Using functions isdigit, isalpha, isalnum, and isxdigit
#include <iostream>
#include <ctype.h>
using namespace std;
int main()
{
cout << "According to isdigit:\n"
<< ( isdigit( '8' ) ? "8 is a" : "8 is not a" )
<< " digit\n"
<< ( isdigit( '#' ) ? "# is a" : "# is not a" )
<< " digit\n";
cout << "\nAccording to isalpha:\n"
<< ( isalpha( 'A' ) ? "A is a" : "A is not a" )
<< " letter\n"
<< ( isalpha( 'b' ) ? "b is a" : "b is not a" )
<< " letter\n"
<< ( isalpha( '&' ) ? "& is a" : "& is not a" )
<< " letter\n"
<< ( isalpha( '4' ) ? "4 is a" : "4 is not a" )
<< " letter\n";
cout << "\nAccording to isalnum:\n"
<< ( isalnum( 'A' ) ? "A is a" : "A is not a" )
<< " digit or a letter\n"
<< ( isalnum( '8' ) ? "8 is a" : "8 is not a" )
<< " digit or a letter\n"
<< ( isalnum( '#' ) ? "# is a" : "# is not a" )
<< " digit or a letter\n";
cout << "\nAccording to isxdigit:\n"
<< ( isxdigit( 'F' ) ? "F is a" : "F is not a" )
<< " hexadecimal digit\n"
<< ( isxdigit( 'J' ) ? "J is a" : "J is not a" )
<< " hexadecimal digit\n"
<< ( isxdigit( '7' ) ? "7 is a" : "7 is not a" )
<< " hexadecimal digit\n"
<< ( isxdigit( '$' ) ? "$ is a" : "$ is not a" )
<< " hexadecimal digit\n"
<< ( isxdigit( 'f' ) ? "f is a" : "f is not a" )
<< " hexadecimal digit" << endl;
return 0;
}
Related examples in the same category
1. | Wrap char pointer to a String class | |  |
2. | Read string and output its length | | 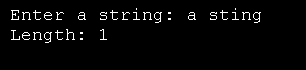 |
3. | Declare a stack class for characters. | | 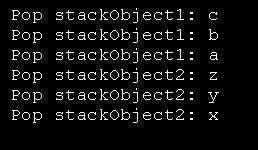 |
4. | Overload string reversal function. | | 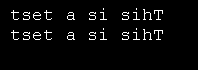 |
5. | Enters a character and outputs its octal, decimal, and hexadecimal code. | | |
6. | cin and cout work with char array | | 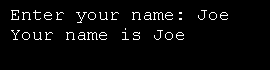 |
7. | Declares str just before it is needed | | 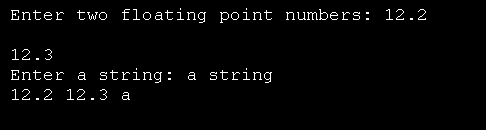 |
8. | Using C strings: cin,=.sync, getline | | 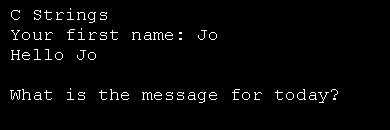 |
9. | Convert a number to a char then convert to upper case and lower case | | |
10. | Using functions islower, isupper, tolower, toupper | | |
11. | Using functions isspace, iscntrl, ispunct, isprint, isgraph | | |