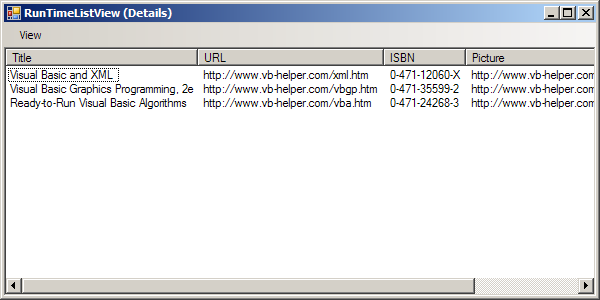
'Visual Basic 2005 Programmer's Reference
'by Rod Stephens (Author)
'# Publisher: Wrox (October 21, 2005)
'# Language: English
'# ISBN-10: 0764571982
'# ISBN-13: 978-0764571985
Imports System.Windows.Forms
public class ListViewRunTime
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
' Make the ListView column headers.
ListViewMakeColumnHeaders(lvwBooks, _
"Title", HorizontalAlignment.Left, 120, _
"URL", HorizontalAlignment.Left, 120, _
"ISBN", HorizontalAlignment.Left, 90, _
"Picture", HorizontalAlignment.Left, 120, _
"Pages", HorizontalAlignment.Right, 50, _
"Year", HorizontalAlignment.Right, 40)
ListViewMakeRow(lvwBooks, 0, _
"Visual Basic and XML", _
"http://www.vb-helper.com/xml.htm", _
"0-471-12060-X", _
"http://www.vb-helper.com/xml.jpg", _
"503", _
"2002")
ListViewMakeRow(lvwBooks, 0, _
"Visual Basic Graphics Programming, 2e", _
"http://www.vb-helper.com/vbgp.htm", _
"0-471-35599-2", _
"http://www.vb-helper.com/vbgp.jpg", _
"712", _
"2000")
ListViewMakeRow(lvwBooks, 0, _
"Ready-to-Run Visual Basic Algorithms", _
"http://www.vb-helper.com/vba.htm", _
"0-471-24268-3", _
"http://www.vb-helper.com/vba.jpg", _
"395", _
"1998")
ListViewSizeColumns(lvwBooks, True)
Text = "RunTimeListView (" & lvwBooks.View.ToString & ")"
lvwBooks.View = View.Details
CheckMenus()
End Sub
' Make the ListView's column headers.
' The ParamArray entries should be triples holding
' column title, HorizontalAlignment value, and width.
Private Sub ListViewMakeColumnHeaders(ByVal lvw As ListView, ByVal ParamArray header_info() As Object)
' Remove any existing headers.
lvw.Columns.Clear()
' Make the column headers.
For i As Integer = header_info.GetLowerBound(0) To header_info.GetUpperBound(0) Step 3
Dim col_header As ColumnHeader = lvw.Columns.Add( _
DirectCast(header_info(i), String), _
-1, _
DirectCast(header_info(i + 1), HorizontalAlignment))
col_header.Width = DirectCast(header_info(i + 2), Integer)
Next i
End Sub
' Make a ListView row.
Private Sub ListViewMakeRow(ByVal lvw As ListView, ByVal image_index As Integer, ByVal item_title As String, ByVal ParamArray subitem_titles() As String)
' Make the item.
Dim new_item As ListViewItem = lvw.Items.Add(item_title)
new_item.ImageIndex = image_index
' Make the sub-items.
For i As Integer = subitem_titles.GetLowerBound(0) To subitem_titles.GetUpperBound(0)
new_item.SubItems.Add(subitem_titles(i))
Next i
End Sub
' Set column widths to -1 to fit data,
' -2 to fit data and header.
Private Sub ListViewSizeColumns(ByVal lvw As ListView, ByVal allow_room_for_header As Boolean)
Dim new_wid As Integer = -1
If allow_room_for_header Then new_wid = -2
' Set the width for each column.
