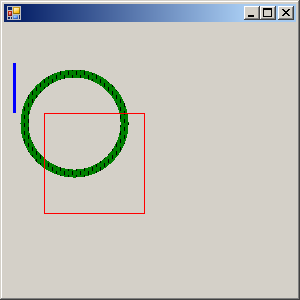
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class CreatePenFromHatchBrush
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
public class Form1
Inherits System.Windows.Forms.Form
Protected Overrides Sub OnPaint(ByVal e As PaintEventArgs)
Dim g As Graphics = Me.CreateGraphics()
g.Clear(Me.BackColor)
' Create a solid and hatch brush
Dim blueBrush As New SolidBrush(Color.Blue)
Dim hatchBrush As New HatchBrush(HatchStyle.DashedVertical, Color.Black, Color.Green)
' Create a pen from a solid brush with
' width 3
Dim pn1 As New Pen(blueBrush, 3)
' Create a pen from a hatch brush
Dim pn2 As New Pen(hatchBrush, 8)
' Create a pen from a Color structure
Dim pn3 As New Pen(Color.Red)
' Draw a line, ellipse, and rectangle
g.DrawLine(pn1, New Point(10, 40), New Point(10, 90))
g.DrawEllipse(pn2, 20, 50, 100, 100)
g.DrawRectangle(pn3, 40, 90, 100, 100)
' Dispose
pn1.Dispose()
pn2.Dispose()
pn3.Dispose()
blueBrush.Dispose()
hatchBrush.Dispose()
g.Dispose()
End Sub
Public Sub New()
MyBase.New()
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen
End Sub
End Class