Using reverse() to create a palindrome tester.
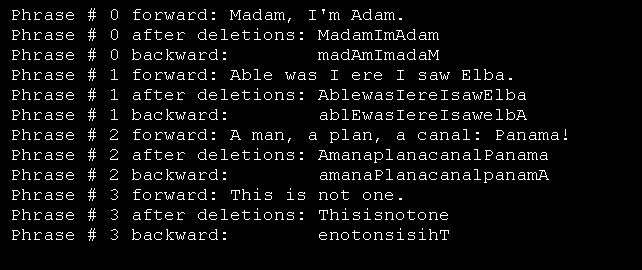
#include <iostream>
#include <list>
using namespace std;
char phrases[][80] = {
"Madam, I'm Adam.",
"Able was I ere I saw Elba.",
"A man, a plan, a canal: Panama!",
"This is not one.",
""
};
int main()
{
list<char> pal;
int i, j;
list<char>::iterator p;
for(i = 0; *phrases[ i ]; i++) {
for(j = 0; phrases[ i ][ j ]; j++)
pal.push_back(phrases[ i ][ j ]);
cout << "Phrase # " << i << " forward: ";
p = pal.begin();
while(p != pal.end()) cout << *p++;
cout << endl;
// remove extraneous characters
pal.remove(',');
pal.remove('.');
pal.remove('!');
pal.remove(':');
pal.remove('\'');
pal.remove(' ');
cout << "Phrase # " << i << " after deletions: ";
p = pal.begin();
while(p != pal.end())
cout << *p++;
cout << endl;
pal.reverse(); // reverse the list
cout << "Phrase # " << i << " backward: ";
p = pal.begin();
while(p != pal.end())
cout << *p++;
cout << endl;
pal.clear(); // get ready to try next phrase
}
return 0;
}
Related examples in the same category