Format data in ToString method.
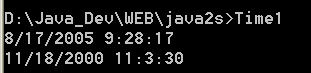
using System;
public class Time1
{
private int hour;
private int minute;
private int second;
public void SetTime( int h, int m, int s )
{
hour = ( ( h >= 0 && h < 24 ) ? h : 0 );
minute = ( ( m >= 0 && m < 60 ) ? m : 0 );
second = ( ( s >= 0 && s < 60 ) ? s : 0 );
}
public string ToUniversalString()
{
return string.Format( "{0:D2}:{1:D2}:{2:D2}", hour, minute, second );
}
public override string ToString()
{
return string.Format( "{0:D2}:{1:D2}:{2:D2} {3}",
( ( hour == 0 || hour == 12 ) ? 12 : hour % 12 ),
minute, second, ( hour < 12 ? "AM" : "PM" ) );
}
}
public class MemberAccessTest
{
public static void Main( string[] args )
{
Time1 time = new Time1();
time.hour = 7; // error: hour has private access in Time1
time.minute = 15; // error: minute has private access in Time1
time.second = 30; // error: second has private access in Time1
}
}
Related examples in the same category