Declare a stack class for characters.
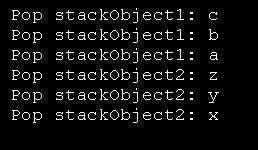
#include <iostream>
using namespace std;
#define SIZE 10
class stack {
char stackData[SIZE]; // holds the stack
int topOfStack; // index of top-of-stack
public:
stack() { topOfStack = 0; }
void push(char ch)
{
if(topOfStack==SIZE) {
cout << "Stack is full\n";
return;
}
stackData[topOfStack] = ch;
topOfStack++;
}
char pop()
{
if(topOfStack==0) {
cout << "Stack is empty\n";
return 0; // return null on empty stack
}
topOfStack--;
return stackData[topOfStack];
}
};
int main()
{
stack stackObject1, stackObject2;
int i;
stackObject1.push('a');
stackObject2.push('x');
stackObject1.push('b');
stackObject2.push('y');
stackObject1.push('c');
stackObject2.push('z');
for(i = 0; i <3; i++)
cout << "Pop stackObject1: " << stackObject1.pop() << endl;
for(i = 0; i <3; i++)
cout << "Pop stackObject2: " << stackObject2.pop() << endl;
return 0;
}
Related examples in the same category
1. | Wrap char pointer to a String class | |  |
2. | Read string and output its length | | 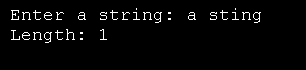 |
3. | Overload string reversal function. | | 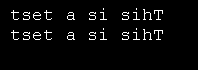 |
4. | Enters a character and outputs its octal, decimal, and hexadecimal code. | | |
5. | cin and cout work with char array | | 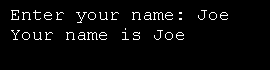 |
6. | Declares str just before it is needed | | 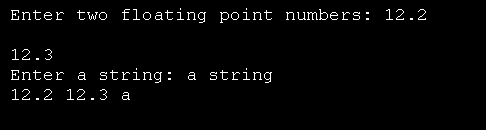 |
7. | Using C strings: cin,=.sync, getline | | 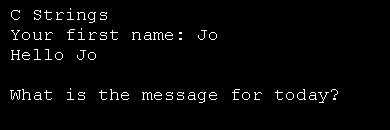 |
8. | Convert a number to a char then convert to upper case and lower case | | |
9. | Using functions islower, isupper, tolower, toupper | | |
10. | Using functions isdigit, isalpha, isalnum, and isxdigit | | |
11. | Using functions isspace, iscntrl, ispunct, isprint, isgraph | | |