Creates a diagonal cross shape.
/*
* JCommon : a free general purpose class library for the Java(tm) platform
*
*
* (C) Copyright 2000-2008, by Object Refinery Limited and Contributors.
*
* Project Info: http://www.jfree.org/jcommon/index.html
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; either version 2.1 of the License, or
* (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301,
* USA.
*
* [Java is a trademark or registered trademark of Sun Microsystems, Inc.
* in the United States and other countries.]
*
* -------------------
* ShapeUtilities.java
* -------------------
* (C)opyright 2003-2008, by Object Refinery Limited and Contributors.
*
* Original Author: David Gilbert (for Object Refinery Limited);
* Contributor(s): -;
*
* $Id: ShapeUtilities.java,v 1.18 2008/06/02 06:58:28 mungady Exp $
*
* Changes
* -------
* 13-Aug-2003 : Version 1 (DG);
* 16-Mar-2004 : Moved rotateShape() from RefineryUtilities.java to here (DG);
* 13-May-2004 : Added new shape creation methods (DG);
* 30-Sep-2004 : Added createLineRegion() method (DG);
* Moved drawRotatedShape() method from RefineryUtilities class
* to this class (DG);
* 04-Oct-2004 : Renamed ShapeUtils --> ShapeUtilities (DG);
* 26-Oct-2004 : Added a method to test the equality of two Line2D
* instances (DG);
* 10-Nov-2004 : Added new translateShape() and equal(Ellipse2D, Ellipse2D)
* methods (DG);
* 11-Nov-2004 : Renamed translateShape() --> createTranslatedShape() (DG);
* 07-Jan-2005 : Minor Javadoc fix (DG);
* 11-Jan-2005 : Removed deprecated code in preparation for 1.0.0 release (DG);
* 21-Jan-2005 : Modified return type of RectangleAnchor.coordinates()
* method (DG);
* 22-Feb-2005 : Added equality tests for Arc2D and GeneralPath (DG);
* 16-Mar-2005 : Fixed bug where equal(Shape, Shape) fails for two Polygon
* instances (DG);
* 01-Jun-2008 : Fixed bug in equal(GeneralPath, GeneralPath) method (DG);
*
*/
import java.awt.Shape;
import java.awt.geom.GeneralPath;
/**
