Display a Dialog Window
Description
To display a dialog window to get a confirmation from the user,
you can override the onCreateDialog()
protected method
defined in the Activity
base
class to display a dialog window.
Example
Java code
package com.java2s.app;
//from w w w . j av a2 s . c o m
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.widget.Toast;
public class MainActivity extends Activity {
CharSequence[] items = {"XML", "CSS", "HTML"};
boolean[] itemsChecked = new boolean[items.length];
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
new AlertDialog.Builder(this)
.setIcon(R.drawable.ic_launcher)
.setTitle("Title text...")
.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
Toast.makeText(getBaseContext(),
"OK clicked!", Toast.LENGTH_SHORT).show();
}
}
).setNegativeButton("Cancel",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
Toast.makeText(getBaseContext(),
"Cancel clicked!", Toast.LENGTH_SHORT).show();
}
}
).setMultiChoiceItems(items, itemsChecked,
new DialogInterface.OnMultiChoiceClickListener() {
public void onClick(DialogInterface dialog,
int which, boolean isChecked) {
Toast.makeText(getBaseContext(),
items[which] + (isChecked ? " checked!" : " unchecked!"),
Toast.LENGTH_SHORT).show();
}
}
).create().show();
}
}
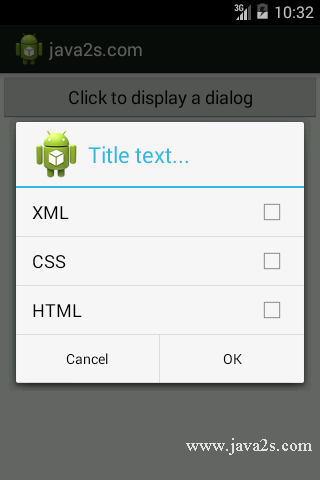