Add CheckBox to AlertDialog
Description
We can set a list of checkboxes for users to choose via
the setMultiChoiceItems()
method.
For setMultiChoiceItems()
method, you passed in two arrays:
- one for the list of items to display
- another to contain the value of each item, to indicate if they are checked.
Example
When an item is checked, you use the Toast class to display a message indicating the item that was checked.
The following code has the layout.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
/*from w w w.jav a2 s . co m*/
<Button
android:id="@+id/btn_dialog"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Click to display a dialog"
android:onClick="onClick" />
</LinearLayout>
Java code
package com.java2s.app;
//from w w w. ja v a2s.co m
import android.app.Activity;
import android.app.AlertDialog;
import android.app.AlertDialog.Builder;
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
public class MainActivity extends Activity {
CharSequence[] items = {"XML", "Java", "CSS"};
boolean[] itemsChecked = new boolean[items.length];
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void onClick(View v) {
Builder builder = new AlertDialog.Builder(this);
builder.setIcon(R.drawable.ic_launcher);
builder.setTitle("This is a dialog with some simple text...");
builder.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
Toast.makeText(getBaseContext(),
"OK clicked!", Toast.LENGTH_SHORT).show();
}
}
);
builder.setNegativeButton("Cancel",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
Toast.makeText(getBaseContext(),
"Cancel clicked!", Toast.LENGTH_SHORT).show();
}
}
);
builder.setMultiChoiceItems(items, itemsChecked,
new DialogInterface.OnMultiChoiceClickListener() {
public void onClick(DialogInterface dialog,
int which, boolean isChecked) {
Toast.makeText(getBaseContext(),
items[which] + (isChecked ? " checked!" : " unchecked!"),
Toast.LENGTH_SHORT).show();
}
}
);
builder.create().show();
}
}
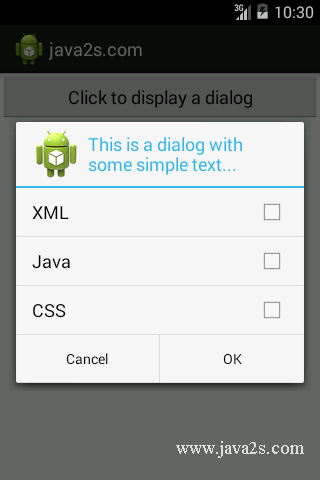