* Utility methods for {@link Shape} objects.
*
* @author David Gilbert
*/
public class Main {
/** A useful constant used internally. */
private static final float SQRT2 = (float) Math.pow(2.0, 0.5);
/**
* Creates a diagonal cross shape.
*
* @param l the length of each 'arm'.
* @param t the thickness.
*
* @return A diagonal cross shape.
*/
public static Shape createDiagonalCross(final float l, final float t) {
final GeneralPath p0 = new GeneralPath();
p0.moveTo(-l - t, -l + t);
p0.lineTo(-l + t, -l - t);
p0.lineTo(0.0f, -t * SQRT2);
p0.lineTo(l - t, -l - t);
p0.lineTo(l + t, -l + t);
p0.lineTo(t * SQRT2, 0.0f);
p0.lineTo(l + t, l - t);
p0.lineTo(l - t, l + t);
p0.lineTo(0.0f, t * SQRT2);
p0.lineTo(-l + t, l + t);
p0.lineTo(-l - t, l - t);
p0.lineTo(-t * SQRT2, 0.0f);
p0.closePath();
return p0;
}
}
Related examples in the same category
1. | Creating Basic Shapes | | |
2. | fillRect (int, int, int, int) method draws a solid rectangle | | |
3. | Creating a Shape Using Lines and Curves | | |
4. | Combining Shapes | | |
5. | Draw rectangles, use the drawRect() method. To fill rectangles, use the fillRect() method | | |
6. | Draw line | | 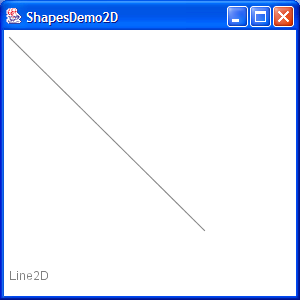 |
7. | Draw a Polygon | | 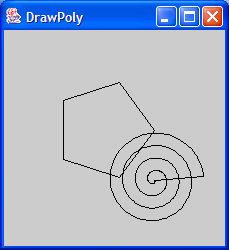 |
8. | Draw an oval outline | | |
9. | Draw a (Round)rectangle | | 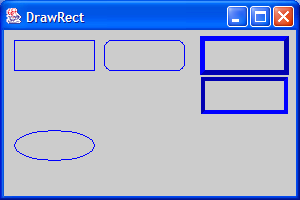 |
10. | Fill a polygon | |  |
11. | Fill a solid oval | | |
12. | Fill a (Round)rectangle | | 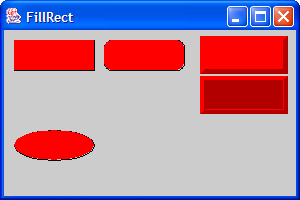 |
13. | Change font | | 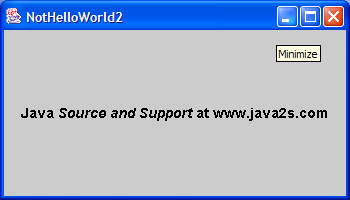 |
14. | Draw rectangle 2 | | 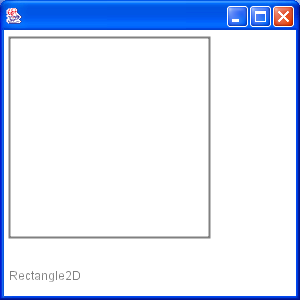 |
15. | Draw Arc | | 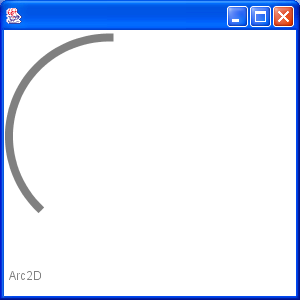 |
16. | Draw Ellipse | | 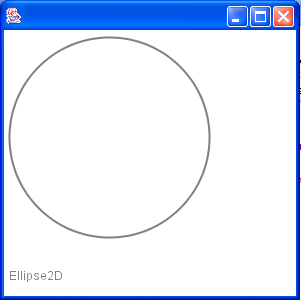 |
17. | Fill a Rectangle 2 | | 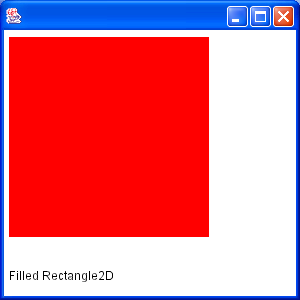 |
18. | Fill Arc 2 | | 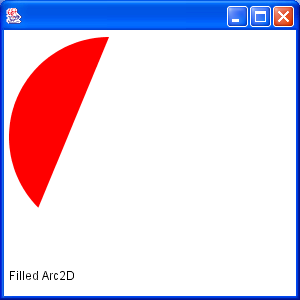 |
19. | Draw text | |  |
20. | Draw unicode string | | 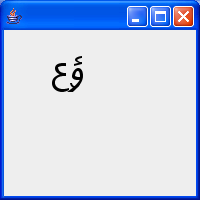 |