For i As Integer = 0 To lvw.Columns.Count - 1
lvw.Columns(i).Width = new_wid
Next i
End Sub
Private Sub mnuViewDetails_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuViewDetails.Click
lvwBooks.View = View.Details
Text = "RunTimeListView (" & lvwBooks.View.ToString & ")"
CheckMenus()
End Sub
Private Sub mnuViewLargeIcons_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuViewLargeIcons.Click
lvwBooks.View = View.LargeIcon
Text = "RunTimeListView (" & lvwBooks.View.ToString & ")"
CheckMenus()
End Sub
Private Sub mnuViewList_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuViewList.Click
lvwBooks.View = View.List
Text = "RunTimeListView (" & lvwBooks.View.ToString & ")"
CheckMenus()
End Sub
Private Sub mnuViewSmallIcons_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuViewSmallIcons.Click
lvwBooks.View = View.SmallIcon
Text = "RunTimeListView (" & lvwBooks.View.ToString & ")"
CheckMenus()
End Sub
Private Sub mnuViewTile_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuViewTile.Click
lvwBooks.View = View.Tile
Text = "RunTimeListView (" & lvwBooks.View.ToString & ")"
CheckMenus()
End Sub
Private Sub CheckMenus()
mnuViewDetails.Checked = (lvwBooks.View = View.Details)
mnuViewLargeIcons.Checked = (lvwBooks.View = View.LargeIcon)
mnuViewList.Checked = (lvwBooks.View = View.List)
mnuViewSmallIcons.Checked = (lvwBooks.View = View.SmallIcon)
mnuViewTile.Checked = (lvwBooks.View = View.Tile)
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.components = New System.ComponentModel.Container
Me.MenuStrip1 = New System.Windows.Forms.MenuStrip
Me.ViewToolStripMenuItem = New System.Windows.Forms.ToolStripMenuItem
Me.mnuViewDetails = New System.Windows.Forms.ToolStripMenuItem
Me.mnuViewLargeIcons = New System.Windows.Forms.ToolStripMenuItem
Me.mnuViewList = New System.Windows.Forms.ToolStripMenuItem
Me.mnuViewSmallIcons = New System.Windows.Forms.ToolStripMenuItem
Me.mnuViewTile = New System.Windows.Forms.ToolStripMenuItem
Me.lvwBooks = New System.Windows.Forms.ListView
Me.MenuStrip1.SuspendLayout()
Me.SuspendLayout()
'
'MenuStrip1
'
Me.MenuStrip1.Items.AddRange(New System.Windows.Forms.ToolStripItem() {Me.ViewToolStripMenuItem})
Me.MenuStrip1.Location = New System.Drawing.Point(0, 0)
Me.MenuStrip1.Name = "MenuStrip1"
Me.MenuStrip1.Size = New System.Drawing.Size(592, 24)
Me.MenuStrip1.TabIndex = 2
Me.MenuStrip1.Text = "MenuStrip1"
'
'ViewToolStripMenuItem
'
Me.ViewToolStripMenuItem.DropDownItems.AddRange(New System.Windows.Forms.ToolStripItem() {Me.mnuViewDetails, Me.mnuViewLargeIcons, Me.mnuViewList, Me.mnuViewSmallIcons, Me.mnuViewTile})
Me.ViewToolStripMenuItem.Name = "ViewToolStripMenuItem"
Me.ViewToolStripMenuItem.Text = "&View"
'
'mnuViewDetails
'
Me.mnuViewDetails.Name = "mnuViewDetails"
Me.mnuViewDetails.Text = "&Details"
'
'mnuViewLargeIcons
'
Me.mnuViewLargeIcons.Name = "mnuViewLargeIcons"
Me.mnuViewLargeIcons.Text = "Large Icons"
'
'mnuViewList
'
Me.mnuViewList.Name = "mnuViewList"
Me.mnuViewList.Text = "&List"
'
'mnuViewSmallIcons
'
Me.mnuViewSmallIcons.Name = "mnuViewSmallIcons"
Me.mnuViewSmallIcons.Text = "&Small Icons"
'
'mnuViewTile
'
Me.mnuViewTile.Name = "mnuViewTile"
Me.mnuViewTile.Text = "Tile"
'
'lvwBooks
'
Me.lvwBooks.Dock = System.Windows.Forms.DockStyle.Fill
Me.lvwBooks.Location = New System.Drawing.Point(0, 24)
Me.lvwBooks.Name = "lvwBooks"
Me.lvwBooks.Size = New System.Drawing.Size(592, 249)
Me.lvwBooks.TabIndex = 1
Me.lvwBooks.View = System.Windows.Forms.View.Details
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(592, 273)
Me.Controls.Add(Me.lvwBooks)
Me.Controls.Add(Me.MenuStrip1)
Me.Name = "Form1"
Me.Text = "RunTimeListView"
Me.MenuStrip1.ResumeLayout(False)
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents MenuStrip1 As System.Windows.Forms.MenuStrip
Friend WithEvents ViewToolStripMenuItem As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents mnuViewDetails As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents mnuViewLargeIcons As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents mnuViewList As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents mnuViewSmallIcons As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents mnuViewTile As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents lvwBooks As System.Windows.Forms.ListView
End Class