Demonstrates overloading the addition operator for two class objects
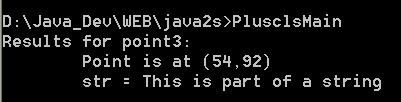
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
//
// Plus.cs -- demonstrates overloading the addition operator for two
// class objects.
//
// Compile this program with the following command line:
// C:>csc Plus.cs
//
namespace nsOverload
{
using System;
public class PlusclsMain
{
static public void Main ()
{
clsPoint point1 = new clsPoint (12, 28, "This is part");
clsPoint point2 = new clsPoint (42, 64, " of a string");
clsPoint point3 = point1 + point2;
Console.WriteLine ("Results for point3:");
Console.WriteLine ("\tPoint is at " + point3);
Console.WriteLine ("\tstr = " + point3.str);
}
}
class clsPoint
{
public clsPoint () { }
public clsPoint (int x, int y, string str)
{
m_cx = x;
m_cy = y;
this.str = str;
}
private int m_cx = 0;
private int m_cy = 0;
public int cx
{
get {return (m_cx);}
set {m_cx = value;}
}
public int cy
{
get {return (m_cy);}
set {m_cy = value;}
}
public string str = "";
static public clsPoint operator +(clsPoint pt1, clsPoint pt2)
{
clsPoint point = new clsPoint();
point.cx = pt1.cx + pt2.cx;
point.cy = pt1.cy + pt2.cy;
point.str = pt1.str + pt2.str;
return (point);
}
public override string ToString()
{
return ("(" + m_cx + "," + m_cy + ")");
}
}
}
Related examples in the same category