LineMetrics: the metrics to layout characters along a line
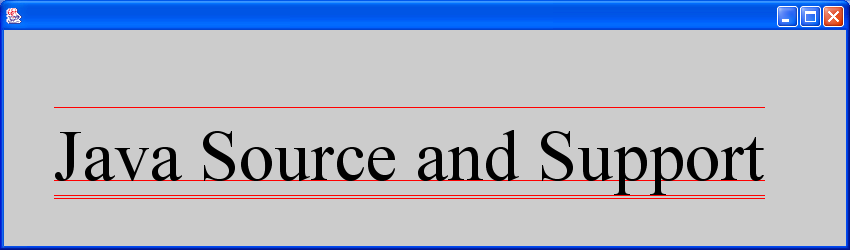
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.font.FontRenderContext;
import java.awt.font.LineMetrics;
import java.awt.geom.Line2D;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class LineMetricsIllustration extends JPanel{
public void paint(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
Font font = new Font("Serif", Font.PLAIN, 72);
g2.setFont(font);
String s = "Java Source and Support";
float x = 50, y = 150;
// Draw the baseline.
FontRenderContext frc = g2.getFontRenderContext();
float width = (float) font.getStringBounds(s, frc).getWidth();
Line2D baseline = new Line2D.Float(x, y, x + width, y);
g2.setPaint(Color.red);
g2.draw(baseline);
// Draw the ascent.
LineMetrics lm = font.getLineMetrics(s, frc);
Line2D ascent = new Line2D.Float(x, y - lm.getAscent(), x + width, y
- lm.getAscent());
g2.draw(ascent);
// Draw the descent.
Line2D descent = new Line2D.Float(x, y + lm.getDescent(), x + width, y
+ lm.getDescent());
g2.draw(descent);
// Draw the leading.
Line2D leading = new Line2D.Float(x, y + lm.getDescent()
+ lm.getLeading(), x + width, y + lm.getDescent()
+ lm.getLeading());
g2.draw(leading);
// Render the string.
g2.setPaint(Color.black);
g2.drawString(s, x, y);
}
public static void main(String[] args) {
JFrame f = new JFrame();
f.getContentPane().add(new LineMetricsIllustration());
f.setSize(850, 250);
f.show();
}
}
Related examples in the same category