Paragraph Layout
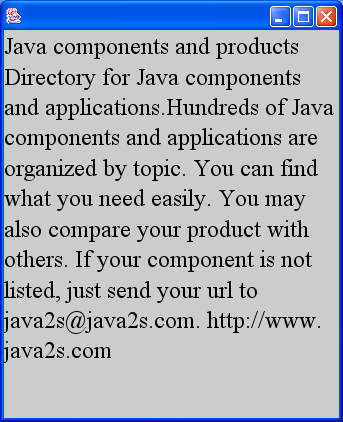
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Insets;
import java.awt.RenderingHints;
import java.awt.font.FontRenderContext;
import java.awt.font.LineBreakMeasurer;
import java.awt.font.TextAttribute;
import java.awt.font.TextLayout;
import java.text.AttributedCharacterIterator;
import java.text.AttributedString;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class ParagraphLayout extends JPanel {
public void paint(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
String s = "Java components and products Directory for Java components " +
"and applications.Hundreds of Java components and applications " +
"are organized by topic. You can find what you need easily. " +
"You may also compare your product with others. If your component " +
"is not listed, just send your url to java2s@java2s.com. " +
"http://www.java2s.com";
Font font = new Font("Serif", Font.PLAIN, 24);
AttributedString as = new AttributedString(s);
as.addAttribute(TextAttribute.FONT, font);
AttributedCharacterIterator aci = as.getIterator();
FontRenderContext frc = g2.getFontRenderContext();
LineBreakMeasurer lbm = new LineBreakMeasurer(aci, frc);
Insets insets = getInsets();
float wrappingWidth = getSize().width - insets.left - insets.right;
float x = insets.left;
float y = insets.top;
while (lbm.getPosition() < aci.getEndIndex()) {
TextLayout textLayout = lbm.nextLayout(wrappingWidth);
y += textLayout.getAscent();
textLayout.draw(g2, x, y);
y += textLayout.getDescent() + textLayout.getLeading();
x = insets.left;
}
}
public static void main(String[] args) {
JFrame f = new JFrame();
f.getContentPane().add(new ParagraphLayout());
f.setSize(350, 250);
f.show();
}
}
Related examples in the same category