<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:src="clr-namespace:MyNameSpace.CustomElementBinding"
Title="Custom Element Binding Demo">
<StackPanel>
<ScrollBar Name="scroll"
Orientation="Horizontal"
Margin="24"
Maximum="100"
LargeChange="10"
SmallChange="1"
Value="{Binding ElementName=simple, Path=Number,Mode=TwoWay}" />
<src:SimpleElement Number="{Binding ElementName=scroll,Path=Value,Mode=OneWay}"/>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Globalization;
using System.Windows;
using System.Windows.Media;
namespace MyNameSpace.CustomElementBinding
{
class SimpleElement : FrameworkElement
{
public static DependencyProperty NumberProperty;
static SimpleElement()
{
NumberProperty = DependencyProperty.Register("Number", typeof(double),typeof(SimpleElement),
new FrameworkPropertyMetadata(0.0,FrameworkPropertyMetadataOptions.AffectsRender));
}
public double Number
{
set { SetValue(NumberProperty, value); }
get { return (double)GetValue(NumberProperty); }
}
protected override Size MeasureOverride(Size sizeAvailable)
{
return new Size(200, 250);
}
protected override void OnRender(DrawingContext dc)
{
dc.DrawText(new FormattedText(Number.ToString(),
CultureInfo.CurrentCulture, FlowDirection.LeftToRight,
new Typeface("Times New Roman"), 12,
SystemColors.WindowTextBrush),new Point(0, 0));
}
}
}
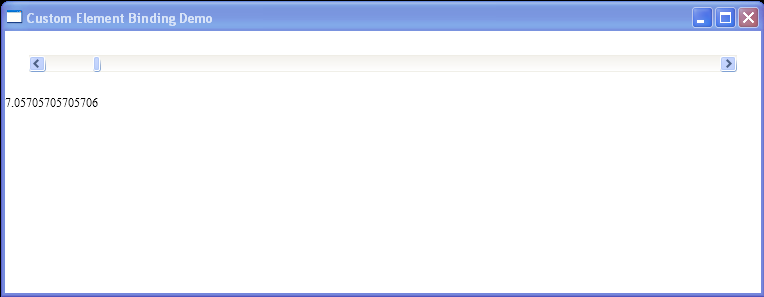