Show the printer dialog box and configure what the default print request attribute set entails,
such as how many copies to print.
public boolean print(JTable.PrintMode printMode, MessageFormat headerFormat,MessageFormat footerFormat, boolean showPrintDialog,PrintRequestAttributeSet attr, boolean interactive)
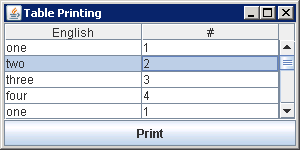
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.print.PrinterException;
import java.text.MessageFormat;
import javax.print.attribute.HashPrintRequestAttributeSet;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
public class TablePrintLastVersion {
public static void main(String args[]) {
final Object rows[][] = {
{"one", "1"},
{"two", "2"},
{"three", "3"},
{"four", "4"},
{"one", "1"},
{"two", "2"},
{"three", "3"},
{"four", "4"},
{"one", "1"},
{"two", "2"},
{"three", "3"},
{"four", "4"},
{"one", "1"},
{"two", "2"},
{"three", "3"},
{"four", "4"},
};
final Object headers[] = {"English", "#"};
JFrame frame = new JFrame("Table Printing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
final JTable table = new JTable(rows, headers);
JScrollPane scrollPane = new JScrollPane(table);
frame.add(scrollPane, BorderLayout.CENTER);
JButton button = new JButton("Print");
ActionListener printAction = new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
MessageFormat headerFormat = new MessageFormat("Page {0}");
MessageFormat footerFormat = new MessageFormat("- {0} -");
table.print(JTable.PrintMode.NORMAL, headerFormat,footerFormat, true, new HashPrintRequestAttributeSet(), true);
} catch (PrinterException pe) {
System.err.println("Error printing: " + pe.getMessage());
}
}
};
button.addActionListener(printAction);
frame.add(button, BorderLayout.SOUTH);
frame.setSize(300, 150);
frame.setVisible(true);
}
}
14.58.JTable |
| 14.58.1. | Creating a JTable | 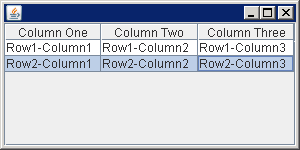 |
| 14.58.2. | Creating a JTable with rows of variable height |
| 14.58.3. | Creating a Scrollable JTable Component |
| 14.58.4. | public JTable(Vector rowData, Vector columnNames) | 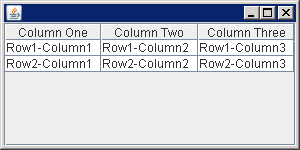 |
| 14.58.5. | Retrieve the value in the visible cell (1,2) in a JTable |
| 14.58.6. | Retrieve the value in cell (1,2) from the model |
| 14.58.7. | Build a table from list data and column names |
| 14.58.8. | To change cell contents in code: setValueAt(Object value, int row, int column) method of JTable. | 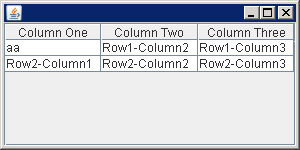 |
| 14.58.9. | Disable auto resizing to make the table horizontal scrollable |
| 14.58.10. | Manually Positioning the JTable View | 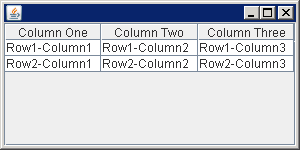 |
| 14.58.11. | Selection Modes | 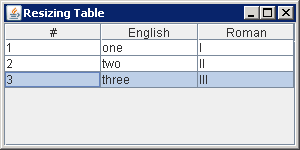 |
| 14.58.12. | Printing Tables Sample | 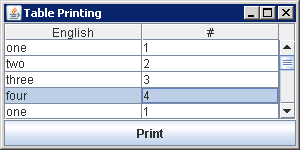 |
| 14.58.13. | Specify the print mode: public boolean print(JTable.PrintMode printMode) | 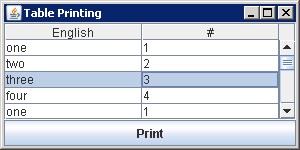 |
