illustrates operator overloading
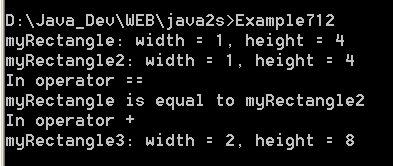
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example7_12.cs illustrates operator overloading
*/
using System;
// declare the Rectangle class
class Rectangle
{
// declare the fields
public int width;
public int height;
// define constructor
public Rectangle(int width, int height)
{
this.width = width;
this.height = height;
}
// override the ToString() method
public override string ToString()
{
return "width = " + width + ", height = " + height;
}
// overload the == operator
public static bool operator ==(Rectangle lhs, Rectangle rhs)
{
Console.WriteLine("In operator ==");
if (lhs.width == rhs.width && lhs.height == rhs.height)
{
return true;
}
else
{
return false;
}
}
// overload the != operator
public static bool operator !=(Rectangle lhs, Rectangle rhs)
{
Console.WriteLine("In operator !=");
return !(lhs==rhs);
}
// override the Equals() method
public override bool Equals(object obj)
{
Console.WriteLine("In Equals()");
if (!(obj is Rectangle))
{
return false;
}
else
{
return this == (Rectangle) obj;
}
}
// overload the + operator
public static Rectangle operator +(Rectangle lhs, Rectangle rhs)
{
Console.WriteLine("In operator +");
return new Rectangle(lhs.width + rhs.width, lhs.height + rhs.height);
}
}
public class Example7_12
{
public static void Main()
{
// create Rectangle objects
Rectangle myRectangle = new Rectangle(1, 4);
Console.WriteLine("myRectangle: " + myRectangle);
Rectangle myRectangle2 = new Rectangle(1, 4);
Console.WriteLine("myRectangle2: " + myRectangle2);
if (myRectangle == myRectangle2)
{
Console.WriteLine("myRectangle is equal to myRectangle2");
}
else
{
Console.WriteLine("myRectangle is not equal to myRectangle2");
}
Rectangle myRectangle3 = myRectangle + myRectangle2;
Console.WriteLine("myRectangle3: " + myRectangle3);
}
}
Related examples in the same category