Directory Tree Host
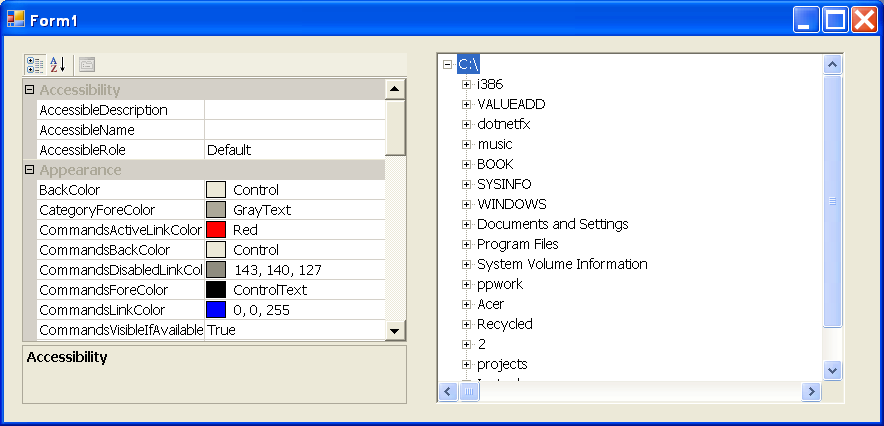
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System.IO;
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace DirectoryTreeHost
{
/// <summary>
/// Summary description for DirectoryTreeHost.
/// </summary>
public class DirectoryTreeHost : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public DirectoryTreeHost()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// DirectoryTreeHost
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Name = "DirectoryTreeHost";
this.Text = "DirectoryTreeHost";
this.Load += new System.EventHandler(this.DirectoryTreeHost_Load);
}
#endregion
private void DirectoryTreeHost_Load(object sender, System.EventArgs e)
{
DirectoryTree dirTree = new
DirectoryTree();
dirTree.Size = new Size(this.Width - 30, this.Height - 60);
dirTree.Location = new Point(5, 5);
dirTree.Drive = Char.Parse("C");
this.Controls.Add(dirTree);
}
public static void Main()
{
Application.Run(new DirectoryTreeHost());
}
}
public class DirectoryTree : TreeView
{
public delegate void DirectorySelectedDelegate(object sender,
DirectorySelectedEventArgs e);
public event DirectorySelectedDelegate DirectorySelected;
private Char drive;
public Char Drive
{
get
{
return drive;
}
set
{
drive = value;
RefreshDisplay();
}
}
// This is public so a Refresh can be triggered manually.
public void RefreshDisplay()
{
// Erase the existing tree.
this.Nodes.Clear();
// Set the first node.
TreeNode rootNode = new TreeNode(drive + ":\\");
this.Nodes.Add(rootNode);
// Fill the first level and expand it.
Fill(rootNode);
this.Nodes[0].Expand();
}
private void Fill(TreeNode dirNode)
{
DirectoryInfo dir = new DirectoryInfo(dirNode.FullPath);
// An exception could be thrown in this code if you don't
// have sufficient security permissions for a file or directory.
// You can catch and then ignore this exception.
foreach (DirectoryInfo dirItem in dir.GetDirectories())
{
// Add node for the directory.
TreeNode newNode = new TreeNode(dirItem.Name);
dirNode.Nodes.Add(newNode);
newNode.Nodes.Add("*");
}
}
protected override void OnBeforeExpand(TreeViewCancelEventArgs e)
{
base.OnBeforeExpand(e);
// If a dummy node is found, remove it and read the real directory list.
if (e.Node.Nodes[0].Text == "*")
{
e.Node.Nodes.Clear();
Fill(e.Node);
}
}
protected override void OnAfterSelect(TreeViewEventArgs e)
{
base.OnAfterSelect(e);
// Raise the DirectorySelected event.
if (DirectorySelected != null)
{
DirectorySelected(this,
new DirectorySelectedEventArgs(e.Node.FullPath));
}
}
}
public class DirectorySelectedEventArgs : EventArgs
{
public string DirectoryName;
public DirectorySelectedEventArgs(string directoryName)
{
this.DirectoryName = directoryName;
}
}
}
Related examples in the same category