| 14.58.14. | Specify a page header or footer during printing | 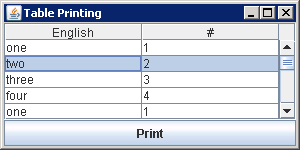 |
| 14.58.15. | No user interaction to print | 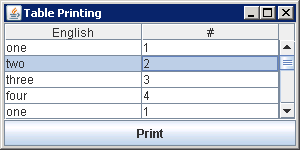 |
| 14.58.16. | Listening to JTable Events with a TableModelListener | 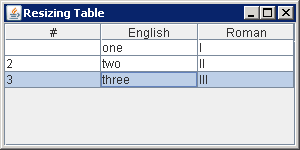 |
| 14.58.17. | Control the selection of rows or columns or individual cells | 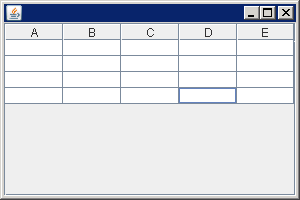 |
| 14.58.18. | Table selection mode |
| 14.58.19. | JTable with Tooltip |
| 14.58.20. | Table Selection Events and Listeners | 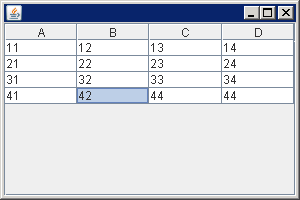 |
| 14.58.21. | Print a JTable out |
| 14.58.22. | JTable Look and Feel |
| 14.58.23. | Listening for Selection Events in a JTable Component |
| 14.58.24. | Listening for Changes to the Rows and Columns of a JTable Component |
| 14.58.25. | Listening for Column-Related Changes in a JTable Component |
| 14.58.26. | Programmatically Starting Cell Editing in a JTable Component |
| 14.58.27. | Select a column - column 0 in a JTable |
| 14.58.28. | Select an additional range of columns - columns 1 to 2 |
| 14.58.29. | Deselect a range of columns - columns 0 to 1 |
| 14.58.30. | Select a row - row 0 |
| 14.58.31. | Select an additional range of rows - rows 1 to 2 |
| 14.58.32. | Deselect a range of rows - rows 0 to 1 |
| 14.58.33. | Select a cell: cell (2,1) |
| 14.58.34. | Select all cells |
| 14.58.35. | Deselect all cells |
| 14.58.36. | Enable row selection (default) in a JTable |
| 14.58.37. | Enable column selection in a JTable |
| 14.58.38. | Enable cell selection in a JTable |
| 14.58.39. | When the width of a column is changed, the width of the right-most column is changed |
| 14.58.40. | When the width of a column is changed, all columns to the right are resized |
| 14.58.41. | When the width of a column is changed, only the columns to the left and right of the margin change |
| 14.58.42. | When the width of a column is changed, the widths of all columns are changed |
| 14.58.43. | Use a regexFilter to filter table content |
| 14.58.44. | Don't show any grid lines |
| 14.58.45. | Show only vertical grid lines |
| 14.58.46. | Show only horizontal grid lines |
| 14.58.47. | Set the grid color |
| 14.58.48. | Show both horizontal and vertical grid lines (the default) |
| 14.58.49. | Getting the Gap Size Between Cells in a JTable Component |
| 14.58.50. | Add 5 spaces to the left and right sides of a cell. |
| 14.58.51. | Scrolling a Cell to the Center of a JTable Component |
| 14.58.52. | Setting Tool Tips on Cells in a JTable Component |
| 14.58.53. | Getting the Number of Rows and Columns in a JTable Component |
| 14.58.54. | Making a Cell Visible in a JTable Component |
| 14.58.55. | Increase the row height |
| 14.58.56. | Determining If a Cell Is Visible in a JTable Component |
| 14.58.57. | Allowing the User to Resize a Column in a JTable Component |
| 14.58.58. | Disabling User Edits in a JTable |
| 14.58.59. | Creating image out of a JTable |