) choice group, and append entries
chgEmail = new ChoiceGroup("Email Options", Choice.EXCLUSIVE);
chgEmail.append("Read", null);
chgEmail.append("Forward", null);
chgEmail.append("Delete", null);
// Create the gauge
gauVolume = new Gauge("Sound Level", true, 30, 4);
cmdExit = new Command("Exit", Command.EXIT, 1);
// Create the form
frmMain = new Form("Form Stuff");
frmMain.append(txfSize);
frmMain.append(chgEmail);
frmMain.append(gauVolume);
frmMain.addCommand(cmdExit);
// Capture events
frmMain.setCommandListener(this);
}
// Called by application manager to start the MIDlet.
public void startApp()
{
display.setCurrent(frmMain);
}
public void pauseApp()
{ }
public void destroyApp(boolean unconditional)
{ }
public void commandAction(Command c, Displayable s)
{
if (c == cmdExit)
{
destroyApp(false);
notifyDestroyed();
}
}
}
Related examples in the same category