/*
* Copyright (c) 1995 - 2008 Sun Microsystems, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* - Neither the name of Sun Microsystems nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS
* IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/*
* SimpleTableSelectionDemo.java requires no other files.
*/
import java.awt.Dimension;
import java.awt.GridLayout;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.ListSelectionModel;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
/**
* SimpleTableSelectionDemo is just like SimpleTableDemo, except that it detects
* selections, printing information about the current selection to standard
* output.
*/
public class SimpleTableSelectionDemo extends JPanel {
private boolean DEBUG = false;
private boolean ALLOW_COLUMN_SELECTION = false;
private boolean ALLOW_ROW_SELECTION = true;
public SimpleTableSelectionDemo() {
super(new GridLayout(1, 0));
final String[] columnNames = { "First Name", "Last Name", "Sport",
"# of Years", "Vegetarian" };
final Object[][] data = {
{ "Mary", "Campione", "Snowboarding", new Integer(5),
new Boolean(false) },
{ "Alison", "Huml", "Rowing", new Integer(3), new Boolean(true) },
{ "Kathy", "Walrath", "Knitting", new Integer(2), new Boolean(false) },
{ "Sharon", "Zakhour", "Speed reading", new Integer(20),
new Boolean(true) },
{ "Philip", "Milne", "Pool", new Integer(10), new Boolean(false) } };
final JTable table = new JTable(data, columnNames);
table.setPreferredScrollableViewportSize(new Dimension(500, 70));
table.setFillsViewportHeight(true);
table.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
if (ALLOW_ROW_SELECTION) { // true by default
ListSelectionModel rowSM = table.getSelectionModel();
rowSM.addListSelectionListener(new ListSelectionListener() {
public void valueChanged(ListSelectionEvent e) {
// Ignore extra messages.
if (e.getValueIsAdjusting())
return;
ListSelectionModel lsm = (ListSelectionModel) e.getSource();
if (lsm.isSelectionEmpty()) {
System.out.println("No rows are selected.");
} else {
int selectedRow = lsm.getMinSelectionIndex();
System.out.println("Row " + selectedRow + " is now selected.");
}
}
});
} else {
table.setRowSelectionAllowed(false);
}
if (ALLOW_COLUMN_SELECTION) { // false by default
if (ALLOW_ROW_SELECTION) {
// We allow both row and column selection, which
// implies that we *really* want to allow individual
// cell selection.
table.setCellSelectionEnabled(true);
}
table.setColumnSelectionAllowed(true);
ListSelectionModel colSM = table.getColumnModel().getSelectionModel();
colSM.addListSelectionListener(new ListSelectionListener() {
public void valueChanged(ListSelectionEvent e) {
// Ignore extra messages.
if (e.getValueIsAdjusting())
return;
ListSelectionModel lsm = (ListSelectionModel) e.getSource();
if (lsm.isSelectionEmpty()) {
System.out.println("No columns are selected.");
} else {
int selectedCol = lsm.getMinSelectionIndex();
System.out.println("Column " + selectedCol + " is now selected.");
}
}
});
}
if (DEBUG) {
table.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
printDebugData(table);
}
});
}
// Create the scroll pane and add the table to it.
JScrollPane scrollPane = new JScrollPane(table);
// Add the scroll pane to this panel.
add(scrollPane);
}
private void printDebugData(JTable table) {
int numRows = table.getRowCount();
int numCols = table.getColumnCount();
javax.swing.table.TableModel model = table.getModel();
System.out.println("Value of data: ");
for (int i = 0; i < numRows; i++) {
System.out.print(" row " + i + ":");
for (int j = 0; j < numCols; j++) {
System.out.print(" " + model.getValueAt(i, j));
}
System.out.println();
}
System.out.println("--------------------------");
}
/**
* Create the GUI and show it. For thread safety, this method should be
* invoked from the event-dispatching thread.
*/
private static void createAndShowGUI() {
// Create and set up the window.
JFrame frame = new JFrame("SimpleTableSelectionDemo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create and set up the content pane.
SimpleTableSelectionDemo newContentPane = new SimpleTableSelectionDemo();
newContentPane.setOpaque(true); // content panes must be opaque
frame.setContentPane(newContentPane);
// Display the window.