21. | Shape combine | | 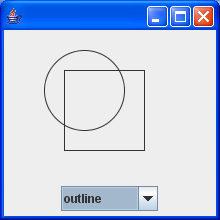 |
22. | Effects | | 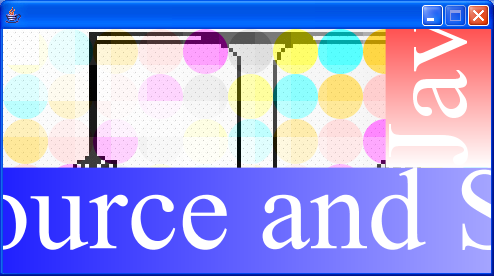 |
23. | Mouse drag and drop to draw | | 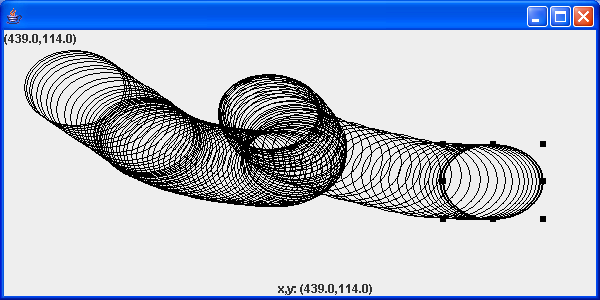 |
24. | Arc demonstration: scale, move, rotate, sheer | | 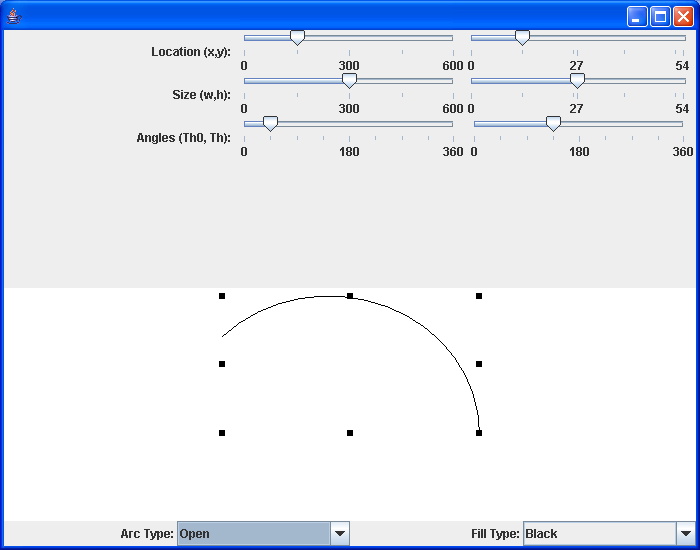 |
25. | Hypnosis Spiral | | 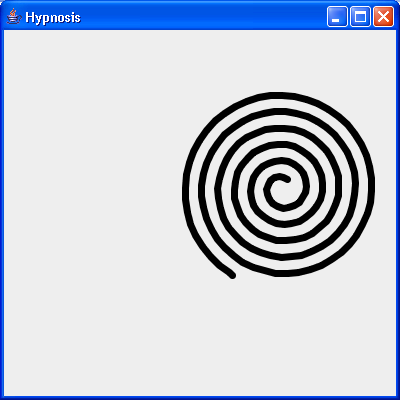 |
26. | GlyphVector.getNumGlyphs() | | |
27. | Resize a shape | | |
28. | Rectangle with rounded corners drawn using Java 2D Graphics API | | |
29. | Compares two ellipses and returns true if they are equal or both null. | | |
30. | Compares two lines are returns true if they are equal or both null. | | |
31. | Creates a diamond shape. | | |
32. | Creates a new Stroke-Object for the given type and with. | | |
33. | Creates a region surrounding a line segment by 'widening' the line segment. | | |
34. | Creates a triangle shape that points downwards. | | |
35. | Creates a triangle shape that points upwards. | | |
36. | Generate Polygon | | |
37. | Polygon with float coordinates. | | |
38. | Polyline 2D | | |
39. | Serialises a Shape object. | | |
40. | Tests two polygons for equality. If both are null this method returns true. | | |
41. | Union two rectangles | | |
42. | Calculate Intersection Clip | | |
43. | Draws a shape with the specified rotation about (x, y). | | |
44. | Checks, whether the given rectangle1 fully contains rectangle 2 (even if rectangle 2 has a height or width of zero!). | | |
45. | Reads a Point2D object that has been serialised by the writePoint2D(Point2D, ObjectOutputStream)} method. | | |
46. | Returns a point based on (x, y) but constrained to be within the bounds of a given rectangle. | | |
47. | RectListManager is a class to manage a list of rectangular regions. | | |
48. | Fill Rectangle2D.Double and Ellipse2D.Double | | 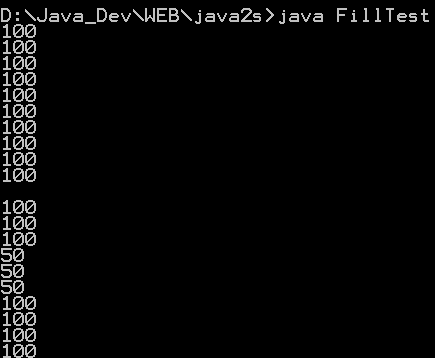 |
49. | This program demonstrates the various 2D shapes | | 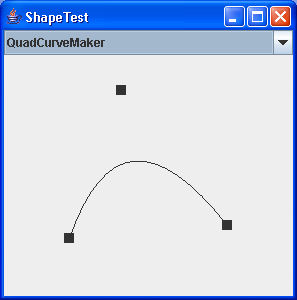 |