Form Juggle
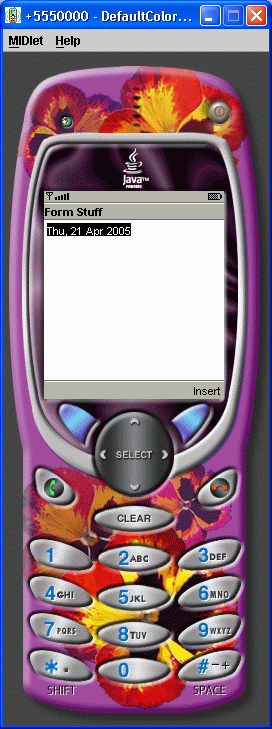
/*--------------------------------------------------
* FormJuggle.java
*
* Show how a Form component handles inserting and
* setting (replacing) Items
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class FormJuggle extends MIDlet implements CommandListener
{
private Display display; // Reference to display object
private Form fmMain; // The main form
private Command cmInsert; // Command to insert items
private DateField dfDate; // Display date
private TextField tfSize; // Product size
private TextField tfQuantity; // Product quantity
private int dateIndex; // Index of dfDate
public FormJuggle()
{
display = Display.getDisplay(this);
// Create the date and populate with current date
dfDate = new DateField("", DateField.DATE);
dfDate.setDate(new java.util.Date());
// Define two textfields and two commands
tfSize = new TextField("Size", "Large", 5, TextField.ANY);
tfQuantity = new TextField("Quantity:", "3", 2, TextField.NUMERIC);
cmInsert = new Command("Insert", Command.SCREEN, 1);
// Create the form, add insert command
fmMain = new Form("Form Stuff");
fmMain.addCommand(cmInsert);
// Append date to form & save index value where it was inserted
dateIndex = fmMain.append(dfDate);
// Capture events
fmMain.setCommandListener(this);
}
// Called by application manager to start the MIDlet.
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{ }
public void destroyApp(boolean unconditional)
{ }
public void commandAction(Command c, Displayable s)
{
if (c == cmInsert)
{
// One item on form, insert textfield prior to datefield
if (fmMain.size() == 1)
{
fmMain.insert(dateIndex, tfQuantity);
dateIndex += 1; // Date index has changed, update it
}
// If two items and last item is datefield, replace it
else if (fmMain.size() == 2 && fmMain.get(1) == dfDate)
fmMain.set(dateIndex, tfSize);
}
}
}
Related examples in the same category