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
// Schedule a job for the event-dispatching thread:
// creating and showing this application's GUI.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
14.58.JTable |
| 14.58.1. | Creating a JTable | 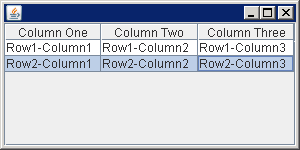 |
| 14.58.2. | Creating a JTable with rows of variable height |
| 14.58.3. | Creating a Scrollable JTable Component |
| 14.58.4. | public JTable(Vector rowData, Vector columnNames) | 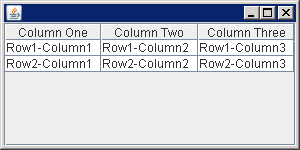 |
| 14.58.5. | Retrieve the value in the visible cell (1,2) in a JTable |
| 14.58.6. | Retrieve the value in cell (1,2) from the model |
| 14.58.7. | Build a table from list data and column names |
| 14.58.8. | To change cell contents in code: setValueAt(Object value, int row, int column) method of JTable. | 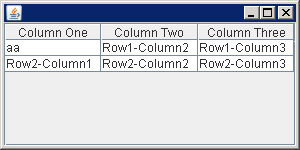 |
| 14.58.9. | Disable auto resizing to make the table horizontal scrollable |
| 14.58.10. | Manually Positioning the JTable View | 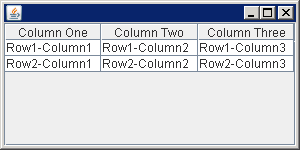 |
| 14.58.11. | Selection Modes | 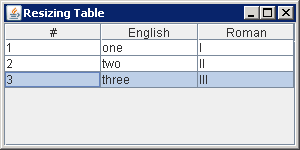 |
| 14.58.12. | Printing Tables Sample | 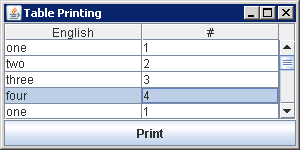 |
| 14.58.13. | Specify the print mode: public boolean print(JTable.PrintMode printMode) | 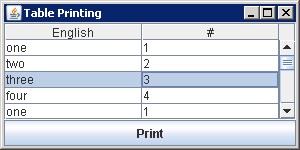 |
| 14.58.14. | Specify a page header or footer during printing | 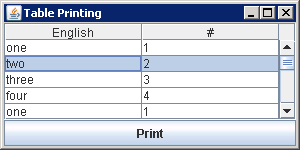 |
| 14.58.15. | No user interaction to print | 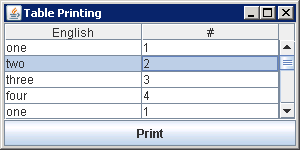 |
| 14.58.16. | Listening to JTable Events with a TableModelListener | 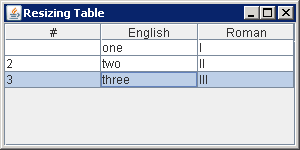 |
| 14.58.17. | Control the selection of rows or columns or individual cells | 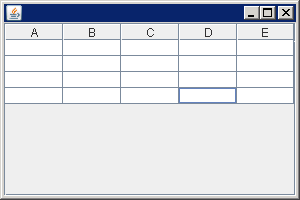 |
| 14.58.18. | Table selection mode |
| 14.58.19. | JTable with Tooltip |
| 14.58.20. | Table Selection Events and Listeners | 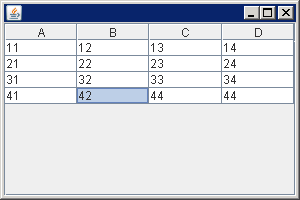 |
| 14.58.21. | Print a JTable out |
| 14.58.22. | JTable Look and Feel |
| 14.58.23. | Listening for Selection Events in a JTable Component |
| 14.58.24. | Listening for Changes to the Rows and Columns of a JTable Component |
| 14.58.25. | Listening for Column-Related Changes in a JTable Component |
| 14.58.26. | Programmatically Starting Cell Editing in a JTable Component |
| 14.58.27. | Select a column - column 0 in a JTable |
| 14.58.28. | Select an additional range of columns - columns 1 to 2 |
| 14.58.29. | Deselect a range of columns - columns 0 to 1 |
| 14.58.30. | Select a row - row 0 |
| 14.58.31. | Select an additional range of rows - rows 1 to 2 |
| 14.58.32. | Deselect a range of rows - rows 0 to 1 |
| 14.58.33. | Select a cell: cell (2,1) |
| 14.58.34. | Select all cells |
| 14.58.35. | Deselect all cells |
| 14.58.36. | Enable row selection (default) in a JTable |
| 14.58.37. | Enable column selection in a JTable |
| 14.58.38. | Enable cell selection in a JTable |
| 14.58.39. | When the width of a column is changed, the width of the right-most column is changed |
| 14.58.40. | When the width of a column is changed, all columns to the right are resized |
| 14.58.41. | When the width of a column is changed, only the columns to the left and right of the margin change |
| 14.58.42. | When the width of a column is changed, the widths of all columns are changed |
| 14.58.43. | Use a regexFilter to filter table content |
| 14.58.44. | Don't show any grid lines |
| 14.58.45. | Show only vertical grid lines |
| 14.58.46. | Show only horizontal grid lines |
| 14.58.47. | Set the grid color |
| 14.58.48. | Show both horizontal and vertical grid lines (the default) |
| 14.58.49. | Getting the Gap Size Between Cells in a JTable Component |
| 14.58.50. | Add 5 spaces to the left and right sides of a cell. |
| 14.58.51. | Scrolling a Cell to the Center of a JTable Component |
| 14.58.52. | Setting Tool Tips on Cells in a JTable Component |
| 14.58.53. | Getting the Number of Rows and Columns in a JTable Component |
| 14.58.54. | Making a Cell Visible in a JTable Component |
| 14.58.55. | Increase the row height |
| 14.58.56. | Determining If a Cell Is Visible in a JTable Component |
| 14.58.57. | Allowing the User to Resize a Column in a JTable Component |
| 14.58.58. | Disabling User Edits in a JTable |
| 14.58.59. | Creating image out of a JTable |